Compress vs Resize
Before we start, let's take a moment to define the difference between these two terms. The term "compress" typically refers to file size, whereas "resize" refers to the size of the actual image contained in the file.
Resizing an image (or at least, downsizing an image) will also result in file compression. But it is possible to reduce an image file size without changing the actual size of the image. This is done by reducing the quality of the image (called "lossy" compression because the quality is "lost.")

In this article, we will look at using lossy compression to change file size. Both of other methods used, however, can also be used to resize an image.
Compress Images Using Pillow
The Pillow library is a fork of PIL (Python Image Library.) PIL was deprecated around 2018. Pillow provides a set of tools for reading, writing and manipulating image data. You can use Pillow to resize images, compress images, change the color, crop, filter, and all sorts of other things.
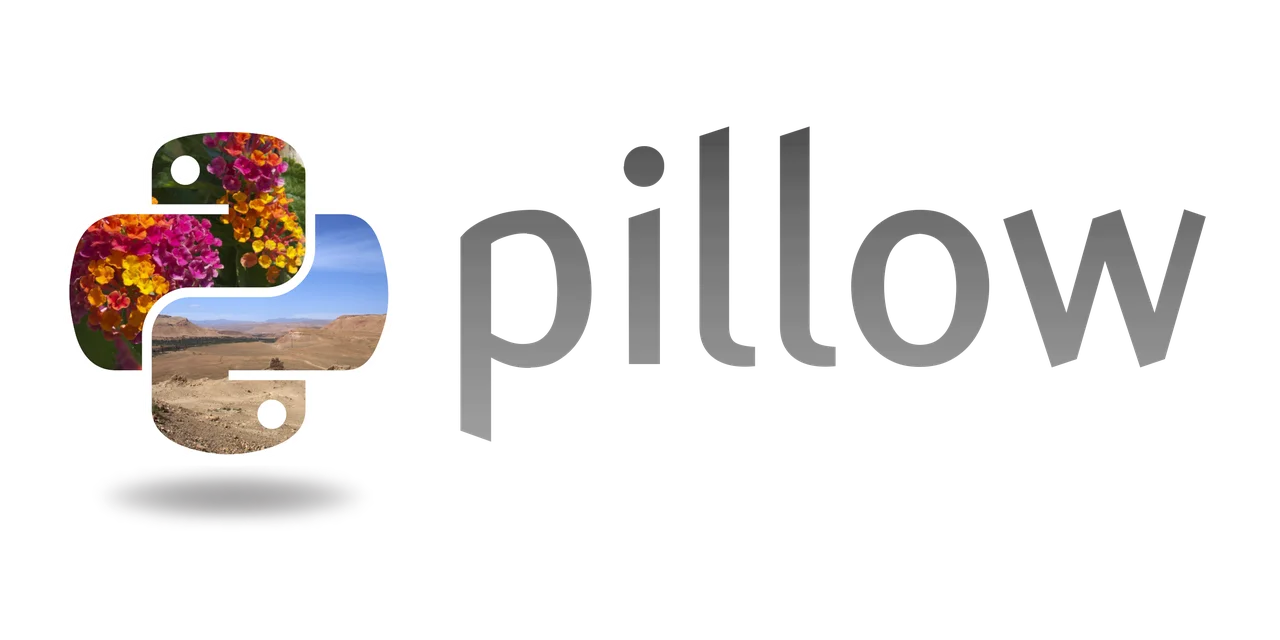
The core of Pillow library functionality is the PIL Image object. This is an in-memory object that Pillow creates. It exposes the methods needed to read, write, and perform image compression.
How to Compress Image Files Without Changing Image Size
Let's look at the basic method for compressing images using Pillow.
Here, we've used the PIL Image object to open our image file from the specified path. We've then simply resaved the image as a new file, but adjusted the image quality value as we saved it. Where the original image had a quality of 100, the new image will have a quality of 10.
This is quite a large degree of quality loss and will result in a drastically smaller file. To maintain higher image quality, simply use a different number for the quality value.
The optimize parameter does a separate pass over the final file to reduce the size of the file as much as possible before saving.
Compress Images Using AbstractAPI
Another way to compress images, particularly if your images are already hosted somewhere online, is to use a third-party compression or image resizing API. These APIs accept all popular image formats, such as png images, jpg, gif, bmp, etc. One such API is AbstractAPI.

