Why is Phone Number Validation Important?
These days, many apps and websites use mobile numbers to authenticate users, so validating phone numbers has become an important step in app development. Before you can send an authentication SMS message to a user, you need to make sure that the number they have given you is correct.
Validating phone numbers also helps your users avoid fraud and scams, and is critical for building a robust and accurate contact list. If you gather mobile numbers as part of your lead-generation process, you need to validate those numbers before you add them to your database.
Finally, having correct and accurate data for your users and contacts improves the ROI of your marketing campaigns and reduces bounce rates and wasted effort by ensuring that messages go to the right people.
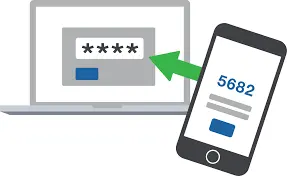
How Does Google Phone Number Validator Work?
Google’s libphonenumber is a library that you can download from Github and include in the code for your application or website. The package contains functions and methods that help you determine if a phone number is valid. It works for both domestic and international phone numbers.
While there are many packages and libraries out there to help validate phone numbers, using a package from a reputable company like Google means you can be sure that the code is robust and the methods work. libphonenumber is also the most popular JavaScript package for this procedure, meaning there are a lot of other devs using it who can answer your questions if you get stuck.
Finally, there are third-party ports and forks of this package that provide additional features or allow the use of the library in other languages like Ruby, Clojure, Python, Go, and more.
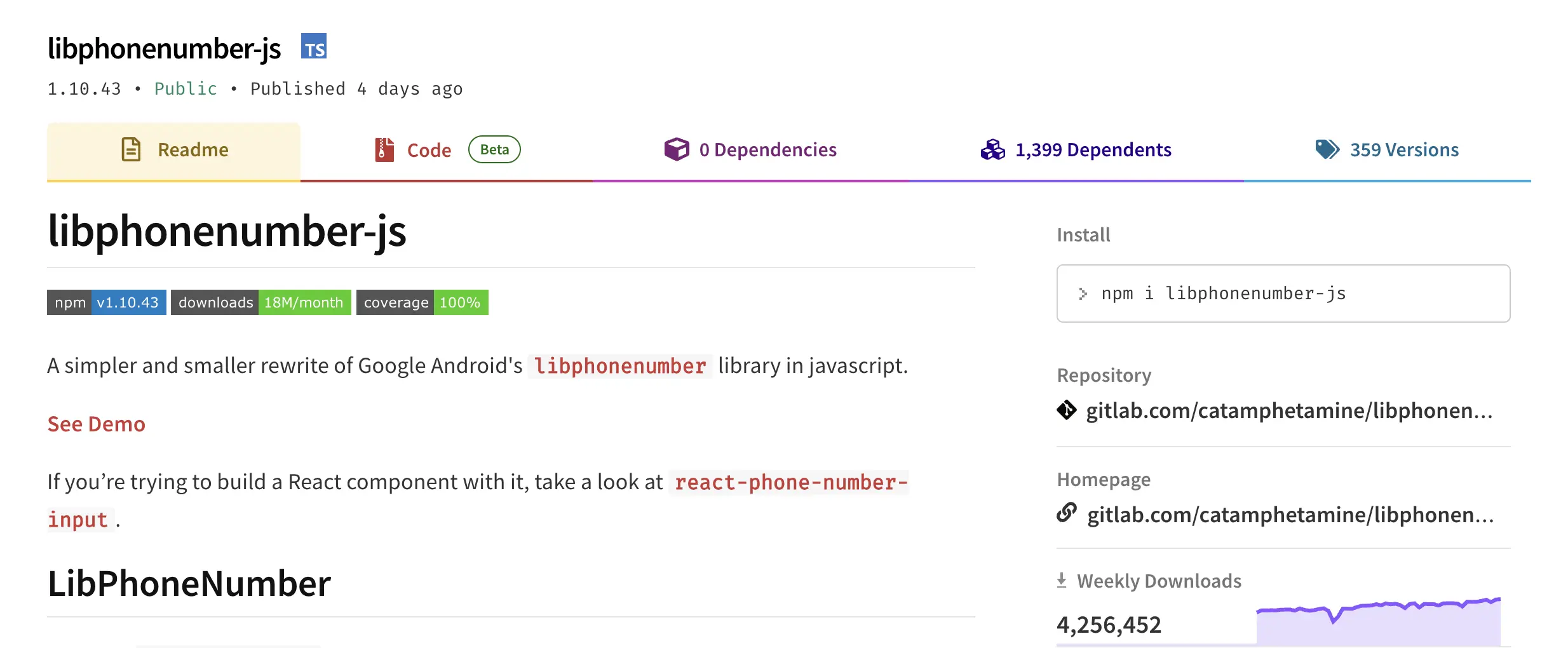
Steps to Use Google Phone Number Validation
Here’s a quick tutorial on how to implement libphonenumber in the code for your Javascript app or website.
