Create a React app and Install Dependencies
This tutorial assumes a basic understanding of React and Create React App. If you don’t know how React or Create React App work, check out this tutorial on React, and this information on Create React App before proceeding.
To spin up a new app using Create React App, run the following command in the terminal.
And start the app
This will open a new browser window with the application running at http://localhost:3000.
Build an Input
Inside the src/ directory, create a new file called regex-iban-number-input.js. Create a new React Component called IBANInput and for now, just render some text inside a <p> tag.
Next, open up App.js and delete all the boilerplate between the two <header>s. Import your new IBANInput component, and render that instead.
Check out your browser window at https://localhost:3000. You should now see something like this:

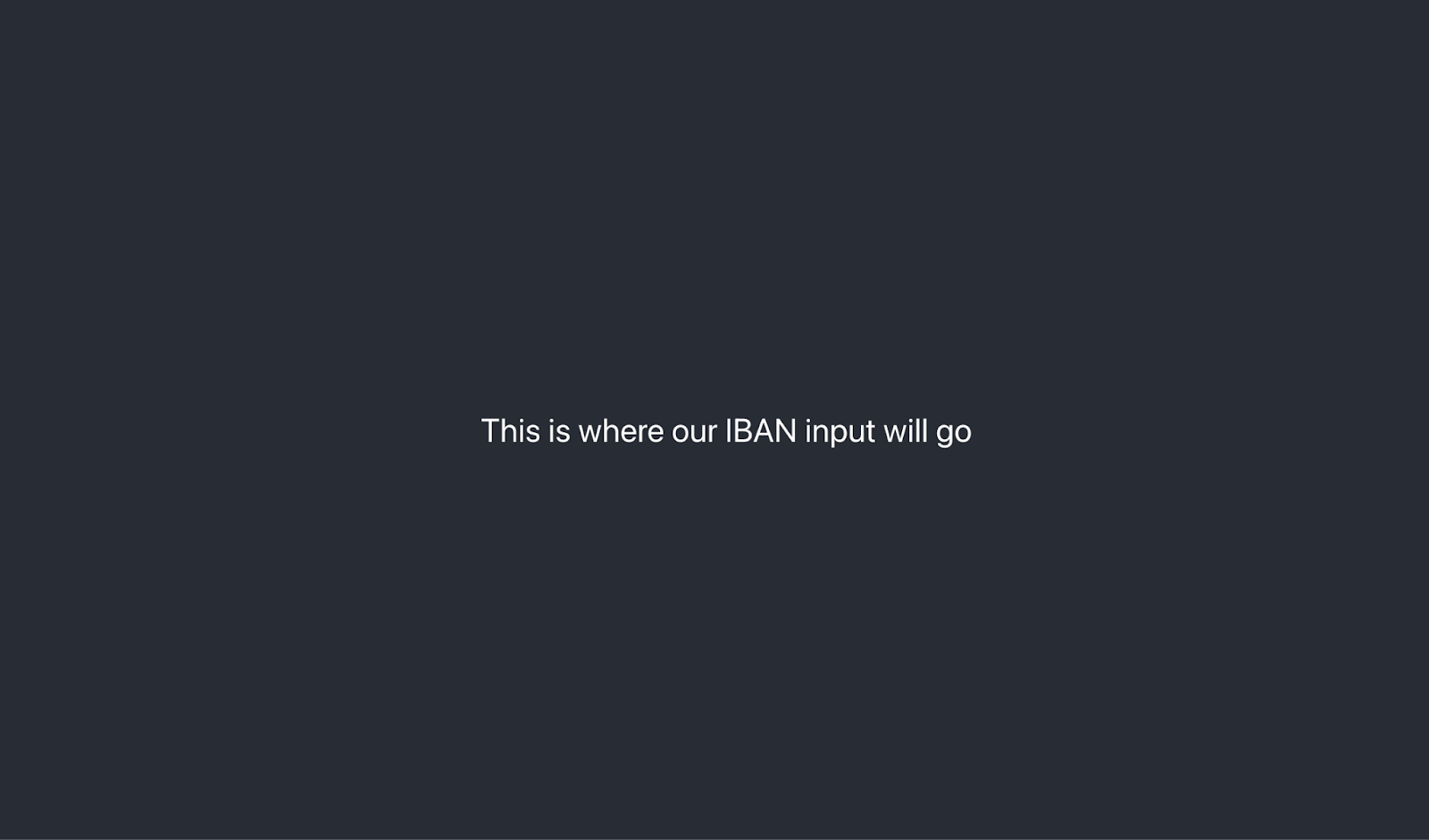
That’s all we need to scaffold out our app. Now let’s build the input.
