Using Location on Android
When utilizing Android-supported location services, your application must request access to location services functionality. There are two categories of permission:
The Tides app asks for precise location, but it can be changed to approximate. The access is foreground only, but we can get more granular: while the app is being used, just this time, or reject access
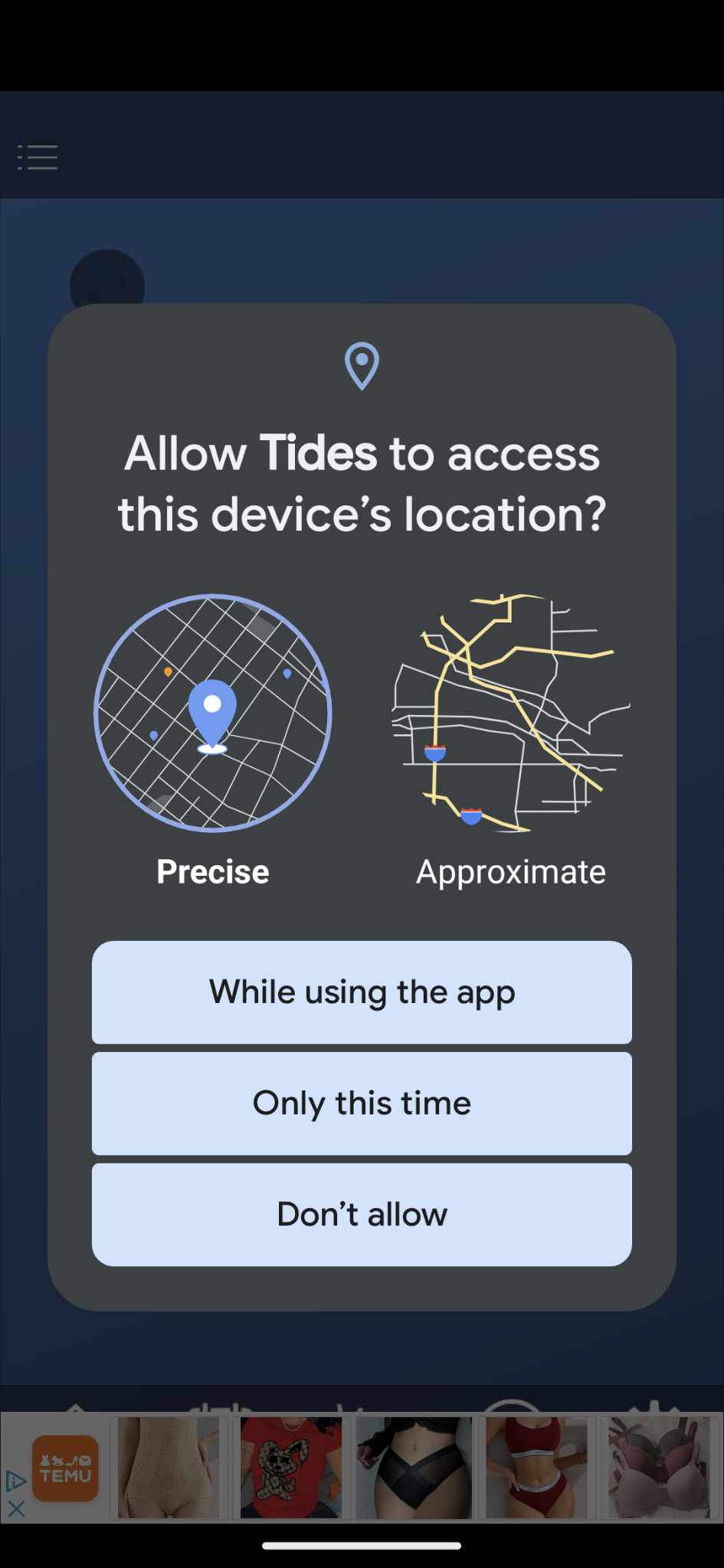
Get Last Location
Using the Location Provider
When requesting a location on Android, a best practice is to ask for the last location for an initial pinpoint.