Download and Install the Package
Download the package into your app using yarn or NPM.
Set Up the Phone Number Validation Utility
The package exposes a utility class, PhoneNumberUtil which has several methods for working with phone numbers. Create an instance of it like so:
Parse a Phone Number String
Before you can validate a number using libphonenumber, you need to parse it into a PhoneNumber object. Use the parse method to do that:
The method should accurately detect the country code, area code, and number. It takes an optional second argument, where you can provide the expected country string:
parsePhoneNumber creates a PhoneNumber object that looks like this:
Validate a Phone Number
Once you have a PhoneNumber object, you can call the isValid or isValidNumber method to validate the number. The method returns a boolean.
Under the hood, this method uses the isPossibleNumber method to first check the length of the string. Once it has determined that the length of the number is as expected for the given country, it uses the country prefix to validate the actual digits.
Additional Methods
libphonenumber exports many other methods for working with phone numbers, including methods to format number strings into national, international, RFC and other formats, and methods to geocode numbers.
Format a Phone Number
You can use the “as you type” formatter to format a number in a text input as the user enters the number. This is useful because it prevents users from trying to input formatting on their own, which can have unpredictable results.
Pass the input string to this from your text field to do real-time formatting as the user inputs the number. The method accepts options to format based on international format and all country formats.
AbstractAPI: The Best Alternative to Google Phone Number Validator
If you’d rather not import additional dependencies into your codebase (which can lead to increased bundle size and affect load times) an API is a great option for phone number validation.
An API is a remote codebase that exposes a REST endpoint and accepts a network request containing a phone number for validation. The API handles the work of validating that number for you. Once the validation is complete, the API returns a response object with the result of the validation.
Let’s a take a quick look at how to use the AbstractAPI Free Phone Number Validation API to validate a phone number.
Get an API Key
Before you can make a request to the API, you’ll need an API key to authenticate yourself. Go to the API documentation page in your browser and click “Get Started.”
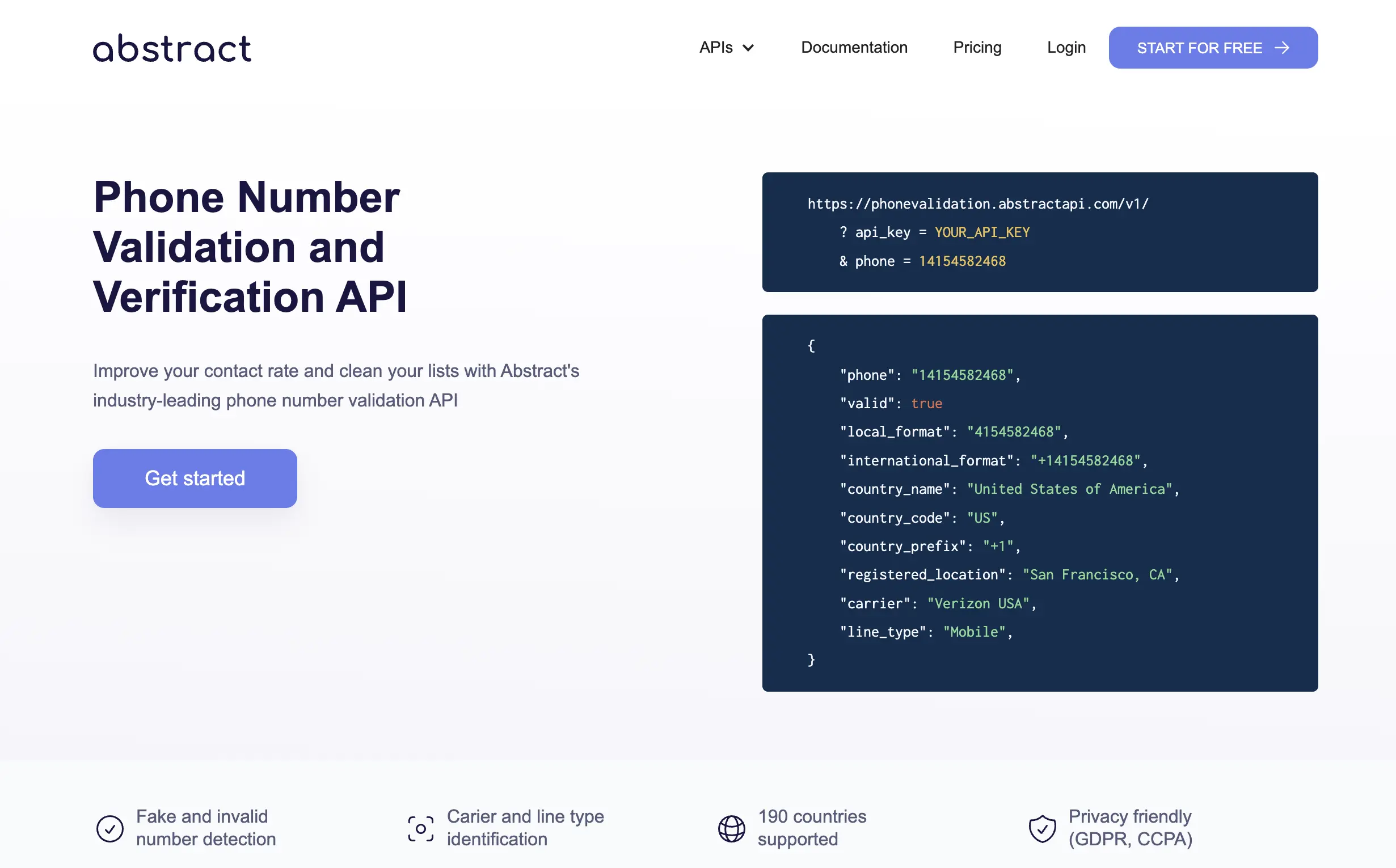
You’ll need to create a free account using an email and password, then you’ll be taken to the API dashboard where you’ll see your API key.
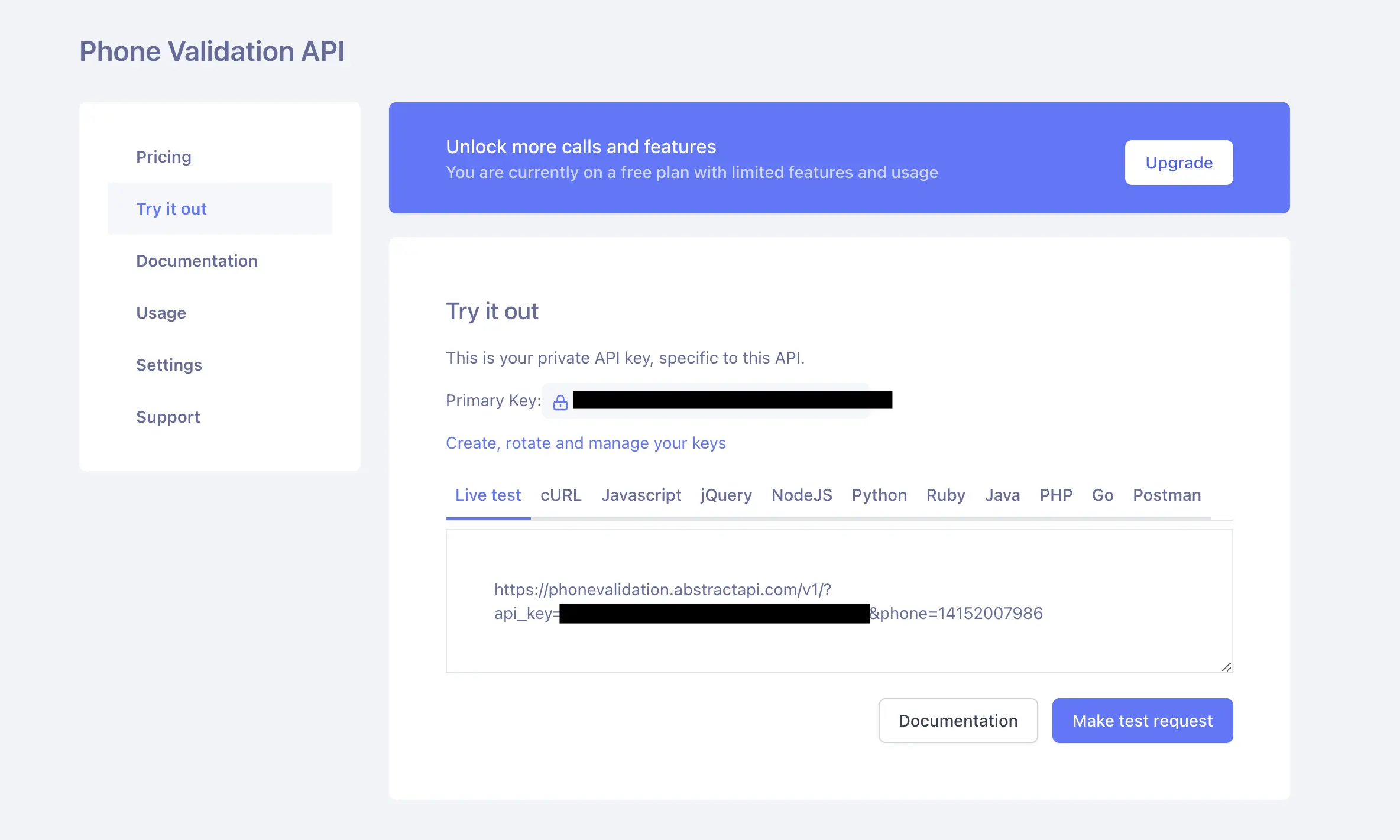
Test the API
