Considerations for Web Scraping using PHP
In order to scrape a single web page, PHP has the built-in cURL library. It is one of the most popular libraries out there and is available by default.
However, most web scraping tasks are not limited to a single page. A typical web scraping project entails scraping an entire website. This requires crawling all the pages of the website and then spawning additional web scraping requests to fetch all the pages.
This also brings in one more consideration, which is the load on the server. Performing web scraping within the PHP code directly results in some performance overruns, since crawling a large website and scraping every page is an involved activity. Therefore, sometimes it is better to offload the web scraping chores to a third-party service.
Based on the above concerns, there are three approaches to achieving web scraping using PHP:
- Using a Built-in Library: For simple and one-off webpage scraping tasks, it is better to use a built-in PHP HTTP client module. This is the simplest option and ideally suited when crawling the website is not a requirement.
- Using a Scraping Library: A web scraping library specifically designed for crawling websites. This helps in identifying the internal links to web pages and streamlines the filtering of HTML page contents from crawled URLs. For web scraping tasks involving moderate to large websites, this is a good option.
- Using a Web Scraping API: If your PHP application has to undertake large-scale web scraping projects, then continuously extracting web pages from within the PHP code leads to a lot of performance overheads. It results in deteriorated server performance when that PHP application has to also handle other requests from the user. In this case, it is better to leverage an external service for scraping the web pages.
We will show you how to perform web scraping using all these approaches, along with sample code in the form of PHP scripts. Therefore, if you want to follow along, ensure that you have the PHP8 runtime available in your development environment.
Approach 1: Scraping a Web Page using cURL
cURL is a widely used built-in PHP library for performing web-based operations, including API handling, or fetching a web page. It ships as a PHP module and is mostly enabled in the PHP runtime.
You can create a simple PHP script to scrape a web page using cURL. Create a new php file named php_curl.php and add the following code:
This script takes the URL to be scraped as an argument and calls the init_curl() function which handles the scraping task. Inside it, the two library functions curl_init( ) and curl_exec( ) are called with appropriate parameters to send HTTP requests for fetching the content of the web page pointed by the URL.
An additional error handling code snippet is added to check for valid URL format, as well as response status. Moreover, checks are also added at the beginning to make sure the cURL PHP module is installed. In case your PHP environment does not have it installed, you can enable it from the php.ini config under your PHP installation directory.
Here is how you can invoke the script.

And the scraped web page is displayed in the terminal.
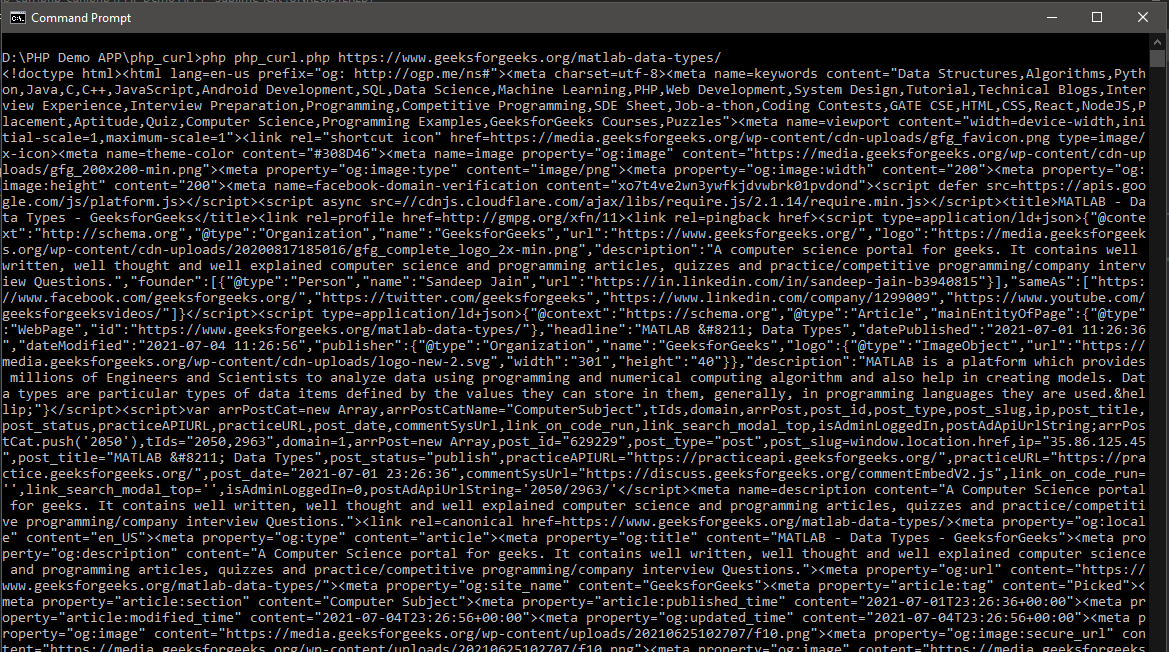
Approach 2: Scraping Web Pages using Goutte
Goutte is a popular, open-source PHP web scraping library. Using Goutte, you can crawl an entire website and define filters to scan and extract specific web page content.