Aside: This is a much better user experience than applications that use 0 latitude/0 longitude as a starting point. If the map blips to the Atlantic Ocean off of Africa before zooming to your location - that’s what the app is doing. Once you see it, you cannot unsee it.
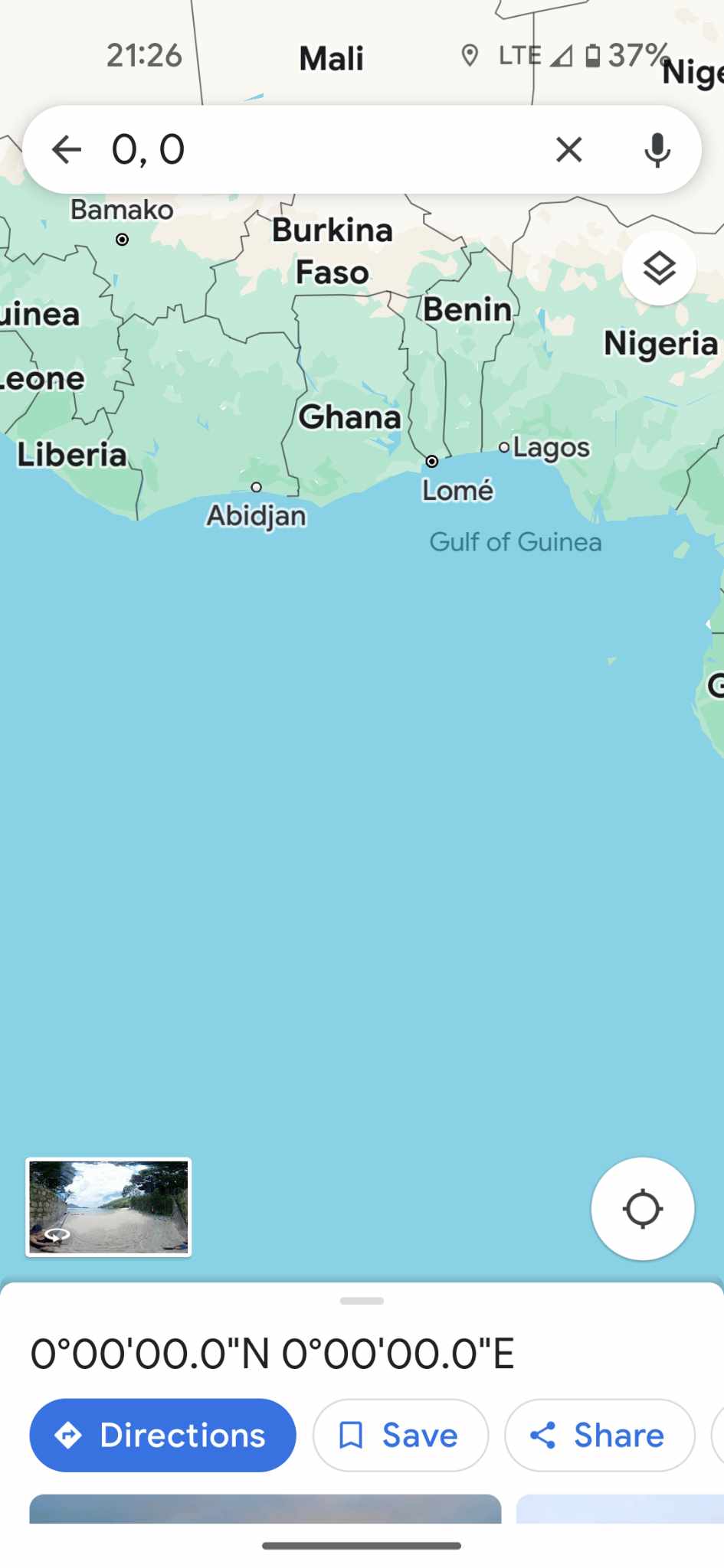
When requesting a location, you have several parameters that can be utilized:
Ensure you have added the correct permissions in your manifest file.
