Development Environment for AJAX PHP Email Validation
This sample application uses a simple web page consisting of a form containing the email address field and a submit button.

Prerequisites
To replicate this application, you must have a working installation of the PHP runtime on your computer with version 8 or above. Check the official PHP download page for the PHP installation packages as per your operating system.
It would also help to have a top-level project directory to contain the source files for this sample application.
Frontend form for email submission with AJAX
The frontend web page for this sample application contains the web form, containing a single, default input type field, for submitting the email address. Here is the complete source code for the HTML web page.
Let’s understand the intricacies of this web page to understand how AJAX is implemented here.
This HTML file is a Bootstrap-based web page containing a form element with an <input> element. There is a submit button that has a click event associated with it. There is also an empty <div> element, with the id as “result”, above the form element for displaying the form submission result.
The AJAX functionality is implemented inside the click event. We have used the popular JQuery $.ajax( ) function. This function makes an HTTP POST request to the server and submits the form data. The HTTP response is handled in the anonymous function assigned to the success parameter of the $.ajax call. It updates the <div> element with the success message returned by the server. This function is always invoked when the server returns a 200 response, which indicates a successful HTTP response.
Save this file as index.html under the project directory.
Backend PHP Script for handling Email validation
Here is the backend PHP script for handling the server-side logic of email validation.
This PHP script is a simple implementation of the server-side response for AJAX calls. It performs a format validation on the email address using the filter_args( ) function. Based on the validation result, it returns a response, which is displayed on the web page.
Save this file as index.php under the project directory.
Testing the Email Validation Logic
To test this application, follow the steps below:
- Open a terminal window and change the directory to your project’s top-level directory.
- Launch the PHP development server by running the following command.
- Launch a browser tab and open the URL http://localhost:8000/index.html
- Test the form by submitting an invalid and valid email address.
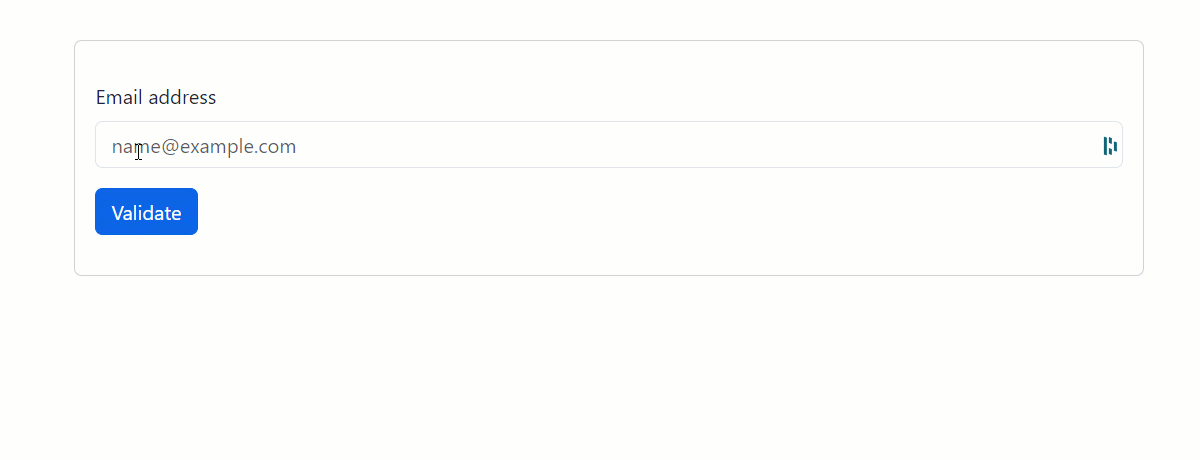
The JQuery AJAX is at play here. Whenever you submit the email address through the input field, an AJAX interaction happens between the client web page and the server PHP script to serialize the email address and check its format validity to return the validation status.
This way, you can use JavaScript to make asynchronous HTTP calls to the server and dynamically update any HTML div element without reloading the entire web page. And that’s precisely AJAX. The only difference here is the use of JSON as a data format instead of XML since JSON has gained preference over the years and has replaced XML. Therefore, some publications on web development now refer to AJAX as AJAJ.
Error Handling in AJAX
The AJAX code presented in the previous section is a bare-bones implementation. Unfortunately, many things can go wrong in a real-world AJAX application, which needs to be handled.
For every AJAX call, two primary errors must be handled:
- HTTP 4xx and 5xx errors: HTTP response codes returned from the server. These codes must be programmed in the PHP script to ensure the server returns an appropriate code to the client web page in case of specific error situations. It ensures consistent error handling and user experience.
