How to validate a phone number using a JavaScript Regex
JavaScript Regex stands for “JavaScript Regular expressions.” It refers to a sequence of characters that forms a search pattern. By using it, you can validate phone number Javascript.
Here is the JavaScript Regex that you will use for phone number validation:
What does it mean? Let’s break it down:
- /^\(?: The number may start with an open parenthesis.
- (\d{3}): Then three numeric digits must be entered for valid formats. If there is no parenthesis, the number must start with these digits.
- \)?: It allows you to include a close parenthesis.
- [- ]?: The string may optionally contain a hyphen. It can be placed either after the parenthesis or following the first three digits. For example, (123)- or 123-.
- (\d{3}): Then the number must contain another three digits. For example, it can look like this: (123)-456, 123-456, or 123456.
- [- ]?: It allows you to include an optional hyphen in the end, like this: (123)-456-, -123- or 123456-.
- (\d{4})$/: Finally, the sequence must end with four digits. For example, (123)-456-7890, 123-456-7890, or 123456-7890.
How do you use Regex in JavaScript? Here is a function that you will use to validate phone number:
By using this function, you can validate phone numbers entered in this format:
(123) 456-7890
(123)456-7890
123-456-7890
1234567890
Validate a Phone Number Using a JavaScript Regex and HTML
To validate a phone number using the JavaScript regex and HTML, you just need to follow these steps:
1. Create an HTML file. Then add these lines:
2. Create a CSS file. Then insert this code:
3. Create a JavaScript file. Add these lines:
The output will look like this:
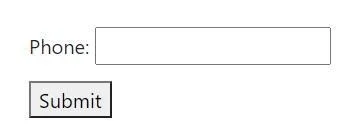
Now, if you add a phone number with the correct format, like (123) 456-7890, you will receive an alert showing “validation success”.
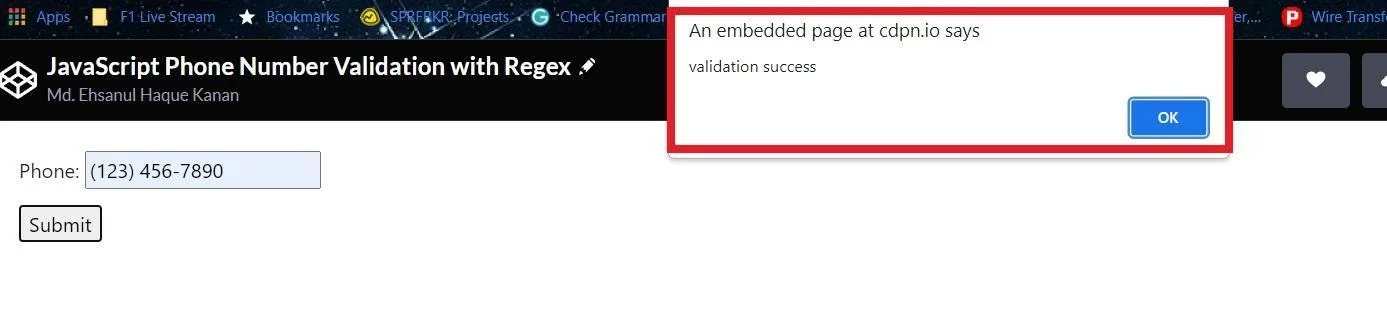
Validating a phone number with HTML5 “tel” input
A much better way for phone number validation from user input is using HTML5 tel input (<input type="tel">). It helps you to create input fields for phone numbers even in international formats. It is used as follows:
As you can see, the type attribute has been set to “tel.” Also, it has a pattern attribute, which takes a regular expression: "[0-9]{3}-[0-9]{3}-[0-9]{4}". It will be used during the phone number validation.
By using the HTML5 “tel” input, you can do phone number validation with shorter code. Also, smartphone browsers can optimize the input field. They can display a keyboard to the user, which is suitable for entering phone numbers conveniently. So, it’s a much better option.
How to Validate a Phone Number with HTML5 “tel” Input
1. Add these lines to your HTML file.
2. Then insert this code to your JavaScript file:
Here, you are using an event listener to display a custom error message. It will show up if the phone number input is entered in an incorrect format.
The output of the code will look like this:
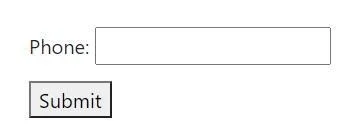
Let’s try adding an invalid phone number with incorrect format, like this: 01787965673. You will see a custom error message, which says “'Enter phone number in this format: 123-456-7890.”

Regional phone number formats
Using invalid phone number format can break integrations, cause data issues between platforms, and create duplicate records. It can make your phone number data very difficult to use manually. So, it’s very important to use regional phone numbers in the correct format.
Here are some of the most common regional phone number formats:
Cell phone numbers
Phone numbers in the United States consist of 11 digits — the 1-digit country code, a 3-digit area code, and a 7-digit telephone number. Let’s take a look at this example:

North American Variations
The format of North American phone numbers is a bit different. You have to enclose the area code in parentheses. Then you have to leave a space. Next, you have to hyphenate the three-digit exchange code with the four-digit number. Here is an example:

UK Variations
The UK phone number starts with a plus (+) sign, followed by its country code. Then you have to add the area code and leave a space. If you want to dial a number in London, you will have to follow this format:

Keep in mind that the area code of London is 20. If you want to dial a number in Oxford, you will have to use the area code 01.
International Dialing Codes
To format international telephone numbers in non-NANP countries, you have to add the country and area codes. You have to add a “+” sign immediately before the country code. Also, you have to separate the groups of numbers in the international phone number with spaces. Here is an example: +1 415 555 0132
While calling international numbers, you might face several dialing errors. The most common one is associated with the trunk prefix, which is a single-digit code that often starts with 0. It is added before the national subscriber number. However, you must leave out this code while dialing abroad. Otherwise, you will face issues.
Here is an example:
London landline dialed from the UK: 020 3465 5678
The same London landline number dialed from the US: 011 44 20 3465 5678
If you look closely, you will notice that the starting zero is left out from 20.
Another common issue is associated with area codes. Unlike the US and Canada, the cell phone numbers of most countries don’t use area codes. Instead, they utilize a unified national dialing format.
You should take a look at this website, which contains dialing codes and conventions of different countries around the world. By following this information, you can avoid the issues and properly format phone numbers.
Why you should validate phone number input
Validating phone number input is very important for security. It enables you to prevent fraudulent activities, like sign-up spam. So, you will never have to worry about fake customer registrations.
To fight against spam, you can use OTP verification technology. It will send a one-time passcode to the provided phone number to ensure the accessibility of the user. It helps you to ensure that your business is getting unique and genuine sign-ups.
Validating phone numbers with an API
You can use an API to validate phone numbers conveniently. It comes with all the necessary functions to check the validity. So, you don’t have to write any code from scratch. It can make the validation process very simple. Also, it can save you a lot of time.
You can try using Abstract’s Phone Number Validation and Verification API. It’s fast, lightweight, and very easy to use. You will only need the API key to check the validity of the client’s phone number. So, you don’t have to write extra code.
Here is an example:
If the phone number request is successful, you will see this output:
As you can see, "valid" field is set to “true.” That means the given phone number is valid. Also, it gives a variety of useful information, including the registered location, country code, and carrier. You can utilize this data to expand your outreach effectively.
Wrapping Up
Now, you have learned the way of validating phone numbers in different ways. The most efficient method is using a verification API (like with Node.js). It can make your life a lot easier.