JavaScript Validation
Since the majority of frontend web apps and websites today are built with JavaScript, it’s worthwhile understanding how to do basic email validation using JavaScript. The most common way of validating emails in JavaScript is to use Regex.
Regex Validation
Regex, or regular expressions, are sequences of characters used for pattern matching in software development. A regular expression can be created and then compared against an input string to find irregularities or mismatches in the string.
A possible regular expression that could be used for basic email validation might look like this:
Let’s break down this regular expression to see how it validates emails.
Using Regex
To use this Regex in your validation, you would create a Regex object using this pattern, and then call the test function provided by the object to compare an input string:
The result true tells us that the input string test@gmail.com is a valid pattern match for the provided regular expression.
To see how to actually use this logic in your app, take a look at the next section, where we’ll plug it into a React component.
JQuery Validation
You can use the regex pattern we just established to validate emails in JQuery quite easily. Simply create a function called validateEmail that accepts an email as an argument and matches the email to the regex pattern.
Once you have the function, you can hook it up to a button or onClick handler to validate an email from an input form before you send it to a server or do anything else with it.
React Validation
React is the most popular frontend JavaScript framework being used today. To use Regex in your React application, all you need to do is pass it as the validator for an input component. You can do this using React Hook Forms, if you’re using that particular library to assist you with form-building, or you can just call your validation function in the onClick or onSubmit handler.
Related:
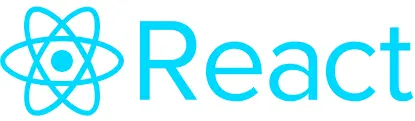
Let’s take a look at what that could look like.
This is a very basic component that holds the value of the email string in state, updates that string with the user’s input when they enter it into the HTML text box, and then calls a handleSubmit function when the user clicks a button. The handleSubmit function compares the email held in state against a regular expression and if the email string passes the Regex match, the email is sent to the server.
Problems With Regex
The main problem with using Regex for email validation is that the regex for matching email addresses can get very complicated. There is no "fool-proof" regular expression that will match 100% of valid email addresses.
There is an official standard known as RFC 5322 that dictates what valid email addresses should look like. That regular expression looks like this:
As you can see, it’s very complicated.
The other issue with using Regex to validate emails is that Regex only checks syntax. It doesn’t allow you to check the validity of the domain name, MX records, whether the address is a known spam trap or on a blacklist, whether it is a role-based email or free email account.
In order to check those things, you need to use a dedicated email validation API.
Using an API
Let’s take a look at how to use the AbstractAPI Free Email Validation API to validate a single email address.
Get Started With the API
Navigate to the API home page and click "Get Started"

Once you sign up for a free account you’ll land on the API dashboard, where you’ll see an API key and links to documentation, pricing, etc.

Copy the API key.
Send an Email Validation Request to the API
In your JavaScript code, create a function called sendEmailValidationRequest.
This function will send an AJAX request to the API endpoint containing the email for validation. It will then receive and analyze the response from the API and return a boolean value for us to use to determine if the email is valid.
The response from the API will look something like this:
For now, we can just look at the is_valid_format field. Pull out the value from this field and return it as the result of our function.
This returns false if the email address provided is an invalid email address. If the email validation is successful, it returns true.
The function is an async function, because we are sending a network request. We can improve the user experience by handing any errors that arise from invalid emails or failed network requests.
The AbstractAPI endpoint checks a lot of things when you send it an email string. It runs a sophisticated regular expression check on the email, checks for syntax errors and typos in the address (for example john@agmil.com), and also does real-time SMTP and MX record checks against the email's domain.
Multiple Email Addresses
What about validating multiple emails? AbstractAPI provides a bulk upload option where you can upload a CSV file containing a list of comma separated email addresses to validate. The API runbus the same validation process against each email on the list and sends you a result file to the email address they have on record.
This is a good way of doing regular email list cleaning once every six months or so. You should be using both real-time validation and regular bulk validation to ensure that your list is free from invalid email addresses.