Check out the official GitHub repository for Goutte to install the library under your PHP environment.
Create a PHP file named php_goutte.php and add the following code:
This code imports the Goutte Client and initializes it with HTTPClient to initiate a GET request on the URL. The URL to be scraped is passed as an argument.
Save the file and invoke the PHP script.

And you get a response containing the URL content.

The above script was a very simple demonstration of web scraping using Goutte. However, Goutte can do much more because it has some intelligent features to perform screen scraping to navigate to specific links on a web page and also scrape data by filtering simple HTML DOM elements and attributes. It also supports form submission, so it is possible to use it on websites where the content is available behind an authentication wall.
Approach 3: Scraping Web Pages using Abstract Web Scraping API
For large-scale scraping projects, it is better to leverage a service that offers a proxy to distribute the scraping requests globally. Abstract Web Scraping API is one of the most reliable options for this purpose. It supports millions of proxies and IP addresses from across the globe and offers customizable extraction options.
To access this API, signup for your free Abstract account to get access to all the APIs. Once logged in, you can access the Web Scraping API from the dashboard.
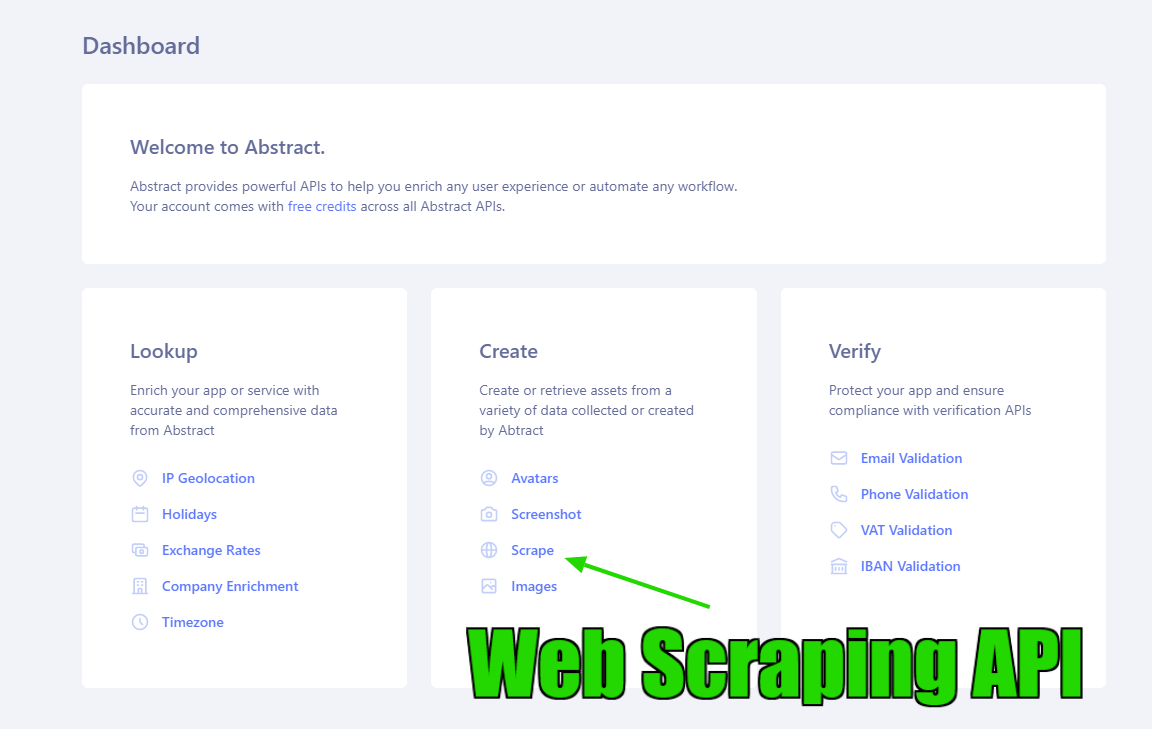
Once you access the Web Scraping API console, you can see your API primary key.
