Why Validate Emails?
There are three primary reasons you need email validation: data accuracy, enhancing the user experience, and security.
Data Integrity
Every invalid email address on your mailing list is a chance for an email to bounce. Every bounced email hurts your sender reputation, which in turn leads to lower deliverability and lessens the chances that your emails to valid email addresses will end up in users’ inboxes.
Not only that, invalid email addresses take up space on your list - space that you pay your email marketing provider for.
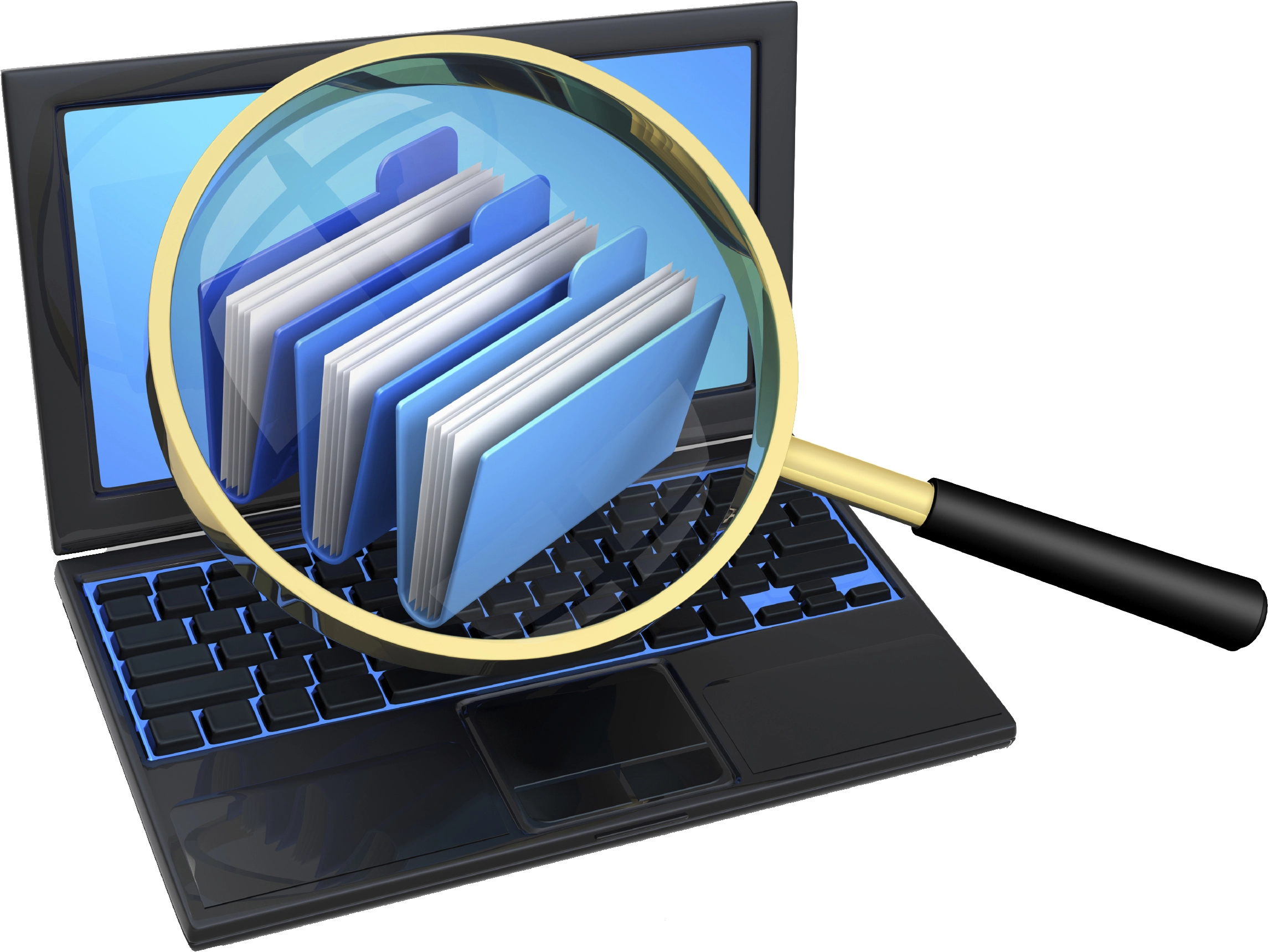
User Experience
Users who sign up for your emails usually want to receive them. Users who sign up for your application usually hope to be able to log in easily. If they accidentally give you an incorrect email address they will never receive your emails and will have a frustrating time trying to sign in to your app.
