Getting Started With Django
In order to use Django, you’ll need to have a Python installation on your machine. We recommend Python3. We’ll also be using a virtual environment to run the Django app. We’ll explain a bit about what a virtual environment is, but if you want more information, check out this guide.
Spin Up a Virtual Environment
The first thing we’ll do is create a project directory and spin up an environment inside it. It’s easiest to keep your virtual environments inside the same folder as your projects to make it easier to know which environment goes with which project.
What Is a Virtual Environment?
A virtual environment is like a container for your Python or Django application. It’s similar to a Docker container. It keeps all your project dependencies and Python installations for a particular contained in one place without affecting the global scope of the machine that runs the application. You can run multiple different virtual environments on one machine, each with its own version of Python and different versions of various dependencies.
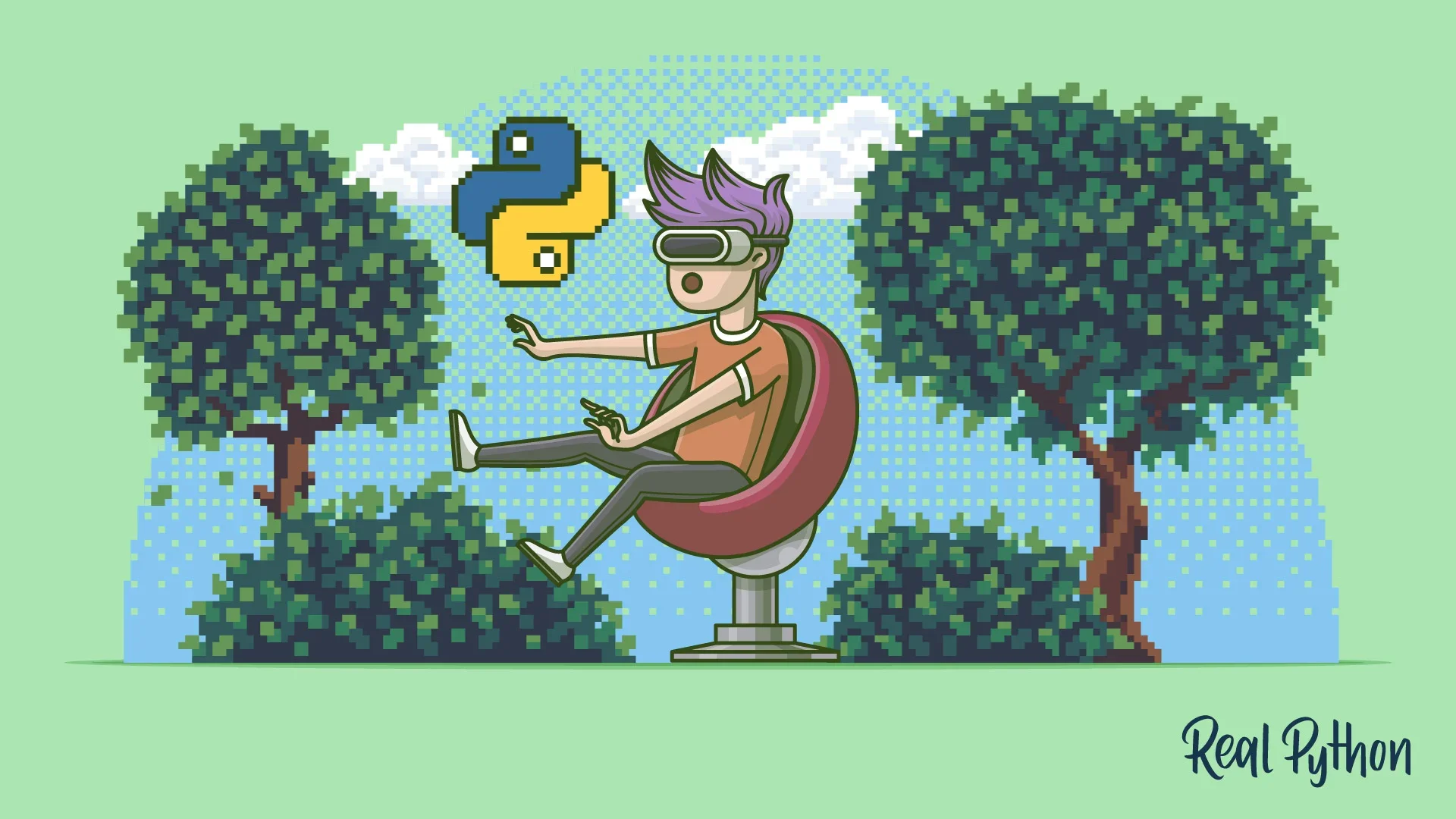
Keeping these dependencies in their own containers means the versions and all the packages remain separate.