You can use the “Make test request” button to send a test request to the API. Select the “Live Test” tab above the provided input box. Inside the box will be a URL including a query string with your API key and an example phone number.
When you click the “Make test request” button you’ll receive a 200 “success” status and a JSON response that looks like this:
The response object contains the number, a boolean to tell you whether it is valid, and some additional metadata including the carrier and formatting options.
Use the Provided Snippet in Your App
AbstractAPI provides code snippets for all the major programming languages. Select your preferred language from the tab above the textbox and click “Copy Code.”
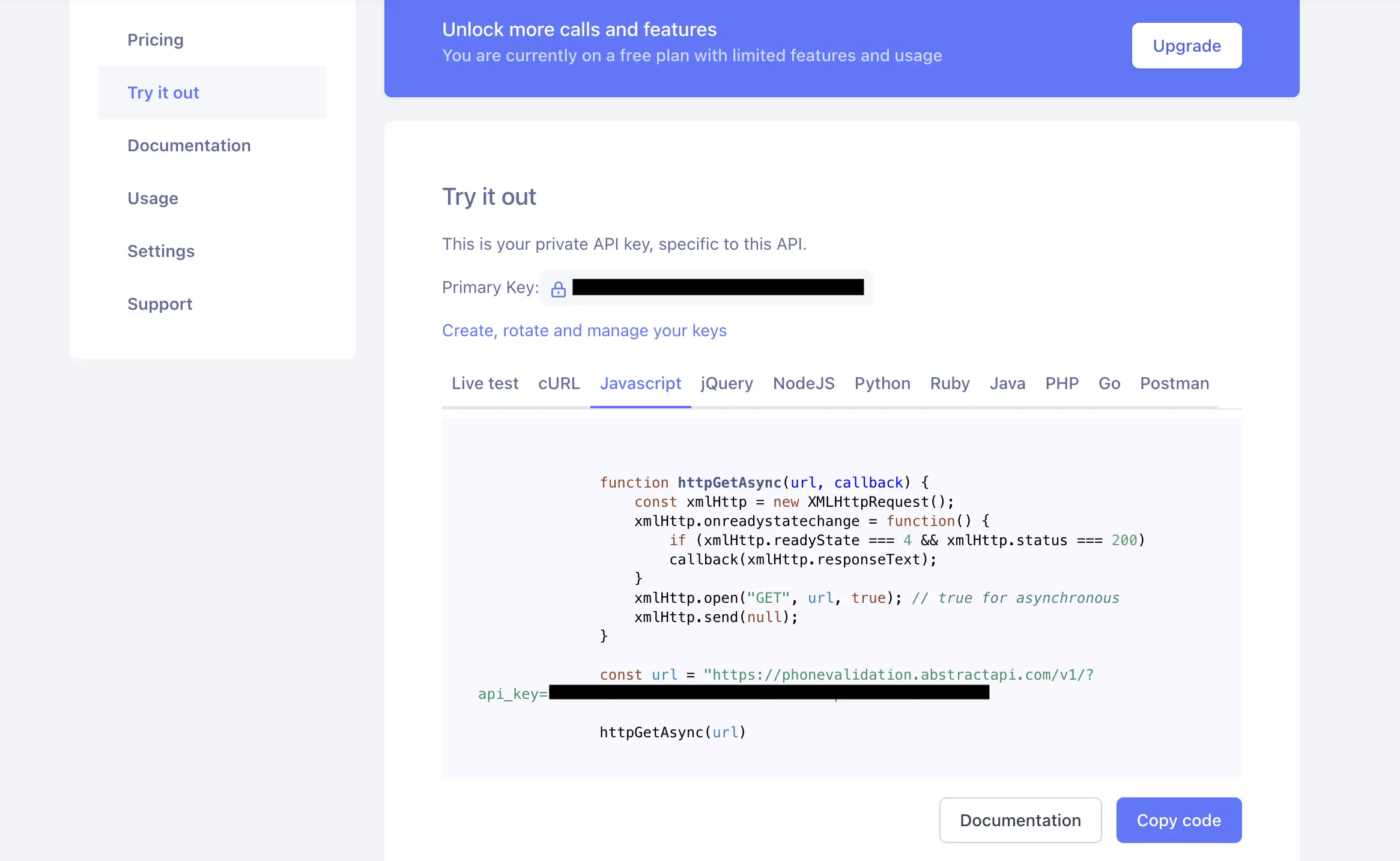
Paste the snippet into your JavaScript, Python or other language file to send the request from your own app.
Frequently Asked Questions (FAQs)
How do I do basic phone number validation?
The easiest way to do phone number validation in your app or website is to use a validation API like AbstractAPI’s Free Phone Number Validation API. Using an API means you don’t need to import any additional dependencies to your app, or write any code yourself. You can just send a simple POST request to the API endpoint along with your API key and the phone number you want to validate. The API will send back a response with all the validation information.