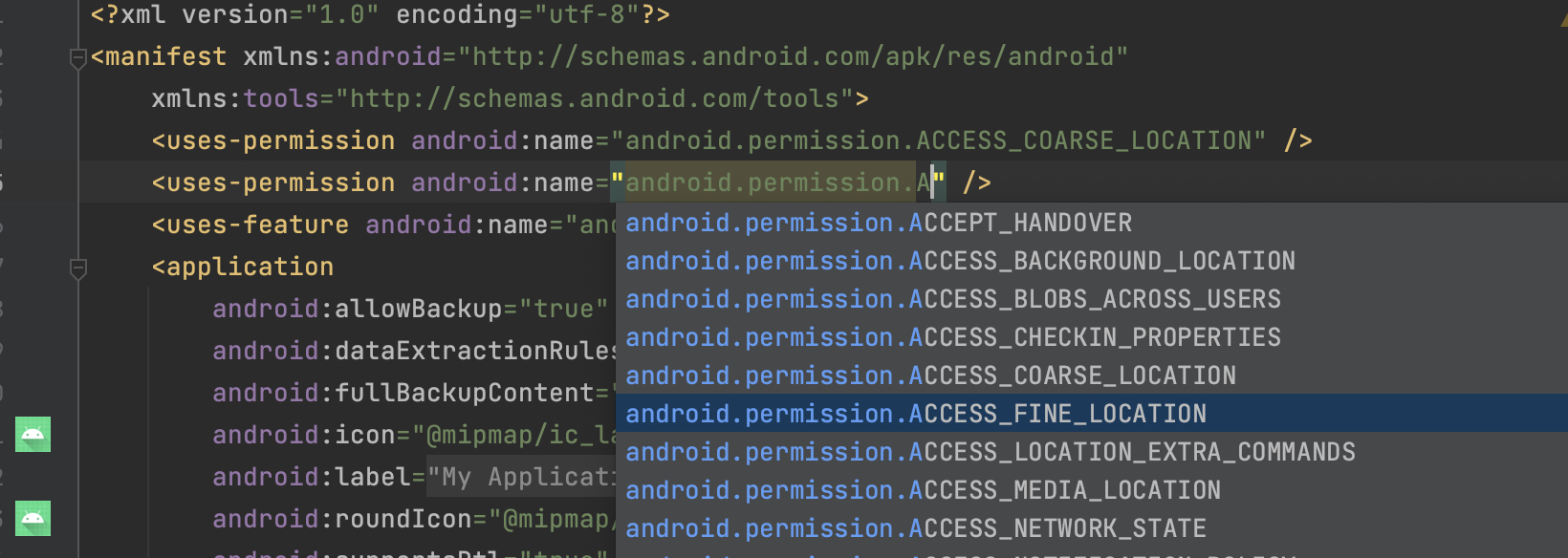
Using Location on iOS
- Location usage is framed similarly as it is for Android.
- Foreground or background: Foreground access to the location is provided by the NSLocationWhenInUseUsageDescription key. If background usage is required, use NSLocationAlwaysAndWhenInUseUsageDescription.
- When requesting location on iOS, you can request a one-time request requestLocation(), or a continuous request startUpdatingLocation() . This will run until the request is stopped stopUpdatingLocation(). Both of these methods will use GPS to get the location to a high level of accuracy.
- iOS also offers the startMonitoringSignificantLocationChanges() , that will only update if large changes in location have occurred. This method uses Wi-Fi and cellular radio, not the GPS.
