This method leverages the PHP’s inbuilt function filter_var( ) to check for the valid email format to return a success (200 OK) or a failure (400 Bad Request).
To make the class methods accessible through an API, add this code outside the EmailValdiation class definition to create an instance of it that accepts an incoming API request.
Here is the complete code for defining the EmailValidation class, and its instance invocation.
Now, we are ready to test this code. You must run this PHP code as a web server by launching it from a command line terminal.
Make sure to run this command in the same directory where you have saved the email.php file. Now, the PHP web server is running and the URL is http://127.0.0.1:8000/email.php
If you launch the Postman API tool and fire up the API with a valid email, you should see an API response with the “Valid email” message.
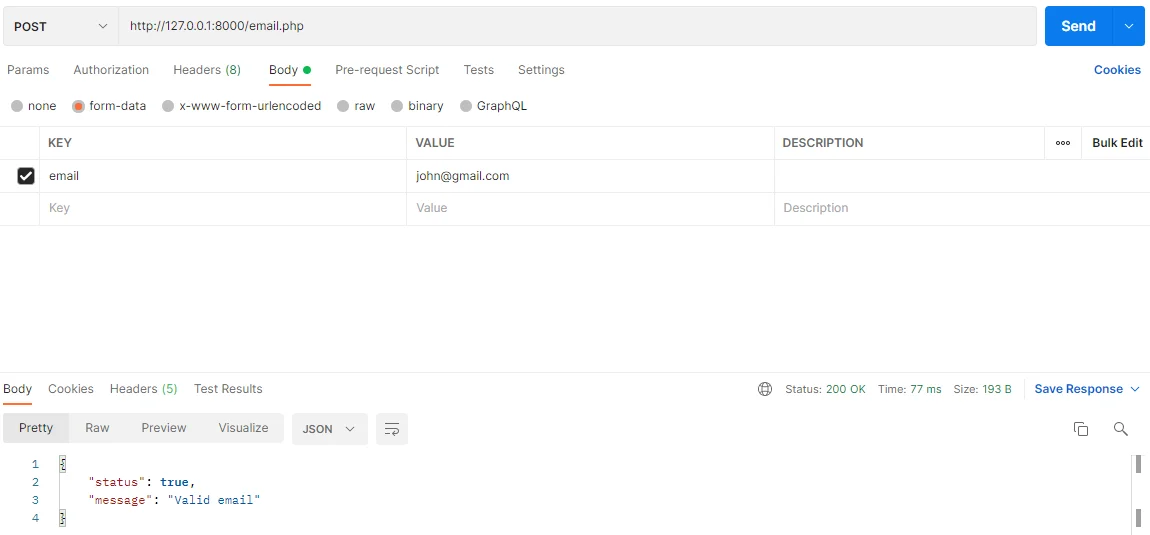
The filter_var( ) can also catch a wrongly formatted email address.
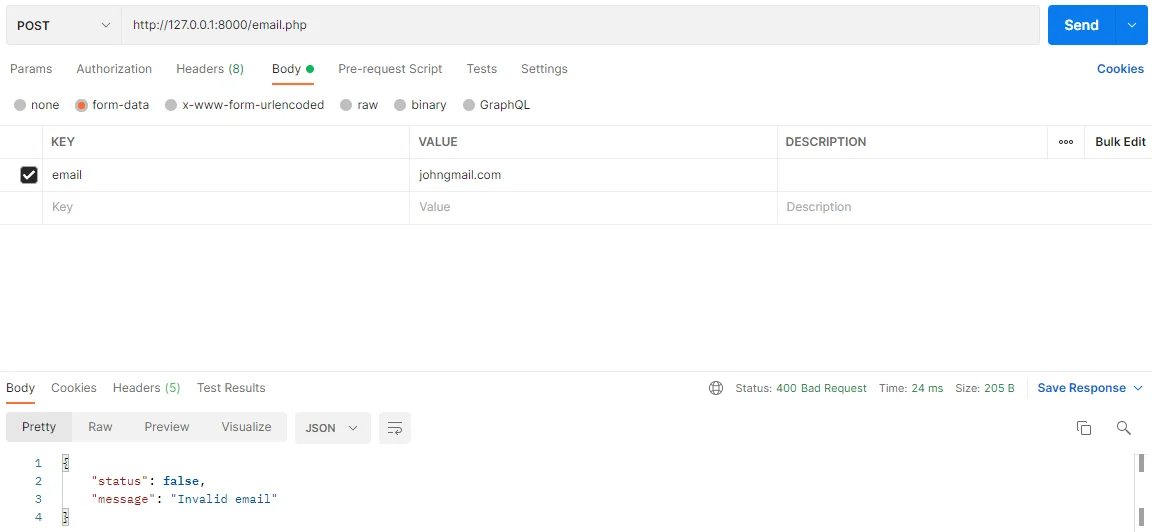
Validating Email Addresses with Regular Expressions in PHP
The in-built function approach can validate an email address based on the standard format. However, for checking custom email rules, the regular expression is the best choice.