Add a Text Input
Inside the IBANInput, import a basic React Text Input component. Create a value in state for the IBAN number using the useState hook. Create an onChange handler that will update the value of the IBAN number when the user edits the input.
Create a handleSubmitPayment method that will be called by the onSubmit prop on the <form>. For now, we’ll just output our submitted data to the console. In reality, this method would send our payment transfer request to our backend or a remote server.
Head back to https://localhost:3000 to see your new, basic IBAN input component rendered in the browser.

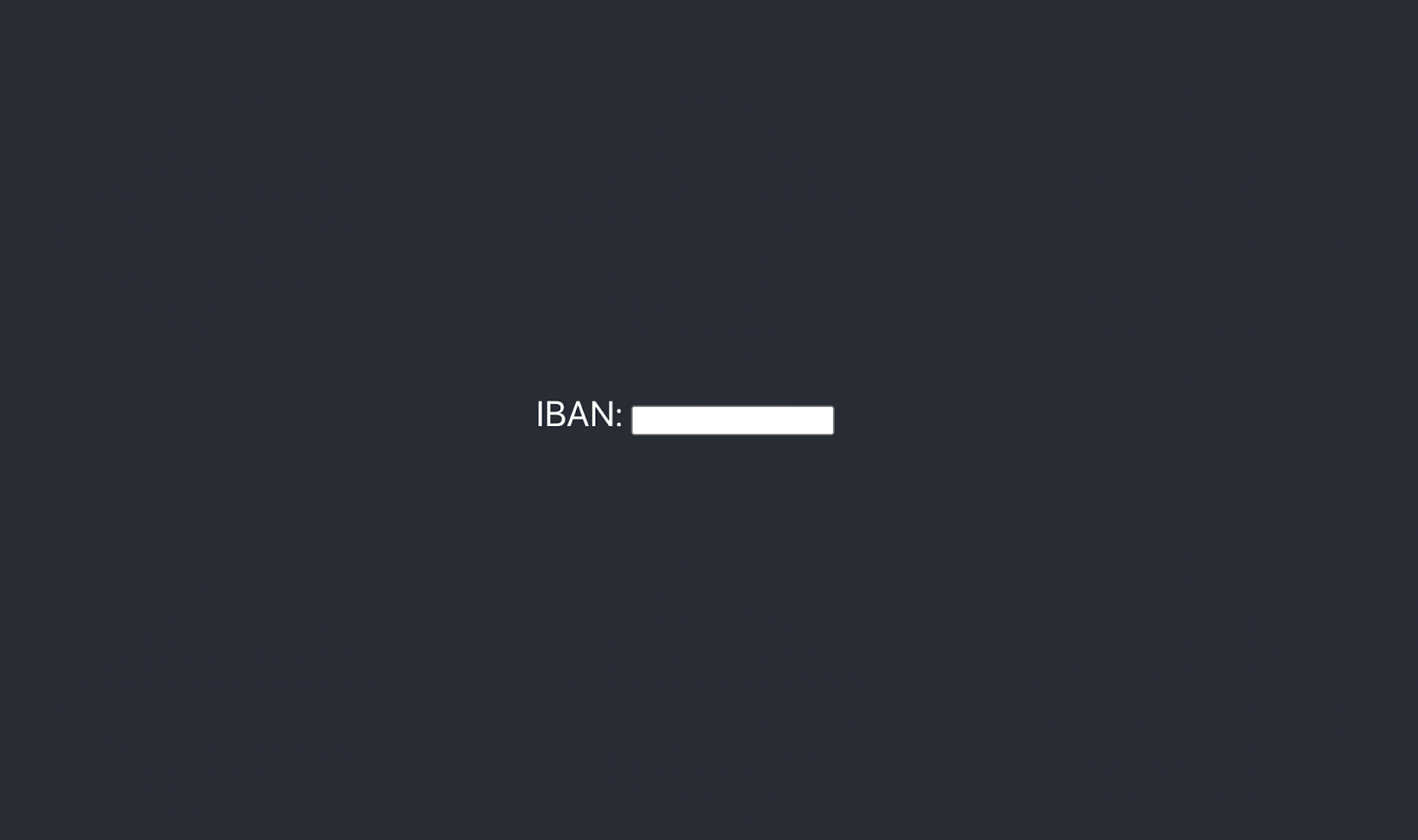
Time to add a validation step using AbstractAPI.
