Read the Image File
First, capture the input type file data by grabbing it from your onChange event's files array.
Create a Blob
Next, create a Blob and get the blob's URL. For those that don't know, a Blob is a file-like object of immutable, raw data. It represents data that is not in Javascript native format. The file blob URL refers to its location in memory.
Create a new Image object and set its source to the blob's URL.
Create a Canvas Element
Use the width and height of the original image to create a Canvas element. Since we don't want to change the actual dimensions of the image, it's important to retain the same height and width.
Get a New Blob from the Canvas Element
Finally, you can use canvas.toBlob to get another Blob from the Canvas element, specifying a reduced image quality for desired output size. The reduction in quality will result in a smaller blob size.
Now you can process the new image element, display it, or upload it.
Compress Images Using an API
There is a much easier way to get a compressed image in a Javascript application, and that's by using a third-party library or API. Libraries and packages like CompressorJS and Browser Image Compression are great, but they increase the size of your bundle and add additional load time to your application.

An image processing API is independently hosted, so it has no effect on bundle size. Let's take a look at using the AbstractAPI Image Processing and Optimization API to create a compressed image file.
Get Started
First, you'll need to sign up for a free account and get an API key. AbstractAPI is free to use, but all endpoints are secured by HTTPS and protected by API keys.
Acquire an API Key
Click “Get Started” on the API home page. If you have an account, you may need to log in, otherwise, you will need to provide an email address and password in order to make one.
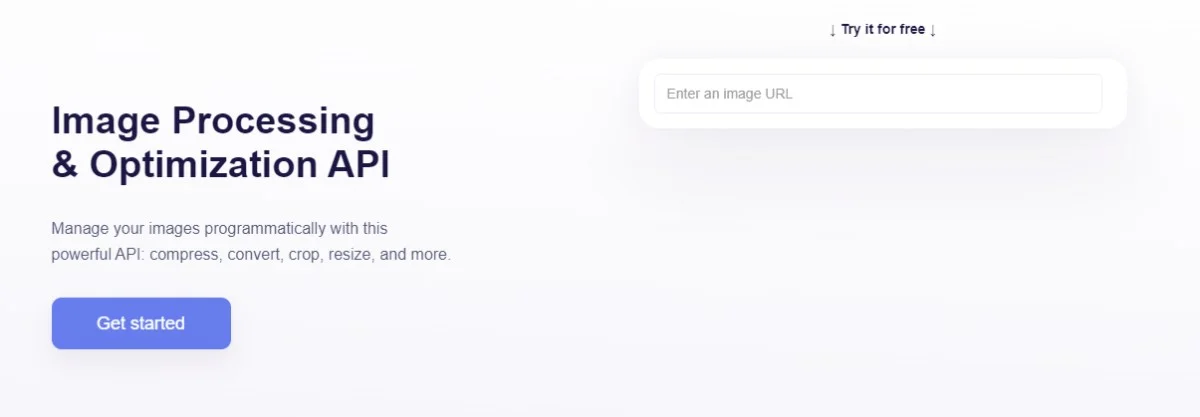
You’ll land on the API test page, where you'll see links to documentation and pricing, as well as settings, account information, and your API key.

Make an API Request
AbstractAPI exposes an endpoint that accepts an image file, performs some optimization on that file, and returns a JSON object with a link to the new file and information about the new image.
There are two methods to upload images: multipart form upload and regular POST request. Multipart upload allows you to send the complete image file, while POST request accepts a URL parameter pointing to a hosted image.
Let's use this sample image from AbstractAPI to send a POST request containing an image URL:
“https://s3.amazonaws.com/static.abstractapi.com/test-images/dog.jpg”
This code block tells the API to use lossy compression to resize the image file. The API will intelligently compress images to achieve maximum file size reduction with minimum quality loss. If you'd prefer to exert more fine-grained control over the output quality, the quality option allows you to do that.
Examine the Compressed Image
The API will return a JSON object with a URL to the new image and some data about both the original uploaded image and the compressed file. The data looks something like this:
Here, we can see that the image width and height have not changed, but we have decreased the file size by about 130500 bytes.
Conclusion
While it is possible to roll your own code to compress images in Javascript, it's not recommended. There are many free libraries and packages out there today that can handle this for you, as well as APIs for compressing images that do not require you to install any additional dependencies.
FAQs
How Do I Compress an Image in HTML?
Is it not possible to compress an image using HTML alone, however you can compress an image using the HTML5 Canvas element and Javascript. There are also many libraries out there that will handle image compression for you, including Browser Image Compression, CompressorJS, and Squoosh.
Image compression and image resizing are two separate things. Image compression refers to the process of changing the size of an actual image file (i.e. shrinking the file from 65MB to 65KB.) Resizing images, on the other hand, is the process of changing the width and height of the image for display.
How Do I Compress Images for the Web?
There are many ways to compress images for a website or application. If you are handling compression in the browser and using React, you might look at a package like Browser Image Compression. You can also rely on a third-party image API like the AbstractAPI Image Optimization API to handle image compression for you.
How do I Shrink an Image Without Distorting It?
To shrink an image without distorting it, the most important thing is to maintain the aspect ratio of the image. That means ensuring that the relationship between the image's width and height doesn't change. If the image is twice as tall as it is high, it needs to remain twice as tall even when the image size changes.