Security
Validating emails not only ensures that your user data is correct - it also prevents hackers, spambots, and other bad actors from making their way onto your list or into your servers. This protects not only you, but your users as well.
Methods of Email Validation in JavaScript
There are several ways to validate emails in JavaScript - some are better than others. Let’s take a look at all of them, and discuss why some are preferred.
Using Regular Expressions
Regular expressions are one way of validating email addresses and other strings like phone numbers - however they are also one of the least accurate methods.
To validate an email address using regex and JavaScript, you simply make a regular expression that matches a valid email address, then compare the email address a user gave you against that regular expression.

The problem is, it’s nearly impossible to write a regular expression that captures all possible valid email addresses. Not only that, using regular expressions to validate emails won’t let you check MX records, Domain validity, or determine whether the address is a temporary email, role-based email, or known spam trap.
Using HTML5 Validators
HTML5 validators are the first line of defense for email form validation. They are quick and easy to use and should be added to any HTML form you include in your app. Validating input with HTML5 is as simple as including a required attribute on the tag, and an input type of email. These are similar to the form name and other HTML5 attributes you include on an HTML form.
HTML5 should not be the only thing you rely on to validate emails, however, for the same reason regex shouldn’t be the only thing you use: it doesn’t check MX records or alert you to potential spam traps and other issues.
Using External Libraries and Plugins
Libraries and plugins like email-validator and email-validator-js take some of the work of validating emails off your plate by running regex and other checks against email addresses for you. This means you don’t have to write your own regular expression to check email addresses.
Again, however, these libraries don’t usually check things like spam traps and domain name validity.
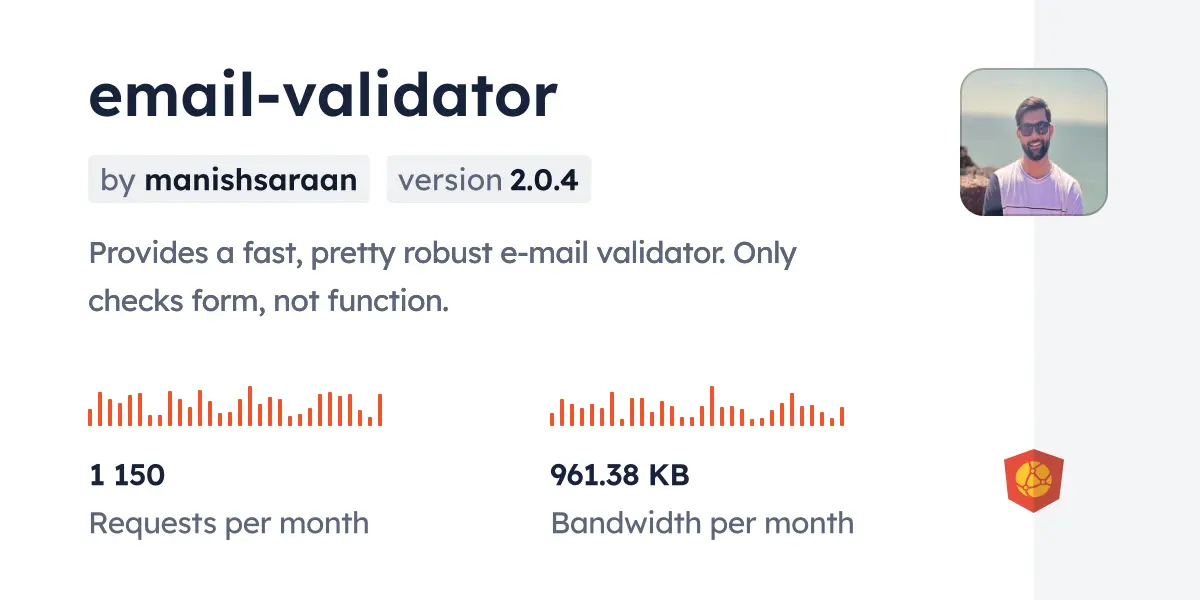
Using an API
The best and most robust way to validate email addresses is using an API. Email validation APIs expose a REST endpoint (secured by an API key) that allows you to send a request containing an email to validate. The API uses regexp, checks MX records, compares against a list of known spam traps and blacklists, performs domain lookup, and does a slew of other tests to determine whether the email is valid, then returns a JSON response with the validation data.
Step-by-Step Guide to Email Validation
Let’s take a look at how to validate an email address using AbtractAPI’s Free Email Validation API.
Get Started With the API
Navigate to the API home page and click "Get Started.” You'll sign up for a free account with an email address and password. Then you'll land on the API dashboard, where you'll see your API key. Copy the API key.