Add Validation Logic
The easiest way to add a validation step to a user form is to simply validate the input when the user hits submit. In the case of our app, we’ll take the string that the user entered into the input, send to the API for validation, and when we receive the response, we’ll then allow the payment request to go through only if the response from the API indicates that the number is valid.
Get Started With the API
The AbstractAPI endpoint is free to use to develop test applications. If you need to make more requests than the free tier will allow, there are several pricing options available. Let’s get started using the API.
Acquire an API Key
Navigate to the API’s Get Started page. Click “Get Started.”
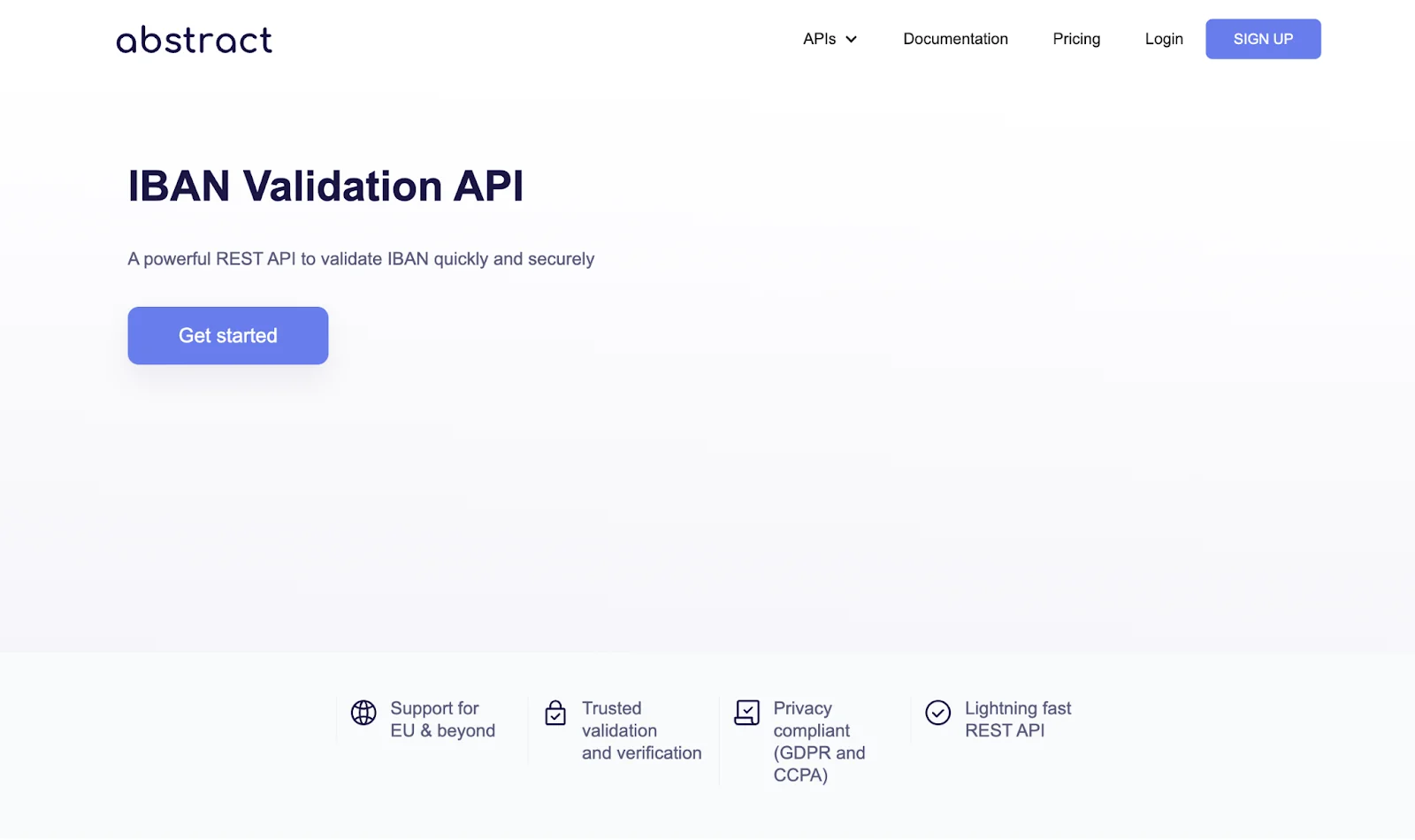
If you’ve never used AbstractAPI before, you’ll be asked to create a free account with your email and a password. If you have used Abstract before, you may need to log in.
