How to Validate Mobile and Landline Phone Numbers in Laravel (6, 7, and 8)
This guide will demonstrate how to validate US and international phone numbers using two different methods:
- The Laravel Controller class
- Regex, or regular expressions.
Each method offers its own advantages and limitations, which we will explore in great detail as we work through each section of this guide.
If you want to learn how to complete email address validation using PHP as opposed to telephone number Laravel validation, check out this article on jow to validate an email address in PHP.
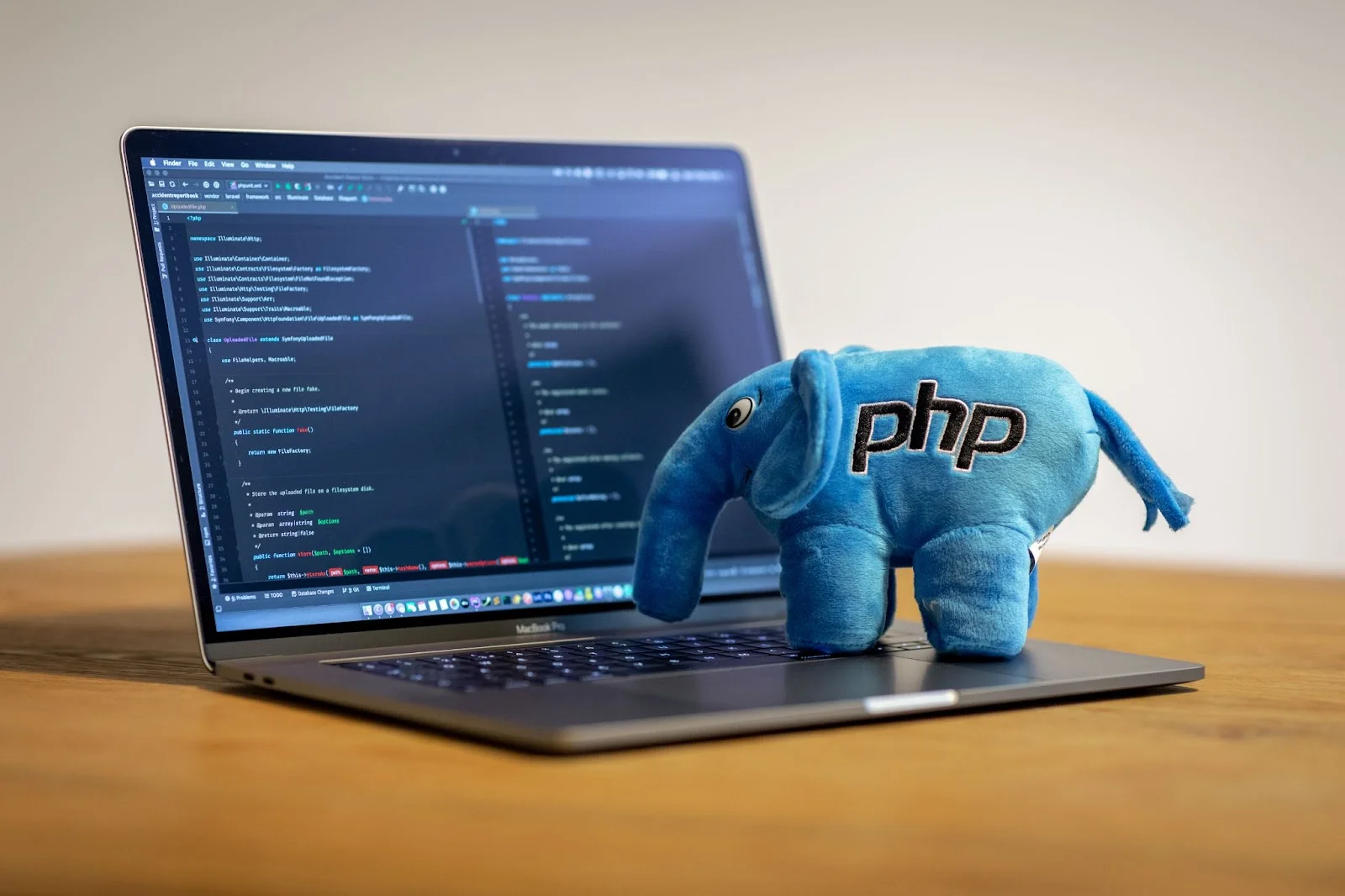
Laravel 5/6/7/8 validation
The Controller Validation method we will demonstrate in this guide will work from Laravel 5.1 onwards.
For Laravel 5.0, the framework did not provide the Controller class out-of-the-box. Laravel provided a class called Validator instead. If you are still using Laravel 5.0, you can learn more about this class from the official documentation for Laravel 5.0.
