Methods to Validate Mobile Number With 10 Digits In ASP.NET
There is a list of easy methods for validating mobile numbers in ASP.NET. In this article, we will discuss the various methods and provide practical code examples.
Improve your contact rate and clean your lists with Abstract's industry-leading phone number verification API.
Phone Number Validation in ASP.NET Core
In net core, validation rules are defined in the model. Phone number validation uses the DataAnnotations namespace, which has two validation attributes: DataType and Phone.
You should use the DataType in your model class if your MobilePhone field is of a simple type.
The DataType enumeration provides a list of standard data types supported by the class. The DataTypeAttribute class uses the DataType.PhoneNumber enumeration to get the specific data type to test.
As an alternative to the [DataType], you can use the [Phone] attribute. In this case, the class, not the enum is used for checking.
The Phone class derives from the DataTypeAttribute class and can be overridden and extended to provide additional validation behavior.
Both of these approaches are interchangeable and will work the same in most cases. However, validation using the Phone class is more complicated internally.
The listed validation methods have some disadvantages:
- No phone number length check.
- There is no verification that the phone number is valid in a particular country.
- A regular expression is used for data validation. By default, they accept the characters "-.()", as well as an extension marked as "ext.", "ext" or "x", which does not always correspond to the desired valid phone number format.
If you want to change the default valid number format, you can use the Phone class. In addition to the [Phone], add the [RegularExpression] attribute.
The following example uses the [RegularExpression] to check if the mobile number matches the standard 10-digit format, for example, 123-456-7890, (123) 456-7890, 123 456 7890, 123.456.7890.
Phone Number Validation in ASP.NET MVC
Model validation in asp.net mvc works just like it does in ASP.NET Core. You can use the [DataType] and [Phone] and code examples above to validate phone number formats in ASP.NET MVC and get the same result as for ASP.NET Core.
Phone Number Validation in ASP.NET WebForms
To validate mobile numbers in an old ASP.NET WebForms project, use a regex expression in the validation control, as shown in the following example.
This code creates a text box for entering a phone number, as well as a validator for it. The validator has a ValidationExpression property that contains a regular expression to check if the user entered value matches. In this example, the validator checks the entered number against the standard 10-digit format.
If the entered value does not match the pattern, then an error message is displayed, which is stored in the ErrorMessage property.
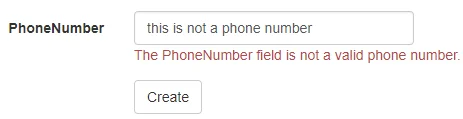
Adding Custom Phone Number Validation with Regular Expressions
A valid phone number can have different formats. They may contain country and city codes, have extensions, or include additional characters. Therefore, their validation is a rather difficult task. Regular expressions allow you to effectively deal with it. To use them in C#, you need to add the following code:
Then you need to define a regular expression that will be used to validate data entry. Depending on the format that is allowed in your application, the regular expression may change.
The following regular expression matches most standard phone number formats such as
0123456789, 012-345-6789, and (012) -345-6789:
If you want to validate the country code, use the following regular expression:
If your application allows you to enter a valid phone number with an extension, you need to choose a different regular expression:
Once you've decided which regular expression to use, all that's left is to write the function that validates entered value. Use the IsMatch function of the Regex class to do this, as shown in the following source code:
Alternative Methods for Validating Phone Numbers with ASP.NET
You don't have to write complex regular expressions to check whether the input number is valid. You can do this without writing a single line of code from scratch. You can use Abstract's Phone Validation API for this. This is a simple, lightweight, and fast API that allows you to validate phone numbers effortlessly, thereby increasing your productivity.
Run the following request to validate the mobile number using Abstract's Phone Validation API.
The request has two parameters:
- phone is the phone number that must be validated.
- api_key is the unique API key that you get after registration.

If the value is valid, you will receive the following response:
The "valid" parameter indicates whether the specified value is valid or not. In addition, you get other useful data such as international_format, country_name, registered_location, and carrier.
Try Abstract's phone validation solution, use its latest features for any of your projects, and make sure your users' data is clean and accurate.
Further Reading on Phone Number Validation
- https://www.abstractapi.com/guides/validate-phone-number-javascript
- https://www.abstractapi.com/guides/jquery-validate-phone-number
- https://www.abstractapi.com/guides/phone-number-validator-free
- https://www.abstractapi.com/guides/angular-phone-number-validation
- https://www.abstractapi.com/guides/c-validate-phone-number
- https://www.abstractapi.com/guides/validate-phone-number-laravel
- https://www.abstractapi.com/guides/php-validate-phone-number
- https://www.abstractapi.com/guides/validate-phone-number-python
Phone Number Validation FAQs
How can I verify a phone number?
To verify a phone number, you can use automated tools such as Abstract's Phone Validation API. It allows you to easily and quickly check whether the specified phone number is valid and also provides additional information about it.
How do I use Regularexpressionvalidator?
First, you need to create a TextBox control and a RegularExpressionValidator control. In the ControlToValidate property of the RegularExpressionValidator control, write the id of the TextBox that needs to be validated.
After that, use the ValidationExpression property of the RegularExpressionValidator control to set the regular expression according to which the data from the TextBox control will be validated. To set up the error message that should be displayed, use the ErrorMessage property of the RegularExpressionValidator control. The text color of the error message is set using the ForeColor property.
What is an alternate mobile number?
An alternate mobile number is an additional number that you can use to set up a separate line for business calls or for specific groups of people. You can use an alternate number on the same device as your main one, on a separate device, or it can be the phone number of another person who can contact you and give you information.
A mobile number that is not your primary number, but which you can use to get information intended for you, is an alternate mobile number.