Run the following commands to get started
Let’s quickly break down what each of these commands does.
Creates a new project directory and navigates into it.
Uses the Python3 installation (you can use Python or Python2 if you prefer) to create a new environment. The -m argument tells Python to use a particular module, and the first venv argument is the module we tell it to use.
The second venv argument tells Python what to call the folder that the environment information will be stored in. You can call this anything you want, but venv is standard and will make it easier for other people who look at your code to know what it is right away.
This activates the environment inside the current shell. Once you run this command, you should see the following output in your terminal
That indicates that you are inside the environment. Great! Now it’s time to install our dependencies.
Install Dependencies With Pip
Pip is Python’s package manager, similar to NPM or Yarn for Node. When we install packages inside the environment, they will only exist within that environment. For this project, we’ll only need django.
Make sure you are inside your environment and inside the project directory, then run the following command.
Create a New Django Project
We’ll use the django-admin command line utility to create a new Django app. This utility comes with Django, so it should work now that you’ve installed it.
This will create a new Django project inside your project directory, next to your venv environment folder. Name the project the same as the parent project directory. Open the project in your favorite code editor or IDE (VS Code is a good one) to start.
Create a New Django Application
The last setup step in getting up and running is to create a new application. Django projects are made up of many “apps.” You might break your project into several apps if it does a few different things. For example, you might have one app to handle authentication and sign-in. You might have another app to handle notifications, and another to manage the primary logic of rendering views and flows.
For now, we’ll just have one very simple app that renders a webpage where we can view geolocation information about IP addresses. Run the following commands to create that app inside your project.
The manage.py startapp command tells Python to start up an app called geolocation. If the app doesn’t exist, it will be created.
Register the Geolocation App With the Main Django Project
In order for the main project to know that the app exists, it must be installed in the main project’s settings.py file. Open that file in your editor and add a line to the INSTALLED_APPS array.
For reference, here is what your project structure should look like now.

Create a Basic Django View
A Django view tells Django how to render the information. Let’s create a simple view that just returns some text about our Http Response.
Add the following the views.py file inside the geolocation application directory.
When a user requests the page that renders this view, it will simply return a string of text that says “Welcome!” In most cases, your Django application will also include an HTML template that the view will render. For our purposes, however, we don’t need one.
Next, let’s tell Django what URL we would like to render this view at.
Render the View at a URL
Create a file called urls.py inside the geolocation app directory. There is already a urls.py file inside the DjangoIPGeolocation main application folder, but we’re not using that file. We’ll come to that in a moment.
Add the following to geolocation/urls.py.
This tells Django that inside the geolocation app, at the root path of the URL, we should render the home view that we just created.
Next, we need to tell the main DjangoIPGeolocation application to render the geolocation app at a particular URL. Open the urls.py file inside the main DjangoIPGeolocation directory and add the following.
You’ll notice that the only things we actually added here are the include import, and the new path variable inside the urlpatterns array. This will tell Django to render our geolocation app at the root path of the main application.
Let’s run the application and take a look at the page in the browser.
You will see a warning in the terminal that you have unapplied migrations. Don’t worry about this for now. Visit localhost:8000 in the browser to see your application.
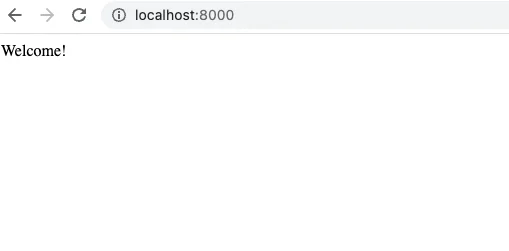
Great! Our application is up and running.
Finding an IP
Next, we want to grab the IP address of the user who is visiting our site. We’ll use the IP to get geolocation information about the user.
Open up the views.py file inside the geolocation app and update it with the following code.
Let’s quickly break down what this does.
The HTTP_X_FORWARDED_FOR header is metadata on the incoming request that contains the IP address that the request was originally sent from. We can pull the header off the incoming request by accessing the META field of the request. Then, we pull the IP out by splitting the HTTP_X_FORWARDED_FOR header string.