Once you’ve logged in, you’ll land on the API’s homepage. You’ll see options for documentation, pricing, and support, and you’ll see your API key. All Abstract APIs have unique keys, so even if you’ve used the API before, this key will be new. Copy the key and the API’s endpoint URL, we will add them to variables in our app.
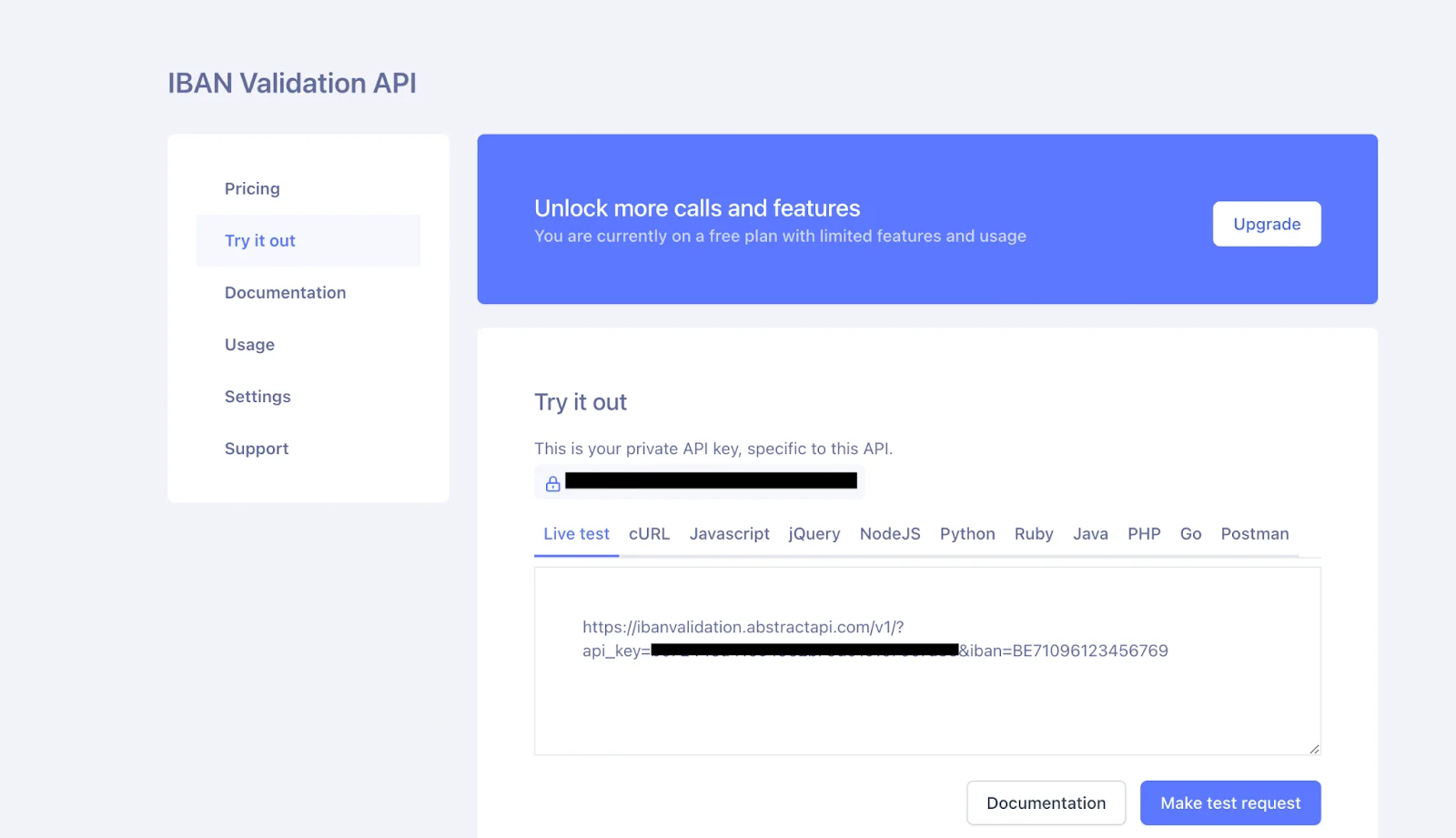
Use Your Key to Send a Request to the API
We’ll use the fetch global HTTP client to send a GET request to the AbstractAPI endpoint. Fetch is included in React by default
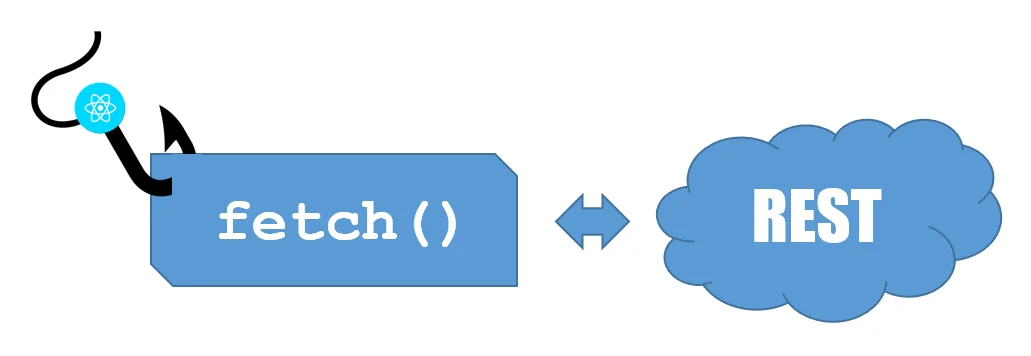
Create a method called validateIBAN. This method will send the IBAN string that was input by the user to the AbstractAPI endpoint, and will evaluate the API’s response, returning a boolean value. We use the API key and the base URL to construct the URL to which we’ll send the request. Then, we’ll append the IBAN string as a query parameter to the URL.
The iban value we append to the end of the string is pulled directly from state. We do not need to pass it as an argument to this method.
The response data will look something like this:
This is a very easy-to-parse response. All we need to do it pull out the is_valid field and return it as the result of our function.
Validate the IBAN Before Submitting Payment Request
Let’s add this step to our handleSubmitPayment function and use the result of the validation request to determine whether payment should be submitted.
Try entering a valid IBAN number into the input and hit “Submit.” You should see the expected output in the console. Same if you enter an invalid IBAN.
Put It All Together
Here’s the full code for our IBAN number input component.
Additional Information
IBAN Number Structure