- Network timeout: Since AJAX works over the Internet, any disconnection or delays in HTTP interaction between the client and server must be handled gracefully to inform the user about the issues.
Here are the modified versions of index.html and index.php with error handling.
You can see two modifications in the $.ajax( ) call. One is the error parameter which is assigned a function to handle errors. This function gets called when the server returns an HTTP response of 400 or 500. The second modification is styling the <div> element to change the text color for success and error messages. The $.ajax( ) call also adds a timeout of ten seconds and handles the timeout error to display an appropriate message.
In the PHP script, HTTP return codes have been added to handle 200, a success response for valid email address validation, and a 400 failure response for invalid email address validation.
You can replace the existing index.html and index.php files with these versions to do a quick test by performing a form submit for an invalid and valid email address.
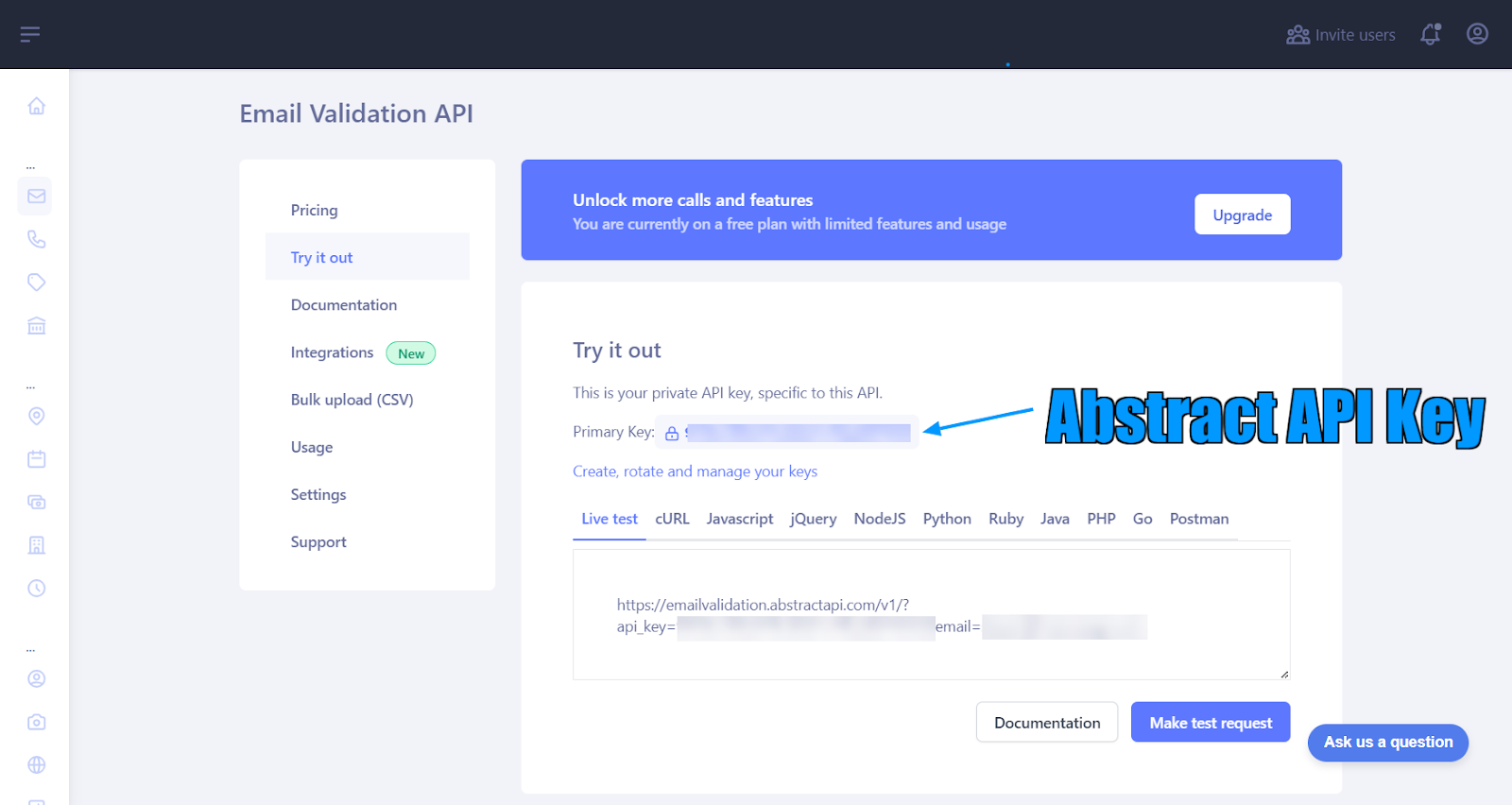
As you can see, the client-side AJAX calls can now differentiate between success and failure responses and update a color-coded text message on the web page.