PHP has in-built support for regular expressions through the preg_match( ) function. Let’s use it to build a matching pattern for an email address.
Default PHP Email Regex Validation
Let’s modify the EmailValidation class to add regular expression-based validation. Add a new private method withRegEx( ) to validate the email address with the preg_match( ) function.
Also, you must modify the last line of getRequest( ) method to call withRegex( ) instead of withPHPFiilter( ).
If you test the API, you should get the same behavior for email address validation as the in-built function in the above example.
Custom PHP Email Regex Validation
Regular expression validation takes the cake when dealing with custom rules for email IDs. For example, if you want to ensure that the submitted email address should not have an underscore ‘_’ character in the email ID, you can modify the regex pattern to omit that.
The existing regex pattern defined in the withRegex( ) method allows alphanumeric characters along with ‘_” , “.” and “-” characters. If you want to omit the underscore, then you must define a regex pattern like this.
Replace this pattern in the withRegex( ) method and save the file. Let’s test it out.
The API will now return a failure for an email address with an underscore character in the ID.

Similarly, you can define finre grained, custom regex rules to omit illegal characters from the email. That’s the power of regular expression. However, this kind of email address validation is also limited to the address format.
Validating Email Address with API
The PHP in-built and regex functions are great options for validating email addresses. But if your business really depends on the legitimacy of email addresses, this becomes mission critical. Under these circumstances, merely validating the email address format is not enough. You need to validate the email address to ensure that the email exists. Moreover, it should belong to the persona you are targeting, and its underlying domain and SMTP servers are also valid.
These advanced validations can only be done via an API service. Abstract API offers an Email Validation and Verification API to solve this problem. It goes beyond the simple addressing patterns to validate email based on the email’s domain and SMTP checks.
Check out the capabilities of this API by signing up for an Abstract account. You can access the API dashboard to make a test API call with any email address. Make sure to take note of your API key.
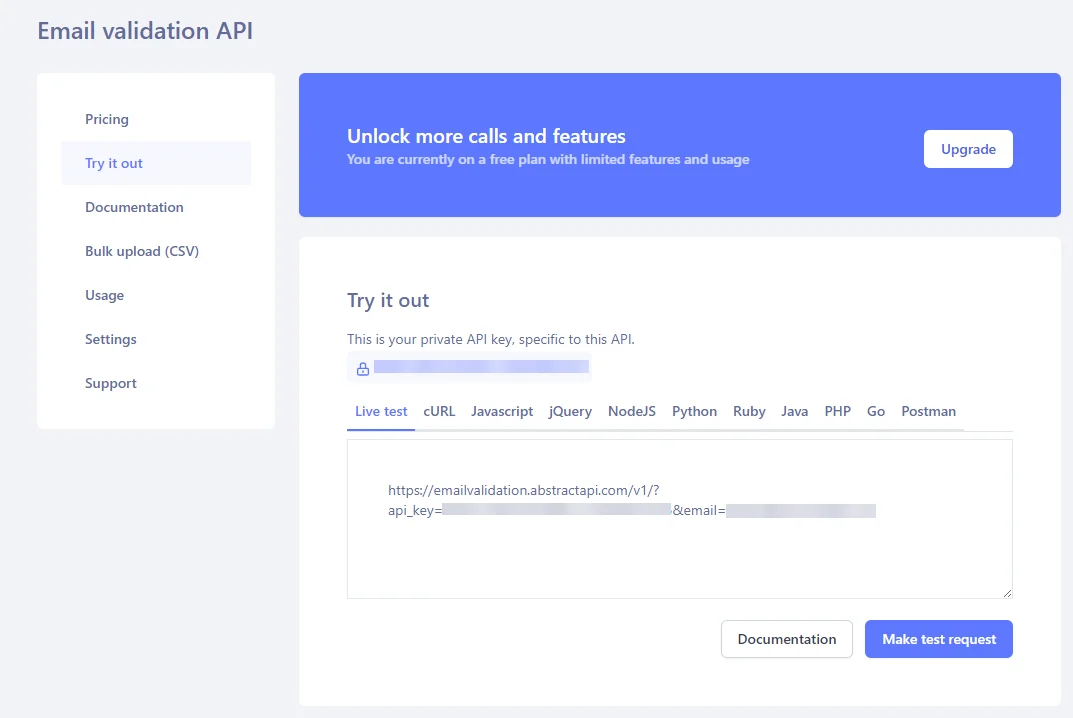
This API provides deeper insights about an email address, such as the deliverability of the email domain and the validity of the MX records, over and above the basic format validation checks. You can check the documentation on the API page. This API gives you 100 free API calls per month.
Integrating this API in any PHP code using the curl library is quite straightforward. Let’s add one more private method withAbstract( ) to the EmailValidation class.