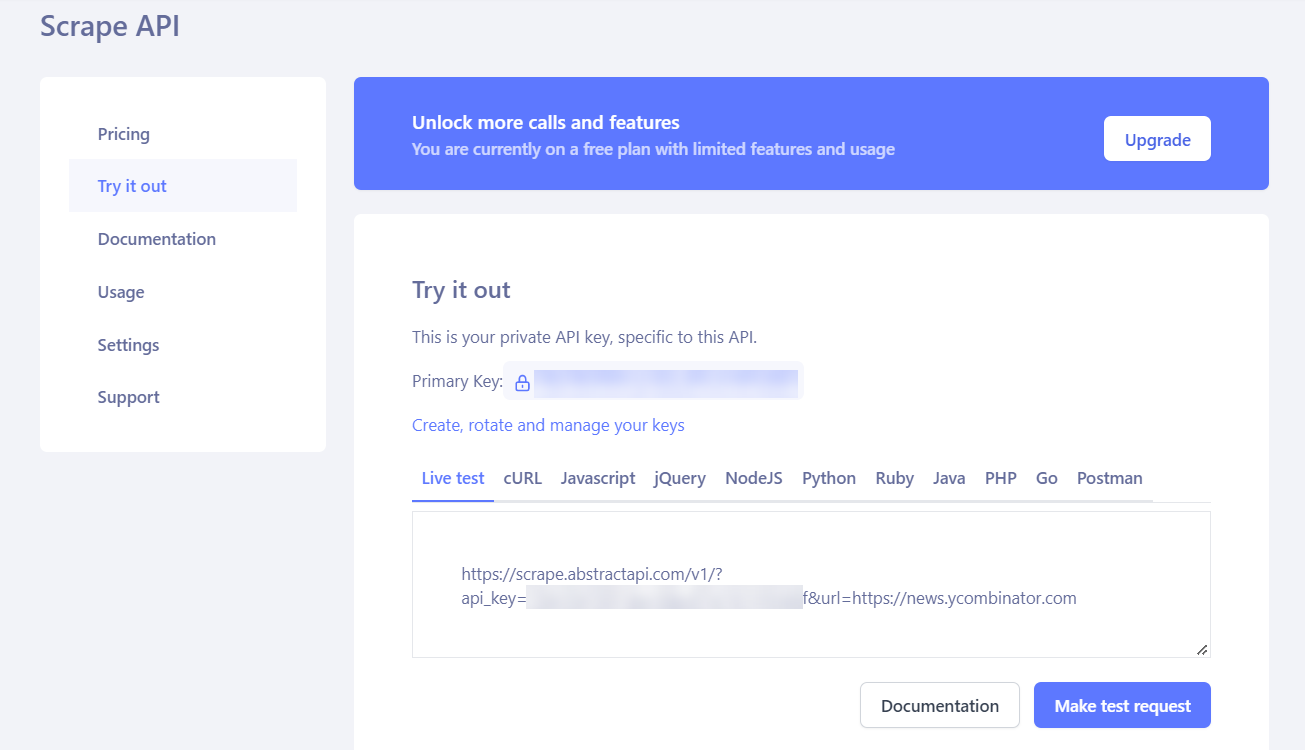
This is a unique key generated by Abstract for your account. Make a note of this key. You can try the live test to see how the API responds after extracting data.
Let's use this API inside a PHP script. For this, create a new PHP file named php_abstract.php and add the following code:
Before saving this file, ensure to replace the <YOUR_ABSTRACTAPI_KEY> with the primary API key allotted to your Abstract Web Scraping API console.
This code is very similar to the earlier approach of using cURL. However, the key difference here is that cURL is used to make HTTP requests to the Abstract Web Scraping API instead of directly fetching the content from the URL.
This is an important consideration for a real-world scraping project. That’s because this approach ensures that the scraping requests are processed by proxies maintained by Abstract API instead of exposing the server IP addresses where the PHP cRUL request is sent.
You can run this script in the same way as the earlier cURL approach.

Now, the Abstract Web Scraping API does the heavy lifting of scraping the URL and the cURL library captures the API response, which is finally displayed by the script.