To test the timeout scenario, you can modify the index.php AJAX handling code to add a sleep function before the if block to simulate a server delay.
This will lead to a twelve-minute delay in server response, by which time the $.ajax call will return with the timeout error since we have set the timeout parameter to ten minutes. Upon timeout, the webpage will be updated with a message indicating the same.
A More Reliable Email Validation using AbstractAPI
The email address validation technique used until now relies on a native PHP approach to check the address format. This approach has a severe limitation in that it cannot assert the deliverability of the email address. If you plan to use the email address submitted by the user for future correspondence, it is essential to validate the deliverability of the email address.
Fortunately, there is an easy way out. Using the Abstract Email Validation and Verification API, you can get a detailed report on the legitimacy of any email address, including its deliverability.
Here is another modified version of index.php that uses the Abstract API endpoint.
This script uses the PHP cURL library to make an API call to the Abstract Email Validation and Verification API. You can sign up for a free Abstract account and get access to your API key under the dashboard. After replacing the existing index.php with this code, replace the placeholder <YOUR_ABSTRACT_APIKEY> with the API key allotted to you, before saving the file.

You can also notice that the PHP script now performs email validation at two levels. At the first level, it does a basic format check and returns a 400 response in case of an invalid format. At the second level, the Abstract API is invoked to return a 200 or 406 error corresponding to the deliverability status. This way, you can return a more granular set of error codes in the AJAX call based on the server’s implementation.
Now, the AJAX call will be able to differentiate between a valid email address that is deliverable from the one that is not. Here is the accompanying modified index.html for handling 200 and 406 HTTP response codes. An additional orange color is added to display the email address validation status, which is not deliverable.
A quick test with valid and invalid email addresses displays all three results for:
- An invalid email address.
- A valid email address.
- A valid email address that is not deliverable.

With this, your AJAX implementation is complete, and you can perform email address validation considering all the common failure scenarios. You can replicate this code to implement a more complex form submission with additional data.
Note: In this tutorial, specific HTTP response codes like 406 have been used based on the application logic’s discretion. This is done to explain how to handle success and failure scenarios in AJAX calls. In a real-world scenario, many such response codes have specific, pre-defined meanings and should not be used to handle application-level errors. In such cases, a 200 success response code with additional data fields must be used to indicate errors.
FAQ
How can I validate an email address using AJAX and PHP?
Validating an email address using AJAX (Asynchronous JavaScript and XML) and PHP involves creating a communication flow between the front end (using JavaScript and AJAX) and the back end (using PHP for server-side processing). For the front end, you can use the popular JQuery library, which offers the $.ajax( ) function to perform AJAX calls. This call triggers an HTTP request with the payload containing the email address. You can implement a basic email validation check at the back end using the PHP filter_var( ) function. You can also leverage the Abstract Email Validation and Verification API for more advanced validation.
How to debug common issues in AJAX PHP email validation?
Most of the issues in AJAX calls are due to network timeouts or HTTP errors. To debug the problems in AJAX, you must handle the error block in the JQuery $.ajax( ) call, which contains a lot of information about the HTTP response codes and error strings. Most importantly, you must handle timeout and HTTP response codes in the 4xx and 5xx categories. Timeout can happen due to network delay and server unavailability. 4xx codes indicate a client-side error, and 5xx codes indicate a server-side error. For conveying application-related errors, you can use the 4xx error codes or a 200 success response with the additional field in the JSON response payload containing error information.
What are the prerequisites for implementing email validation with AJAX and PHP?
To implement AJAX, you need a JavaScript API to perform AJAX calls from the webpage. You can use native browser API like the fetch( ) function. To avoid browser-to-browser inconsistencies, it is best to use a common library like JQuery, which offers a set of AJAX methods like $.ajax( ), $.post( ), and $.get( ) to perform AJAX calls in a browser-agnostic way. If your web application is built using a framework such as Angular or React, you get specific APIs available in those frameworks for performing AJAx calls. If you are using vanilla PHP on the server side, you can use the built-in PHP super global variables like $_SERVER, $_POST, and $_GET to check for AJAX requests coming from the client, extract and process the AJAX payload, and return an appropriate response with an HTTP response code. In the case of using a PHP framework like Laravel or CakePHP, you have access to the framework-specific directives for handling AJAX calls instead of reading the raw data directly from the super global variables.