This method calls the Abstract Email validation API and checks the response for
- Deliverability, to check if the email is deliverable or not
- Format, to check if the email address has the right format or not
- MX Record, to check if the SMTP domain configuration is email address exists or not
- Non-disposablity, to check if the email address is a permanent address or not.
If any of these checks fail, the email address is marked invalid. You can also see that the API response can now indicate additional error messages for each of these failures.
You need the API key to call the Abstract API from within this method. Add the API key as a public class property on the EmailValidation class.
Make sure to replace the value of $apiKey with the actual key allotted to you in your Abstract API account.
Make one more update in the getRequest( ) method to replace the call to withRegex( ) with withAbstract( ). Now you are ready to test the PHP-hosted API.
Let’s test with a fake email address.
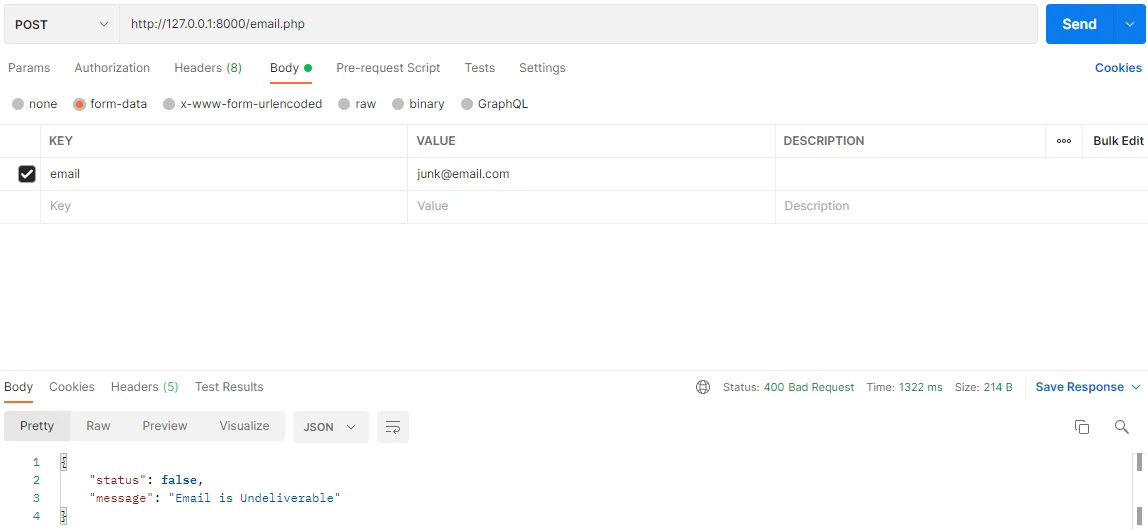
As you can see, the email address format is valid. Still, the email address does not point to a legitimate mail domain. Therefore, Abstract API will validate the email to indicate that it is undeliverable. The in-built PHP function and regular expression validation would never be able to catch such validation issues in email addresses.
You can combine the Abstract API with your custom regex function filters to build a foolproof PHP email validation logic. In this can, you can define another method that performs the regex validation of the email id and then lets the Abstract API validate the deliverability and other aspects of the email address.
We encourage you to extend the EmailValidation PHP class to add this new method for combining custom regex validation with Abstract API email validation to build your own rule for the valid email address. Give it a try, and you will realize how easy it is to build custom email validation logic in PHP without writing much code.
FAQ
How Do I Validate Email Addresses in PHP?
PHP provides a simple validation function for detecting valid email addresses. Using the in-built filter_var( ) function, you can validate any email address for the standard format checks, such as the presence of the email id, the ‘@’ symbol, and the domain part. This is a quick and easy approach to validating email addresses. However, it is ineffective in detecting fake email addresses that appear to be formatted correctly but are undeliverable. For such advanced validation, you can use the Abstract Email Validation API to detect fake email addresses based on database lookup and real-time SMTP checks on email domains.
What Validations Are Possible for Email using PHP Regular Expression?
The preg_match( ) is an in-built function in PHP, for performing regular expression matches. Email addresses have a standardized format. Therefore, regular expression matches can be used to validate the format of any email address. Apart from the standard norms for checking the email id, ‘@’ symbol, and the domain part of the email address, regular expressions can enforce custom rules for email ids that include or exclude certain characters. For example, it is possible to build a regular expression that invalidates any email address that contains an underscore ( ‘_’ ) character in the email id.
How To Check if an Email Address is Legitimate?
The sure-shot way of checking for valid email addresses is to send a test e-mail to those addresses. However, this may not be the most scalable and speedier approach. Also, this approach does not take into account email addresses that are genuine but are temporary or role-based mail aliases. You can leverage the Abstract Email validation API for foolproof validation of any email address. This API provides more profound insights about validating emails, from basic format checks to the MX records and deliverability status. This API helps you profile email addresses and filter out any invalid email addresses based on multiple criteria.