- The following code uses the foreground access NSLocationWhenInUseUsageDescription to get a one-time location request:
Background location and geofencing
Geofencing is the creation of virtual fences in an area. When a target device enters (or leaves) the area, the user is alerted. Geofencing can be used for targeting advertising - “Get 15% off pumpkin spice lattes, today only with the Starbucks app” when a customer enters a geofence around a popular coffee shop.
Android
Geofencing requires background location access - it is unlikely that everyone has the Starbucks app open at every given minute! To prevent over-alerts, Android offers both GEOFENCE_TRANSITION_ENTER (the user has entered the geofence, and GEOFENCE_TRANSITION_DWELL (how long the user has been inside the geofence). So if they are driving through - the alert might not fire. Android suggests that geofence alerts may take 2 minutes to fire - as background location tracking is not as frequent to save battery.
iOS
iOS works much the same way. Once a user has crossed the boundary of your geofence, iOS waits 20 seconds to send the alert - making sure that the user is still on the same side of the geofence.
Location Based Apps Best Practices
Smartphones are mobile computers, and if your location can utilize the specific location of the device, you can better optimize the experience for your customers. But there are privacy and “creepiness factors” that must also be taken into account when in the development process of your location-based application.
When using GPS location, your application is collecting the exact location of your user. There are implications that you should understand before collecting this data.
Privacy: How will your application store user data? If your application is using precise location data, laws like GDPR and CPRA require specific actions to protect your customer’s data. While apps like Strava now hide the start/stop of your run (in order to not publicly broadcast your front door), Strava accidentally exposed the location of US military bases in Iraq and Afghanistan with a data release.
For fleet tracking apps (think UPS and FedEx, but also perhaps the delivery app for doordash Uber Eats, and the driver apps for Lyft and Uber, the precise location of your employees is a great way to track that everything is going as expected.
On a positive note, all users must grant permission to share location data with the application. Users can use the device settings to review all apps with location permission and revoke location access to any app.
Creepiness: It is important to know your target audience. For example, it is probably inappropriate to use precise locations to match in a dating app. Using the city-level location would be more acceptable (and possibly a lot safer for those on your application). It is also a key indicator of dating success.
Apps should broadcast the user’s current location with extreme discretion. For example, sharing your Uber ride with friends adds a level of safety. Sharing your drive to grandma’s house gives grandma a heads-up as to your ETA.
Battery: When your customer’s device uses GPS, Wi-Fi, or Bluetooth to obtain the location, radio receivers are powered up to receive signals. These receivers use battery resources, so it is important to balance the frequency and accuracy of the location call with the amount of power drain that will result. If your location application can get your users out to the wilderness, but not back from the wilderness, you might have angry customers (or worse).
AT&T’s Drive Mode app suspends the delivery of Text messaging when your speed is over 25 MPH. By delaying SMS messages from arriving, distracted driving accidents can be decreased. The initial version of the Android App used GPS location every 3 minutes to determine speed (even when you are sitting on your sofa watching Netflix). This led to complaints about battery drain. The app has since been improved to use the Android Activity Recognition API to determine you are in a car, before using the GPS to measure speed.
Alternatives to Location-based services
Smartphone location-based services have resulted in the launch of entire industries. But, there is also a mistrust of developers who misuse location data (“Why does my sudoku app need my location?”). So, what are the alternatives to using the location sensors in the device?
One such option is AbstractAPI’s IP Geolocation service. When your application makes a call to your server, you’ll receive the IP address assigned to the mobile device. Use the Geolocation API to look up the IP Address, and return a coarse location. This requires a free API key that you can create when you sign in to AbstractAPI.
The request looks like this:
This should be considered a very coarse location. Your mobile data does not enter the “internet” when it hits the cell tower - it is carried to a data center owned by your cell carrier. In my case, the API shows my location to be about 90 minutes by car away from my actual location.
One advantage to this approach is that your application will not require the granting of location permissions. The downside to this is that you’ll have to be very careful in how you utilize location - for both accuracy and to not appear as if you are trying to circumvent any app store guidelines.