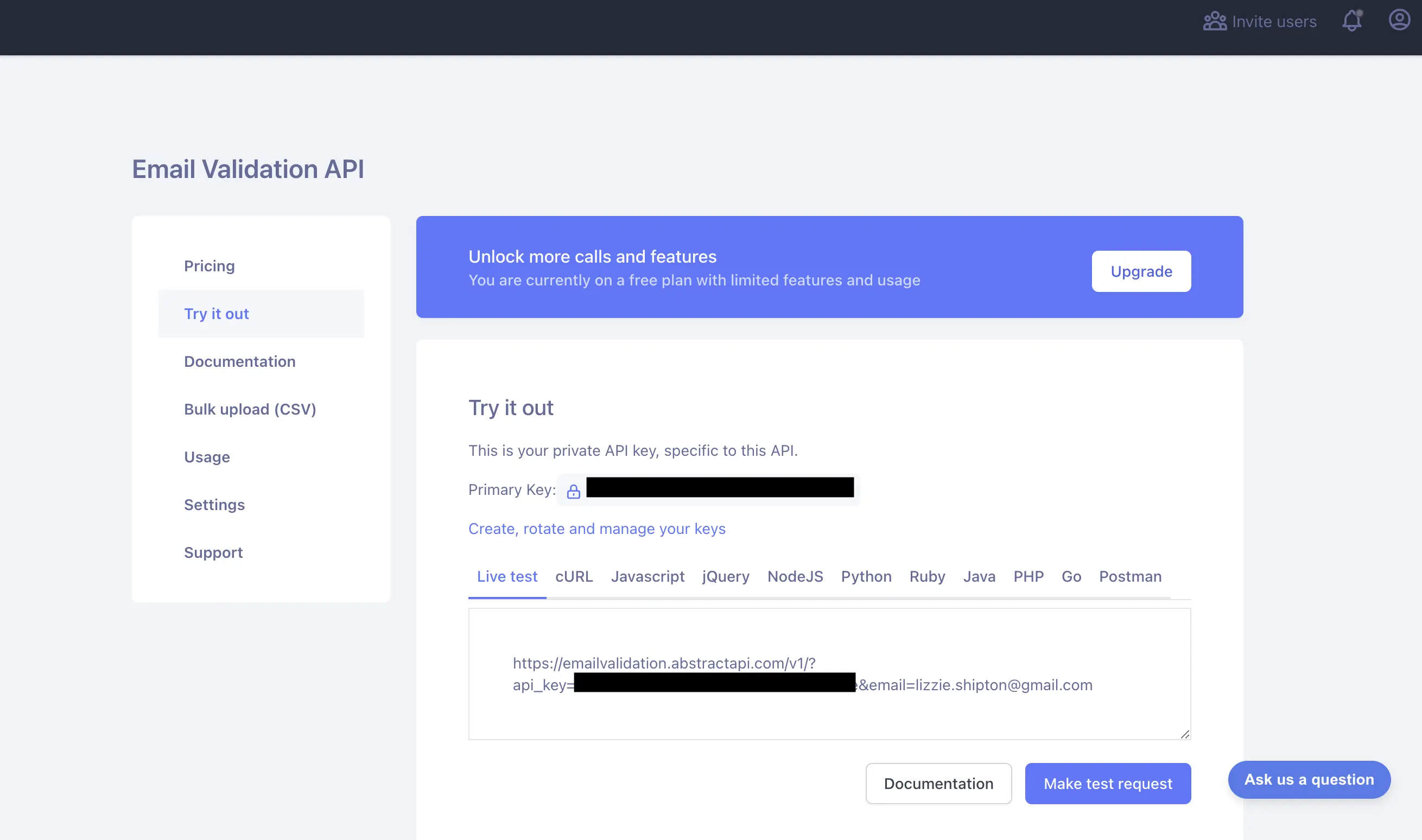
Send an Email Validation Request to the API
In your Javascript code, create a function called function validateEmail.
This Javascript function accepts an email address as its argument and sends an AJAX request containing the email to the API endpoint. The API returns a JSON response. If you’re doing this validation on the frontend, you should call this function in the onclick of you form submit button (before you send the data to your server.)
The response from the API will look like this:
You can use any or all of these fields to determine whether the address is valid. For this tutorial we’ll just use the is_valid_format.value field.
Here’s the code snippet you would use to send this request:
This returns false if the email address provided is an invalid email address. If the email validation is successful, it returns true.
The function is a async because it involves a network request. We can improve it by adding error handling.