In case there is no IP address in the HTTP_X_FORWARDED_FOR header, we fall back to using the REMOTE_ADDRESS header instead, which should contain the IP of the most recent proxy that handled the request.
Go back to localhost: 8000 to see the IP rendered. Because we are doing local development, we see the loopback address, or localhost address, or 127.0.0.1.
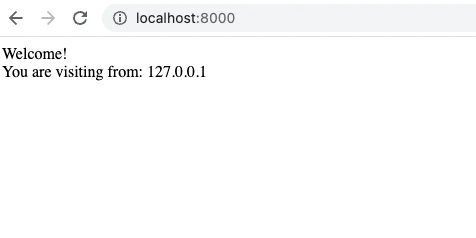
Getting Geolocation Information From an IP Address
Now that we are getting the IP, we can use the Django rest framework and the AbstractAPI Free IP Geolocation API to get geographical information about the user. We can send the IP address that we pull from the incoming request to the API, and get back a JSON object from the rest API with the following information.
I’ve redacted some of the information for privacy’s sake.
Get Started With the API
In order to use the rest API, you’ll need to sign up for a free account and get an API key.
Acquire an API Key
Go to the AbstractAPI Free IP Geolocation API homepage and click on "Get Started."
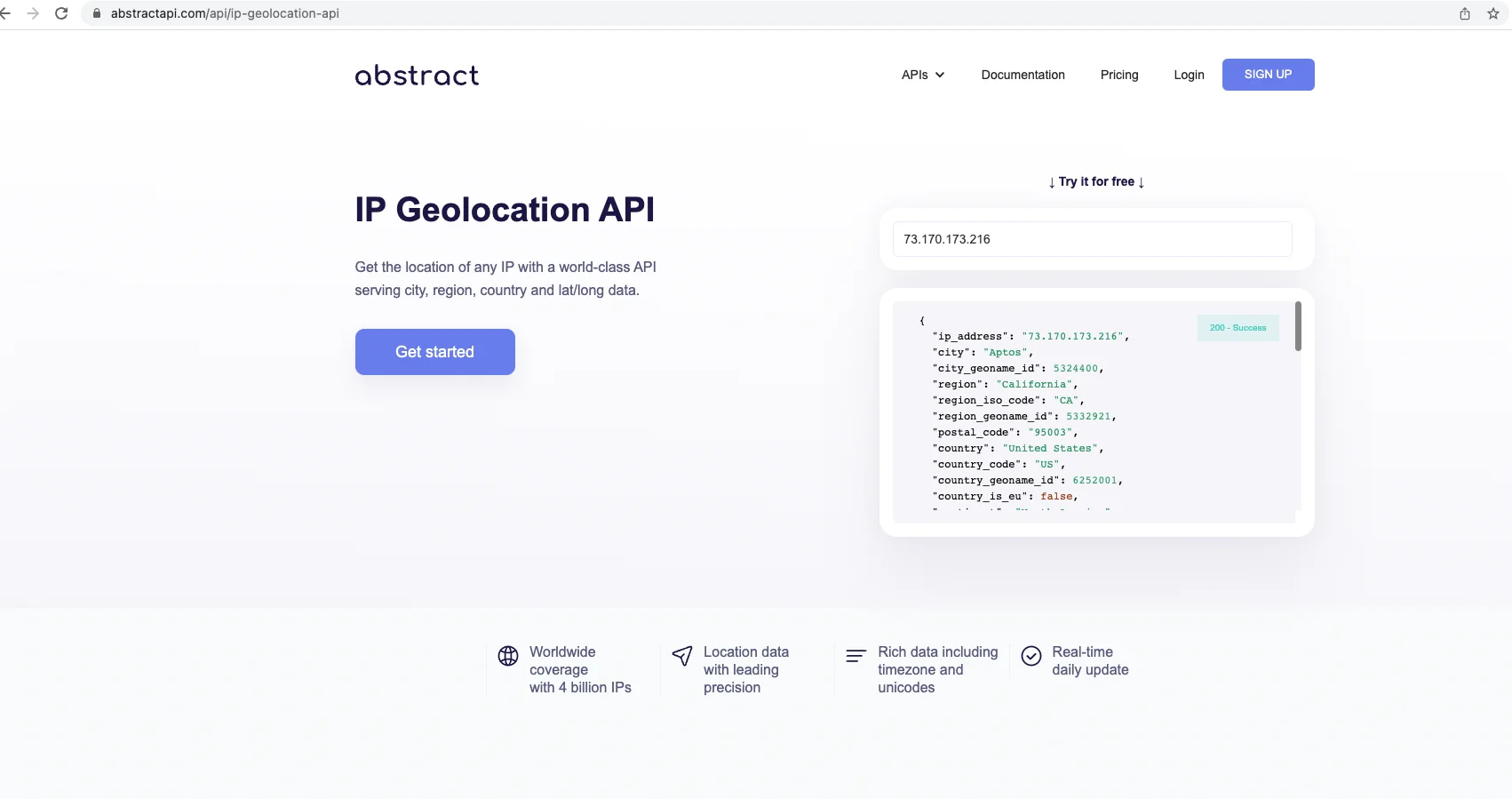
Once you’ve signed up or logged in, you’ll land on the API’s dashboard, where you’ll see your API key and links to documentation and pricing.