Laravel Phone number validation code example 1 - Controller
What is the Laravel Controller method for validation? Controllers can group related request handling logic into a single class. For example, a PhoneController class might handle all incoming requests related to a telephone number, including showing, creating, updating, validating, and deleting numbers. This is useful for performing form validation in your Laravel application, like when a new user is trying to register their details.
Using a Controller class saves you from having to define all of your request handling logic in a single routes.php file. This results in a more structured and organized code base that is easier to maintain. By default, Controllers are stored in the app/Http/Controllers directory.
We’ll look at using a Controller class that can successfully validate phone numbers. But what is the format of the numbers we are using? We will be handing both landline and mobile phone numbers for the United States. These numbers are 10 digits in length when the extension is included, eg.14151251234.
Setting up our Route
Let’s get started with writing our Controller class. Remember that Controllers save us from having to handle validation logic in our routes files. However, we still must add a route to our Controller class in routes/web.php with the following command:
This POST request will be used when the user submits their number.
PhoneController class to reject invalid phone number
Let’s create our PhoneController class. This class will act as a validator extension to the base Controller class. Examine the code below:
Let’s run through the code! The first thing you’ll notice is how the function called save corresponds to the Route we set up in routes/web.php:
Validate Phone Number Laravel
Let’s take a look at a validation example and the save method itself to see how to handle a phone number in Laravel:
In our save function we use the validate method provided by the Illuminate\Http\Request object. When a number is validated using this method, two different results can occur:
- If the validation rule passes, your code will keep executing normally.
- If validation fails because of an invalid phone number not following our new rule, an Illuminate\Validation\ValidationException exception will be thrown and the proper error response will automatically be sent back to the user.
If validation fails during a traditional HTTP request, a redirect response to the previous URL will be generated. If the incoming request is an XHR request, a JSON response containing the validation error messages will be returned.
Imagine how this would look to be used for something like form validation. The user would input an invalid phone number to the form and hit save, and an error message would be displayed through the UI informing the user they entered an incorrect number. The message would not be displayed immediately when the input starts, but when the save button is pressed.
Phone Validation Rule Breakdown
We provided the following validation rules: ‘required|numeric|min:10’. But what do each of these mean? Here are the definitions for each:
- numeric: The input must be numeric, ie. a number. Check out this documentation for what HP considers to be numeric.
- min:10: This field must be at least 10 characters in length.
- required: The field under validation must be present in the input data and not empty.
A field is considered "empty" if one of the following conditions are true:
- The value is null.
- The value is an empty string.
- The value is an empty array or empty Countable object.
- The value is an uploaded file with no path.
Okay, so the user must input a numeric value that has to be 10 characters in length. Compare this to the US number we displayed earlier: 14151251234. Our PhoneController will ensure the user input phone number is validated correctly.
However, what if you didn’t want to create a separate Controller class. What if you had familiarity with Regex and wanted to use a Regex pattern within the Laravel framework to add phone number validation to your application. This is possible, and in the next section, we’ll demonstrate how!
Laravel Phone number validation code example 2 - Regex
If you decide to use Regex to validate phone numbers, you won't have to use the numeric and digits rules we have previously shown. It is important to note that with Regex, you may find many different patterns that will suit your use case, there is no one accepted answer. Let’s implement a simple save method designed to store telephone numbers, and use a regular expression to act as our own validation rule.
Take a look at the following code and validation example where we use a function with the same name as our last example, save:
Let’s break down the rules we’ve applied to our phone_number input:
- required: this rule validates against null, empty strings, empty arrays, or uploaded files with no paths.
- regex:/^([0-9\s\-\+\(\)]*)$/
- This regex pattern accepts number 0-9, and the additional characters of '-','+','(', and ')'. Any input that does not match this given pattern will be rejected.
- min:10: This input must be at least 10 characters in length. Any input that does not follow these size validation rules will be rejected.
This is how you can use Regex for US number validation. But what about the international format for telephone and common mobile numbers? Let’s look at how we can alter what we’ve used so far to add phone number validation for different formats.
Laravel international phone number validation
There are a lot of different valid phone number formats as you can see from this Wikipedia entry on conventions for writing telephone numbers. You can see a mobile number may also differ from a landline number. A phone number format can change drastically depending on the country code and the extension for that number. A mobile number field may expect a different length than a landline number field. Handling all of these different cases can be tricky.