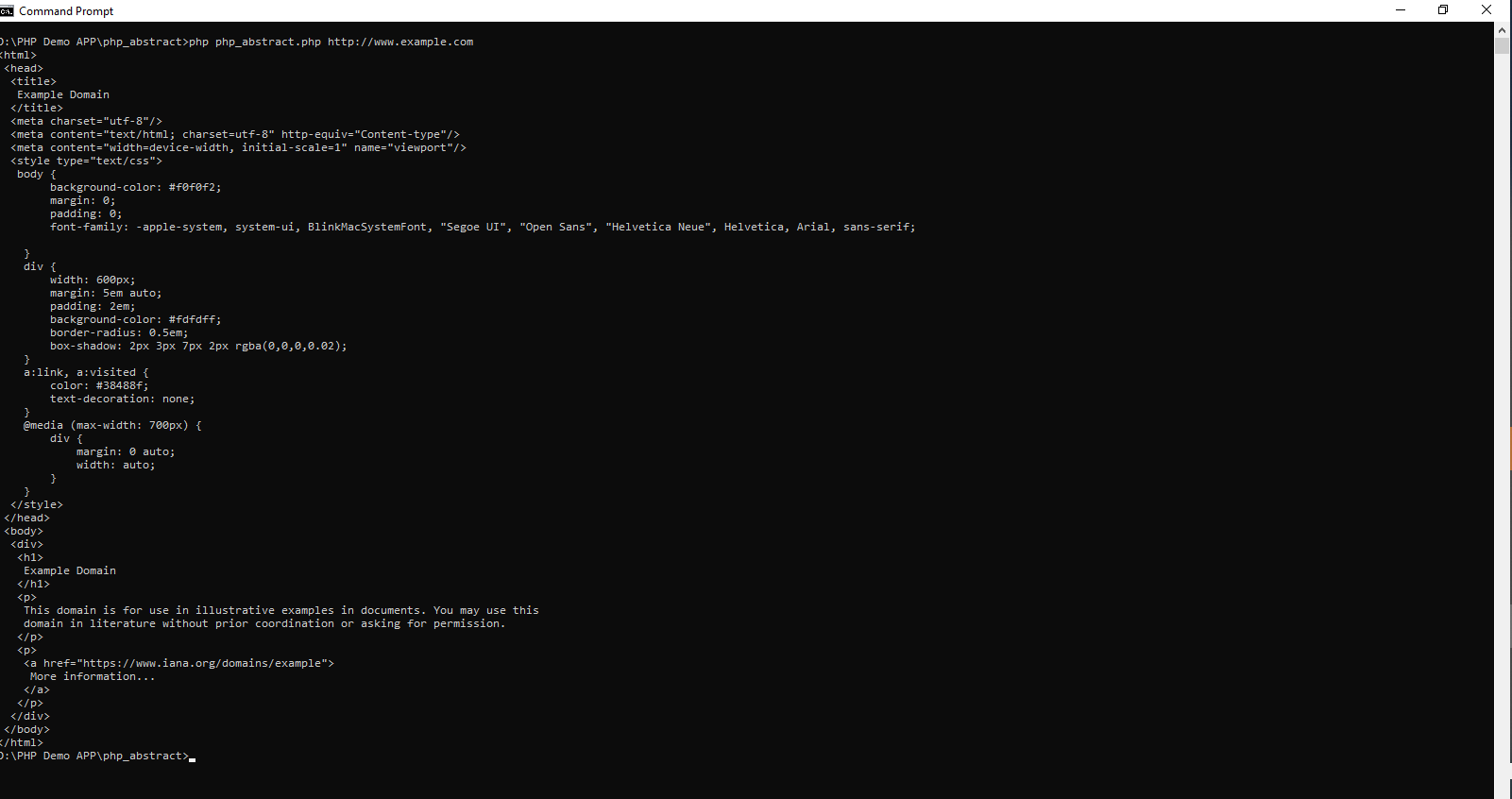
FAQs
Can PHP be used for web scraping?
Yes, PHP can be used for web scraping. PHP as a programming language for the web offers a few options for scraping a web page. For small scraping tasks, developers can use the built-in cURL library. PHP also has a rich ecosystem of web scraping libraries such as Goutte which offers some intelligent options for crawling the internal links of a website to scrape selected content. You can use this library in conjunction with an external scraping service such as Abstract Web Scraping API to massively distribute the web scraping requests across millions of proxies.
How to scrape a website using PHP?
PHP offers built-in support for web scraping. Using the cURL module, developers can pass URL arguments and extract the contents of the web page. However, note that this is a very basic form of web scraping, which does not scale well. For scraping entire websites, there is a better option than cURL, such as Goutte which is a web scraping library designed to crawl an entire website. Additionally, you can also use an external service, like Abstract Web Scraping library to massively distribute the scraping requests across millions of IP addresses.
How to get data from another website in PHP?
You can extract data from another website using one of the PHP web scraping libraries. For extracting the entire content of a web page, a cURL request does an excellent job. In case the requirement is to filter the data based on certain conditions, such as HTML tags, you can use one of the PHP libraries specifically designed for web scraping. Goutte is a popular choice for web scraping using PHP. Additionally, you can also use an external web scraping service, such as the Abstract Web Scraping API. With this API, you can scale the scraping request to many proxies and IP addresses to prevent the other website from blocking your PHP server’s IP address due to too many scraping requests.