Send the IP Address to the Rest API
We'll use the Python programming language requests module to send a request to the AbstractAPI endpoint, passing it our IP address. You'll need to grab your API key and the AbstractAPI URL from your API dashboard.
Inside your views.py file, import the requests module and write a function called get_ip_geolocation_data that accepts an IP address as a parameter and sends it to the API.
Now, call the function after you pull the IP address out of the incoming request. When you visit localhost:8000, the view will send the request to the API and print the output in your terminal.
Unfortunately, all you’ll see is the following information.
Why is that? Well, the address we just sent to the API is the loopback address (127.0.0.1), which can’t be traced to any particular geographical location. Every computer has the same loopback address. The loopback address can’t uniquely identify a machine to the network.
So, for the purposes of this tutorial, we’re going to bypass sending the address that we pulled from the incoming request, and instead, simply send the public IP address of our machine. To do that, all we need to do is remove the ip_address parameter from the query string when we send our request to the API.
Rewrite the get_geolocation_data function to the following
When the API receives a request with no IP address passed, it falls back to using the IP address of the machine that sent the request. Keep in mind that this will not work in production, because you are simply sending the IP address of the machine that is hosting the application, not the IP address of the user who is accessing the application. For testing, however, this works fine.
Visit localhost:8000 again and check out the new output in the console.
Again, I’ve redacted some information.d
Render the User’s Location Information
Now we just need to add the information to the string that renders in the browser. We will need to parse the incoming data from JSON into a format that Python can read. To do that, we’ll use the json module. This will turn the response data into a dict. We can then access fields on that dict using square bracket notation.
Update views.py with the following code.
Head back to localhost:8000 in your browser to check out the new information.
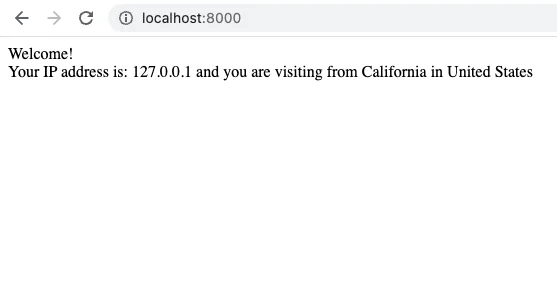
Neat! We’ve used the user’s IP address to look up their geographical information.
Conclusion
In this tutorial, we learned how to get started with Django and make API calls to the AbstractAPI Free Geolocation API. We built a basic application that uses a user’s IP address to determine the user location and show them information about their location.
The next step of this process would be to render the information inside a template, which would allow us to add more style to the webpage.
FAQs
What is Geo Django?
GeoDjango is a framework for working with geographic data in Django. It allows you to do things like finding the distances between two points on a map, finding areas of polygons, the points within a polygon, etc. For doing basic geolocation lookup, such as from an IP, GeoDjango is a bit overkill. You can use any third-party API or a smaller, more basic package like django-ip-geolocation to do this.
How Do I Use Google Maps in Django?
Django provides a package that integrates with the Google Maps API. The package is called django-google-maps and provides basic hooks into the Google Maps v3 API. The package allows you to create models that accept address and geolocation information and then renders them on a Google map.
What library do you need to work with geolocation in Django and Python?
There are several libraries that can do geolocation for both Django and Python. For Django, these include django-ip-geolocation, GeoDjango, and Django GeoIP2. Python can do geolocation from an IP address using the GeoPy or geolocation-python packages.
You can also use third-party APIs such as the AbstractAPI Free Ip Geolocation API to lookup geolocation information for a specific IP address.