Get Started
First, download the package from NPM.
Next, import the module into your React component.
Compress an Image File
Add the following code block to your component to use browser image compression in an async/await context.
As you can see, the image compressor logic is very straightforward. Simply pass the image file to be compressed, along with an object specifying the options for image quality and image size. The compressed file can then be uploaded to a server, saved, or forwarded to another image manipulation function.
The options object allows you to specify multiple different extensions for the image compressor, including whether or not to use web workers, a callback function to run once compression is complete, a signal to indicate whether the process has been completed or has been aborted, and advanced configuration options.
The complete options API is as follows:
The only required parameters are maxSizeMB or maxWidthOrHeight.
Compress an Image File Using AbstractAPI
Libraries and packages are great, but they do add to your bundle size and ultimately make your app larger. If you're looking for a way to compress an image file without installing an additional package, consider using an API service.
Image compression APIs expose endpoints that allow you to upload an image, specify the way an uploaded image should be compressed, and then return either the compressed image or a link to a hosted image file.
Let's look at one such API: the AbstractAPI Image Processing and Optimization API.
Get Started
To use the API, you'll need to sign up for a free account and get an API key. All of the Abstract API endpoints are secured by HTTPS and protected by API keys. They are all free to use and signing up for an account will not add you to any mailing or spam lists.
Acquire an API Key
Go to the API home page and click “Get Started.” If you’ve never used AbstractAPI before, you’ll need to input an email and password to create an account. If you have an account, you may need to log in.
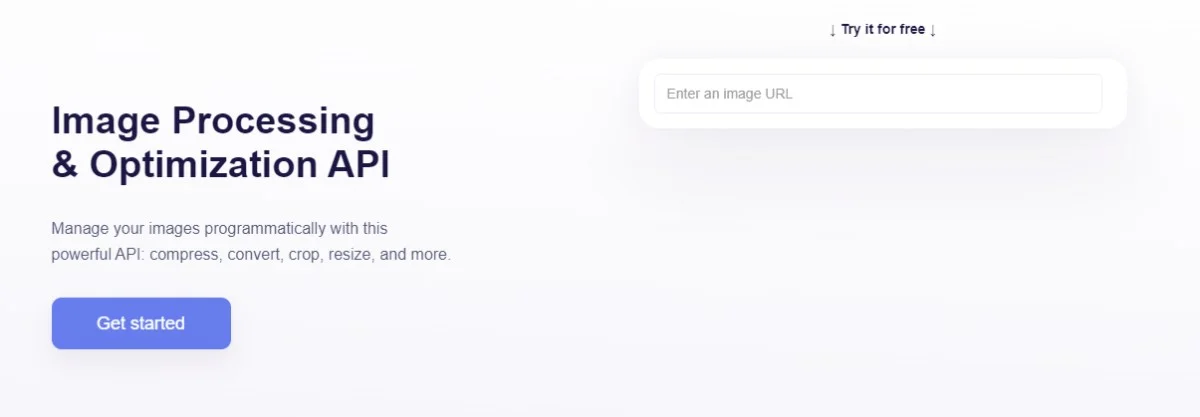
You’ll be taken to the Images API test page, where you'll see links to documentation and pricing, as well as your unique API key.

Make an API Request
To compress an image file, we'll send an HTTP request to the API endpoint. AbstractAPI provides two methods for image upload: multipart form upload so you can send the complete image file, and regular POST request, where you specify the image to be compressed by providing a URL.
In both cases, AbstractAPI will process the image and return a url for the resized image path, hosted at an Amazon S3 bucket.
We’ll use this example image from AbstractAPI to send a regular POST request with an image URL:
“https://s3.amazonaws.com/static.abstractapi.com/test-images/dog.jpg”
Here, we've told the API to compress the image by setting the lossy option to true. The API uses lossy compression to massively shrink the image file size while slightly reducing resolution quality.
Receive the Compressed Image
The API returns a JSON object with information about the original image and a URL to the new image. The data looks something like this:
As you can see, the image width and height have not changed, but we have decreased the file size by about 130500 bytes.