An international bank account number consists of a two-letter country code, followed by a two-digit checksum that is calculated using the MOD97 formula, followed by a NIB—a 34-character sequence of digits that identifies the bank, a PSP reference number, basic bank account number, and two NIB check digits.

How IBAN Numbers are Validated
An IBAN (international bank account) number is primarily validated using the MOD97 algorithm. MOD stands for “modulus” and it is a mathematical operation. There are several other steps involved in validating IBANs, including validation of the length of the number and whether or not the country identified by the country code can support IBAN, but the MOD97 evaluation is the first and most important step.
If an IBAN number does not pass MOD97 validation, it can be assumed to be invalid.
Modulus
The modulus operation is the process of finding the remainder when one number is divided by another number. You will also see it referred to as “modulo.” They are the same thing.
As an example, when you divide 4 by 2, the remainder is 0. So 4 modulus 2 is 0. The modulus operator is typically represented by the ‘%’ symbol in programming. So to represent the above example, we could write
4 % 2 = 0.
MOD97
The MOD97 algorithm uses the modulus operator to find the remainder of the numeric value of an IBAN number when it is divided by 97. If the remainder of the operation is 1, then the IBAN number is assumed to be valid.
Steps
- First, the IBAN number is stripped of all non-alphanumeric characters (spaces, dashes, etc.)
- Next, the first four digits (the country identifier and checksum) are moved to the right-hand side.
- The alphabetic characters are converted to their numeric representations, using a standardized conversion method.
- Finally, the resulting number is divided by 97, and the remainder is found. If the remainder is 1, then the IBAN is valid.