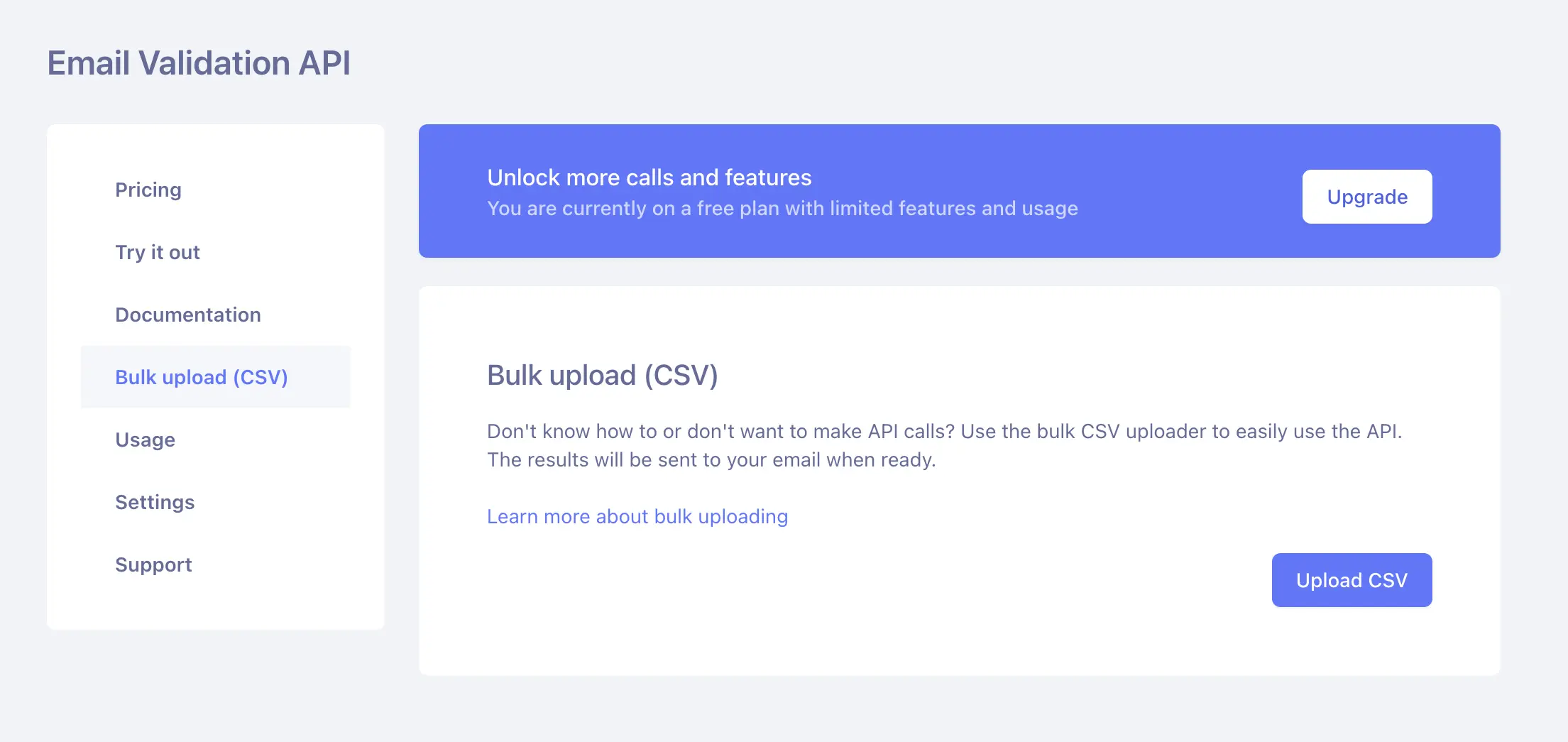
Common Pitfalls
The primary mistake people use when validating mail-addresses in Javascript is using only one validation method. If you solely rely on Regex to validate incoming mail-addresses, you will not capture all possibly valid emails, and you could prevent some valid addresses from getting through.
Regex is a great first step in validation - as is using the HTML attributes provided by HTML5 components. These can do a lot in raising basic errors to your user for you. However, your validation should be backed by a dedicated API that checks MX records, SMTP, and domain name validity.
IMAGE: email-validation-error
Conclusion
Validating email addresses in Javascript is a simple and effective way of preventing invalid email addresses from making it onto your email list. Make sure your email marketing campaigns are targeted and effective, and that you’re not sending emails to people who can’t receive them or don’t want them.
FAQs
How to validate multiple emails in Javascript?
The best way to validate multiple emails in Javascript is to use a dedicated email validation API such as AbstractAPI’s Free Email Validation API, or a service like Neverbounce or Zerobounce. These APIs can be easily integrated into your application via a simple network request.
How do you check whether email is valid or not in bulk?
The fastest way to bulk validate emails is using a bulk email validator that allows you to upload a CSV file full of email addresses. Most email marketing services providers give you a simple way to download a complete list of your emails - once you have this, simply upload it to your validating software of choice (AbstractAPI, Neverbounce and Zerobounce are good options.) The service will run checks on all the addresses in the list and provide you with output about which emails are valid and which are not.