Get Started With the API
The AbstractAPI Image Resize API allows you to compress, convert, resize, and crop your images through an easily accessible, API key-protected REST endpoint. The API allows two methods for working with an image: a direct file upload to the API through multipart form data, or a simple POST request containing the URL for a hosted image.
In this tutorial, we’ll work with an image already hosted in an AWS S3 bucket:
https://s3.amazonaws.com/static.abstractapi.com/test-images/dog.jpg

This is an image provided by AbstractAPI to use when testing the endpoint.
Acquire an API Key
In order to gain access to the API, you’ll need an API key. Navigate to the Image API homepage and click “Get Started.”

If you’ve never used AbstractAPI before, you will need to create a free account using an email address and a password. Once you’ve logged in, you’ll land on the API dashboard.

The dashboard contains links to documentation and pricing, settings and usage reports, as well as your API key.
Resize an Image
First, let’s look at the process of resizing an image using the API. Because the API operates through a simple REST endpoint, it is language-agnostic. It’s not necessary to install an SDK or any other kind of library to interface with the endpoint. A POST request with the image URL is all that’s needed.
Let’s assume you’re sending your request from a React frontend as part of a serverless app such as a Gatsby site. The code you would use to send the request would look like this:
When we log the data object, we will see something like the following:
In this case, because we set the resize options to resize just the width and use the auto strategy, the image will be resized to the specified width and the aspect ratio will be maintained. Other options include the ability to resize the image to the exact height and width given, without maintaining the aspect ratio, or to crop the image to fit into a given width and height (through the exact, and fit strategies.)
The image quality will not be affected by this process. AbstractAPI provides a lossy option to specify whether you would like the API to use lossy compression to compress the file size. If the option is omitted, it defaults to false. When false, the image filesize will still be reduced a small amount (about 10-20%) but there will be no affect on the image quality.
Compress an Image
Let’s now take a look at simple lossy image compression using the AbstractAPI endpoint. Keep in mind that the API only provides the option for lossy image compression, so when compressing any image in this way, there will be some small decrease in the image quality.
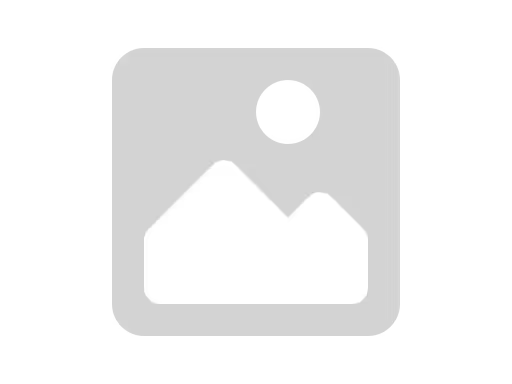
Update the request method we built in the previous example to request image compression rather than resizing.
Here, we’ve set the lossy boolean to true, and specified that we’d like to maintain an image quality of 75%. Generally, AbstractAPI recommends that you do not provide a quality option unless you know what you are doing. If the quality option is not provided, the API will determine the quality output intelligently. Specifying a quality of over 95 could result in a larger image than the original, while anything less than 25 will result in an unusably low-quality image.
When we log the data, we will see something like the following:
As you can see, there has been no reduction in image size (as evidenced by comparing the original_width and final_width and original_height and final_height.) There has, however, been a decrease in the size of the file, of about 130458 bytes.
Conclusion
Using APIs for image compression and manipulation is an excellent way to build low-code and no-code solutions for image processing apps. In this how-to-guide, we looked at using one such API—the AbstractAPI Image Processing and Optimization API—to both resize a hosted image and compress that image using lossy compression.
FAQs
How Do I Resize An Image In Code?
There are many ways to resize images in code, and the method you choose will depend on the language you are using, and what the resulting image will be used for. If you are simply resizing a loaded image for display, setting the height and width attributes via CSS or HTML may be enough.
If you need to resize an image for upload, using a library like react-image-file-resizer, or an API like AbstractAPI’s Image Processing API are excellent solutions. If you need to resize an image on the backend, APIs also work, or there are many image processing libraries available for various languages.
What Is An Image API?
In programming, API stands for “Application Programming Interface” and it refers to any library or hosted solution that helps a developer complete a task in code. Usually, an API is a remote endpoint that you access through some kind of network request, send your data along for manipulation, and receive a response.
An image API is an API that receives requests with image data, and performs some kind of operation on that data (for example, resizing, compressing, cropping, filtering, etc.) and then returns either the new image file, or a link to the new image hosted somewhere.
How Can You Define Image Size in HTML?
While HTML itself does not provide a way to dynamically manipulate image size, it does allow developers to set specific width and height attributes for images. These attributes dictate how an image is displayed within a webpage but do not alter the actual file size or resolution of the image.
However, manually setting width and height can sometimes distort the image's aspect ratio if not handled correctly. To ensure images maintain their proportions, it's best to use CSS properties like max-width
, height: auto
, or object-fit
, or leverage responsive design techniques such as using srcset
for adaptive image rendering across different screen sizes.
For true image resizing—where the actual file size is reduced—developers should consider using server-side processing or image manipulation APIs to optimize image delivery and performance.