Common Pitfalls and How to Avoid Them
Here are some of the most commonly made mistakes when it comes to validating email addresses.
Overly Strict Validation Rules
If you are using regex to validate email addresses, you will likely run into the issue of using a regular expression that doesn’t capture all valid email addresses, meaning many valid addresses will be incorrectly marked as invalid.
A typical regex email validation string looks like this:
Unfortunately, not only is this difficult to read, it doesn’t allow for special characters in the email address.
Ignoring User Experience
One thing developers frequently forget to do when building email validation components is handle errors properly. You should surface helpful, user-friendly errors whenever something goes wrong in your application. If the user can take steps to fix the problem, make sure you outline exactly what steps they should take.
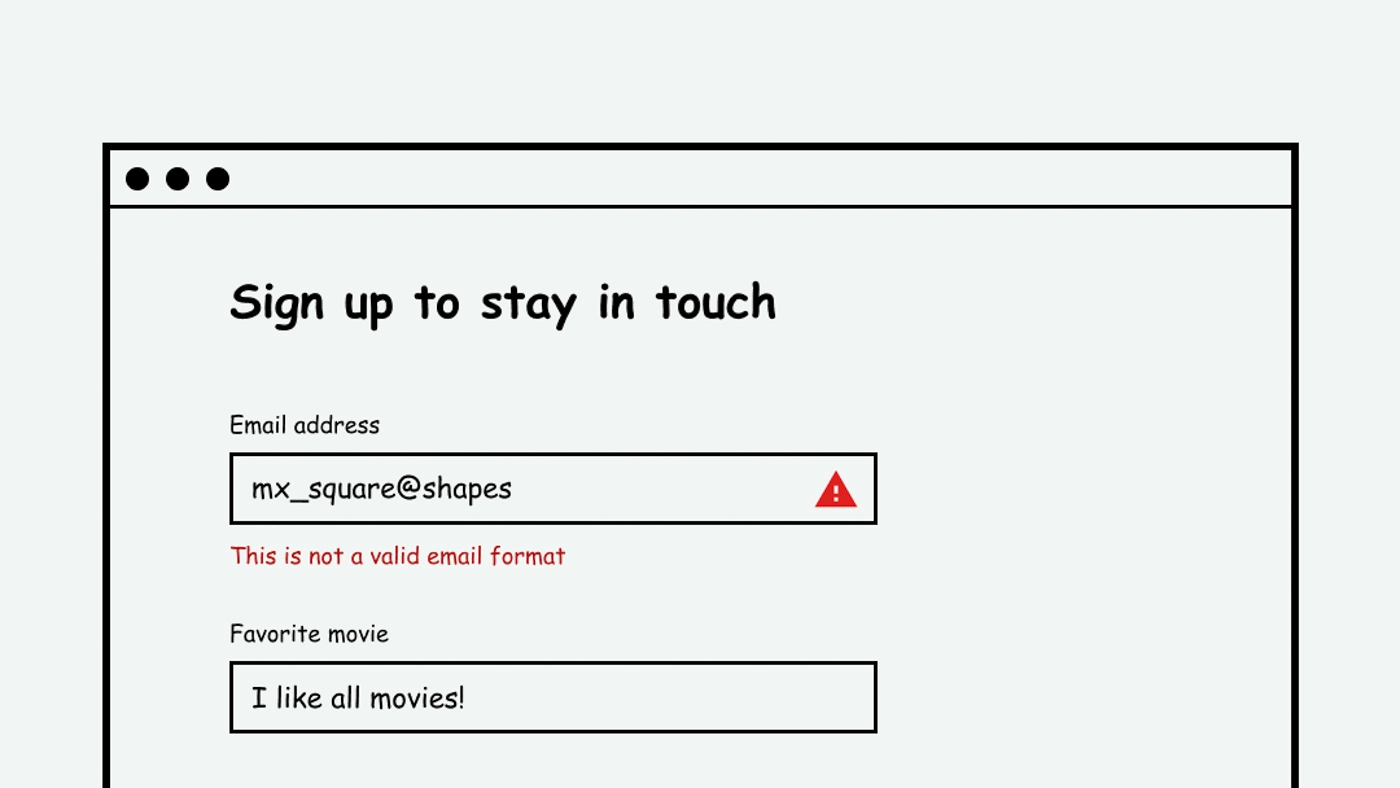
User experience is extremely important, especially during sign up, as this is the first time your users come into contact with your app.
Not Testing Across Different Email Providers
Once you have your email validation code written, you must test it across a number of different providers, using as many different top-level domains, patterns, and special characters as you can. Ideally, you should automate testing.