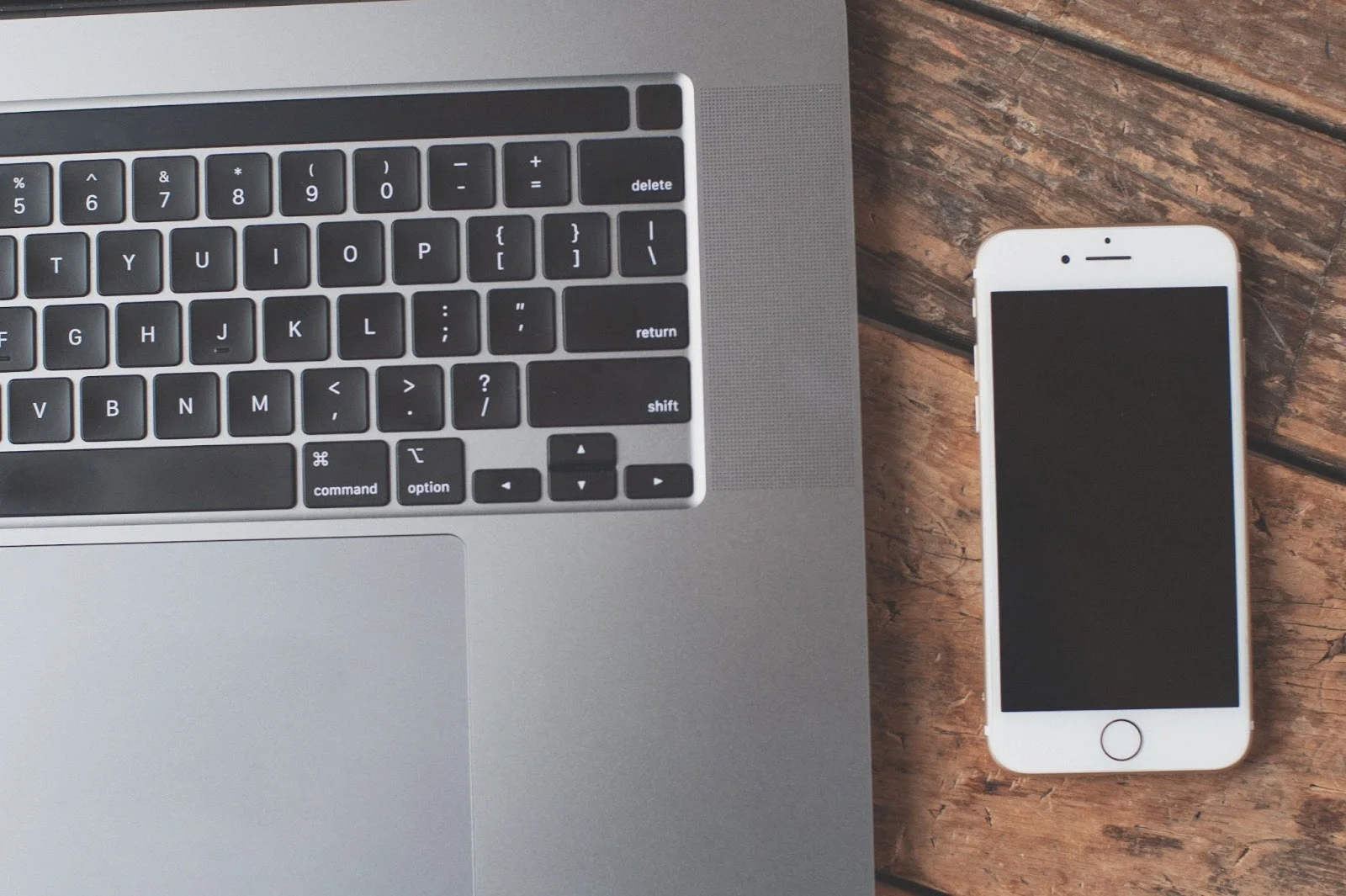
Let’s look at phone numbers in the United Kingdom as an example, and compare it with the US number format we’ve covered so far.
- US Phone Number - +1 213 621 0002
- UK Phone Number - +44 20 8759 9036
The US number follows this structure:
- Has an international extension of +1.
- Contains 9 digits following the country code.
The UK number follows this structure:
- Has an international extension of +44.
- Contains 10 digits following the country code.
Let’s look at our previous Regex example and update it so that it can complete validation with the country code for UK numbers.
You can use the following Regex pattern to validate a UK number:
What if you didn’t want to use Laravel’s in-built Controller method or Regex to perform your phone number validation? Let’s look at some alternatives we can use for validating phone numbers.
Alternative methods to validate phone number
There are some alternatives out there to the methods we have discussed so far. One such method is to use a library.

Using a library to check a number format
One popular alternative for performing phone number validation I recently discovered is Google’s libphonenumber. This phone validator package is a Java, C++, and JavaScript library for parsing, formatting, and validating international phone numbers. It can handle all countries and regions in the world and offers extremely useful functionality out of the box. If you need to add mobile validation, the Java version of the library is optimized for running on smartphones, and is used by the Android framework since 4.0 to validate a mobile number.
Using an API for to check phone numbers
Another alternative is to have an API act as a phone number validator. There are many terrific APIs, like this phone number validation API from Abstract, that offer lots of functionality. They are often easier to integrate into your existing workflows. This Abstract API can also detect a fake and invalid number from a non-existent service provider, this is functionality you will not get from any other solution previously mentioned.
That concludes our guide to phone number validation in Laravel, we hope you will take this information and apply it to your own unique scenario and project!