You could also use Regex to check if a phone number is valid, but doing so is not recommended. It is very difficult to write a regular expression that captures all valid formats of international phone numbers.
How do you check if a number is real?
The easiest way to check if a number is real is to call it. If you are building an app that needs to validate a phone number, however, calling the number is not an option. In this case, you can use a package like Google’s libphonenumber library or an API to validate the number.
How can I verify a phone number for free?
Use AbstractAPI’s Free Phone Number Validation API. You can easily sign up for a free account and use the test sandbox on their dashboard to send a test request to their API endpoint with any phone number you want to validate. The API will send back a response telling you whether or not the number is valid, along with other information about the number.
Conclusion
Phone number validation is a non-negotiable step for apps and websites that want to use SMS verification for their user authentication. Beyond that, phone number validation provides security for your users and improves your data accuracy for your contacts and mailing lists.
Using Google’s libphonenumber library or AbstractAPI’s Free Phone Number Validation API are two quick and easy ways to implement phone number validation in your Android or iOS app or website.
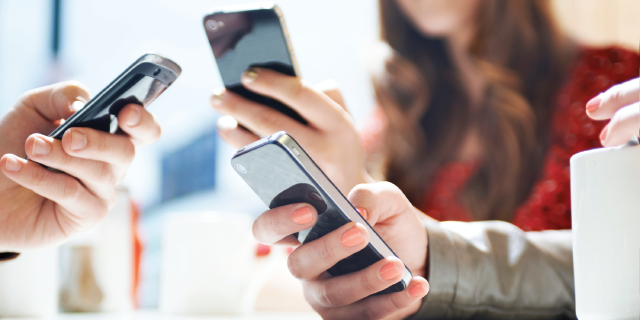