Advanced Techniques and Best Practices
When it comes to validating emails, there are a few best practices you should always engage in to make sure you’re giving your users a good experience and getting the most out of your validation:
- surface helpful errors to users
- use multiple validation methods (regex, HTML5 on user inputs, and real-time API validation)
- maintain email list hygiene by periodically bulk cleaning your email list
Client-side vs. Server-side Validation
All the methods we’ve discussed so far have been client-side validation methods. However, if you’re also using JavaScript on your backend (i.e. Node.js) these methods transfer quite easily to the server. Obviously, you can’t do HTML5 checks on the server, but you can use Regex to validate email addresses, and you can send validation requests to an API.
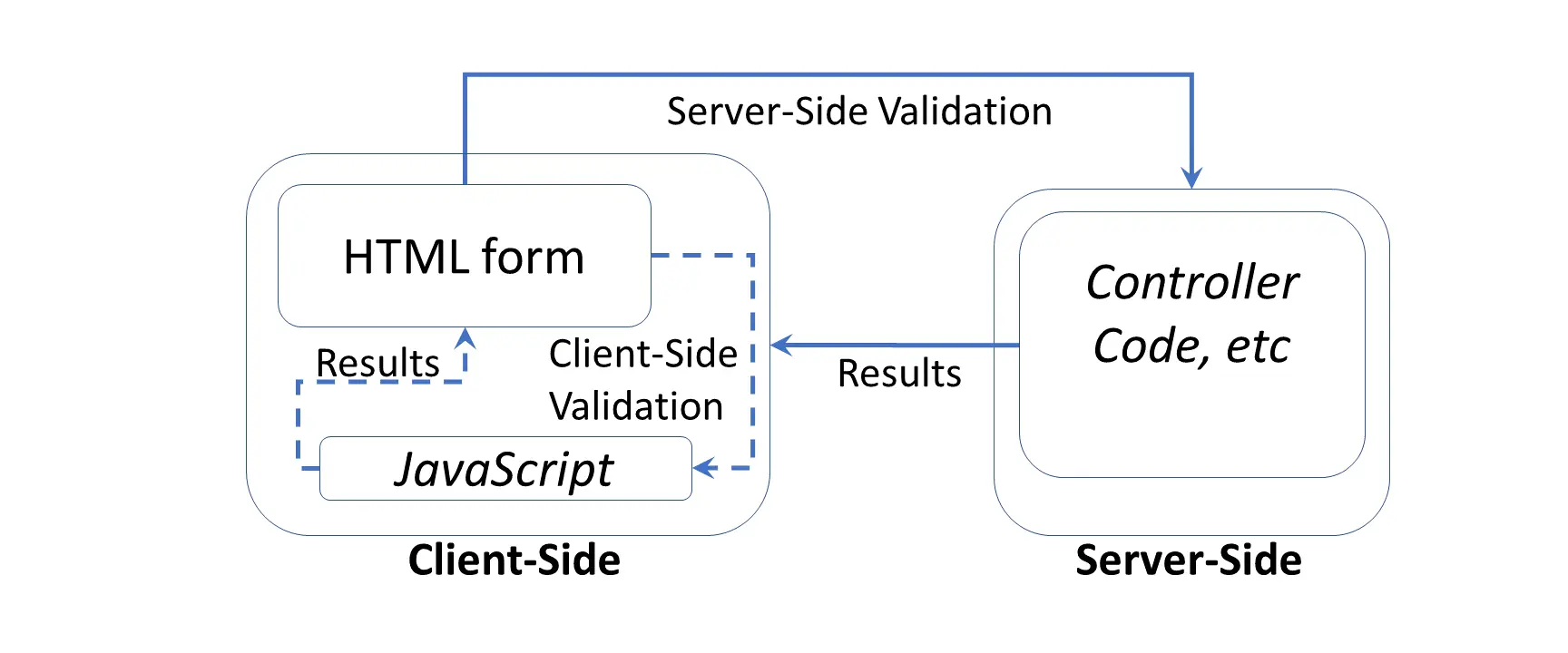
It’s really up to you whether you want to perform these validations on the frontend or do serverside validation. If you use Python, PHP, or some other language to run your server, you can still use all these methods - you will just need to look into the specific syntax for using Regex and making network requests in that language.
Conclusion
Email validation is critical to modern web development and email marketing. Invalid email addresses take up precious (and costly) space on your list while increasing your bounce rates and damaging sender reputation.
Maintain good email list hygiene by performing real-time validation against an email validation API and by doing periodic bulk email validation of your entire list.