AbstractAPI allows you to make a simple POST request to an authenticated endpoint protected by an API key. You provide the endpoint with the URL that points to the image you want to compress, along with other optional flags like whether you want to resize the image, and whether the API should use lossy compression to reduce the file size.
To compress an image without resizing it using this method, we simply tell the API to use lossy compression without changing the resulting file size.
How to Get Compressed Images Without Changing Image Size
Let's quickly look at how to use the AbstractAPI Image Processing endpoint to reduce image file size.
Acquire an API Key
Go to the Images API home page in your browser and click “Get Started." If you haven't used AbstractAPI before, you’ll need to sign up with your email and a password. If you already have an Abstract API account, you should log in.
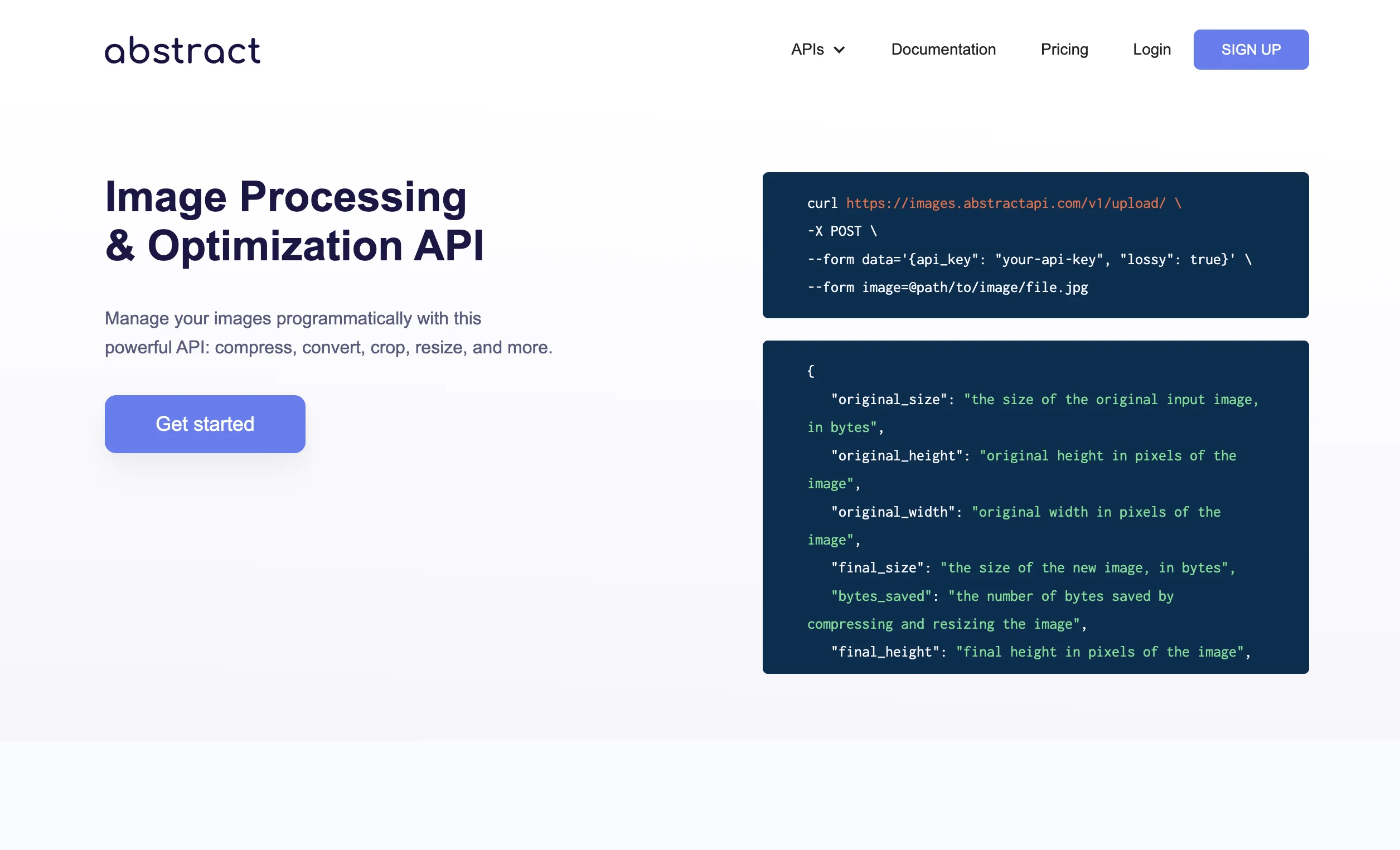
Once you’ve done that, you’ll land on the API dashboard.

Make a Request to the API
You can use the Python requests module to send a POST request to the endpoint with the information.
This request will return a JSON object like the following:
As you can see, the original image was not modified: the original_height and original_width are the same as the final_height and final_width. However, the file size was reduced by 130458 bytes. This is because the lossy parameter reduces the file quality slightly to achieve about a 10-20% reduction of file size.
The final image will have a slightly lower quality than the original image. You can also pass a quality parameter that further reduces the quality of the output image. Read the documentation for a deeper explanation of the optional parameters available.
The API returns a URL to a new file, hosted at one of AbstractAPI's AWS S3 buckets.
Conclusion
In this article, we looked at two different ways of performing image compression in Python. First, we used Pillow (a fork of PIL) to reduce the image file by saving a new file and passing a quality parameter to reduce image quality.
Next, we used AbstractAPI's Free Image Processing endpoint to perform lossy compression on a hosted image file.
FAQs
How Do I Lower the Resolution of an Image in Python?
The easiest way to lower image resolution in Python is to use Pillow. First, use the PIL Image object to open the image file. Next, write the image data to a new file, passing a quality option that lowers the quality of the final image. This will result in a loss of picture quality and a smaller file size.
How Do I Resize an Image Without Losing Quality Python?
There are only two ways to shrink an image: by throwing away some pixels or by blending those pixels with their neighbors. Same with upscaling: you will need to create new pixels based on some kind of interpolation algorithm.
Either one of these operations will always result in some quality loss. One way to reduce the amount of quality lost is to use lossless compression to save the photo, and set the image quality to 100.
What Is Lossy vs Lossless Compression?
Lossless compression allows a file to be reduced in size in a way that still allows that file to be rebuilt exactly as it was before it was compressed. All of the data that was in the original file is still available once the file is uncompressed.
Lossy compression works by permanently eliminating some data from the file, so it will not be possible to reconstruct the file in the same way once it is uncompressed. Lossy compression results in smaller file sizes, but there is some loss of file quality, hence the name "lossy."