Get Started With an Angular App
The first thing we'll do is spin up a basic Angular app using the Angular CLI. This tutorial won't go in-depth into how to configure your Angular application, or how to work with Angular. There is more information about how to do this in the Angular docs.
Spin Up A New Angular Application
Use the CLI to create a new Angular application. The CLI will guide you through the setup process and will take a minute to install dependencies.
Once the app is created, cd into the root folder and start up the server.
By default, the app runs on port 4200, so head to https://localhost:4200 to see it in action.
Use Reactive Form
Angular provides a handy module for working with forms called ReactiveFormsModule. To use it, import it into your NgModule imports array from the /forms module.
Now, create a form component. This is where all the display logic for your form will live.
Import the FormBuilder class and inject it into the constructor:
Use FormBuilder to generate all the display logic for your form. FormBuilder can even handle some basic email validation for you.
As you can see, we're making the email address field required and letting the FormBuilder know that it should expect an email address in that input. If the syntax for the address is incorrect, the form will render an error.
Make the HTML Template
To render the actual form, we need some HTML. Paste the following code into the email-form.component.html file:
The formControlName, and name attributes tell Angular that these HTML elements should be hooked up to the FormControl component you just built.
Create an Email Service
The actual logic of sending emails will be handled by a service component. Angular uses the word service to refer to any module that handles data level logic and behind-the-scenes stuff like making HTTP requests and processing data.
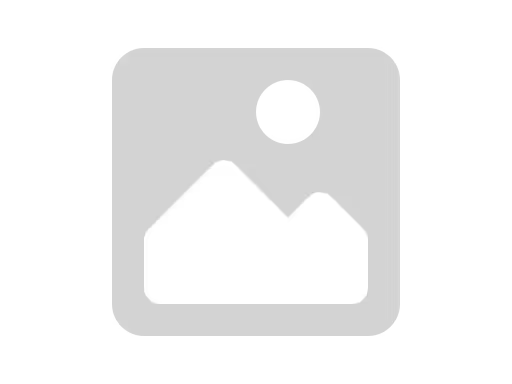
Generate the email service file using the following command:
Into that file, import the HttpClientModule and add it to the providers array.
Now, create the function that will send the email request to our third-party service.
We've left the url variable blank for now. That will be filled in once we set up our Mailthis alias and receive a URL to post our request to.
Send Emails from an Angular App Using MailThis
Before sending an email in an Angular application, it's essential to validate the input to ensure users provide a properly formatted email address. Implementing Angular email validation helps prevent invalid or malformed email entries, reducing errors and improving the overall user experience. This step ensures that only valid emails are processed before being sent through your Angular app.
The easiest way to send client-side emails is to use a third-party email service library like Mailthis. Mailthis allows you to set up a URL that redirects incoming email requests to an email address of your choosing. It's very easy to set up.
Create an Alias
In order to use Mailto, you'll need to create an alias. Head over to the website to sign up and create one. It's free.

Once you've created your alias, you'll receive an email with a confirmation link. Just click the link to confirm your alias and you're done.
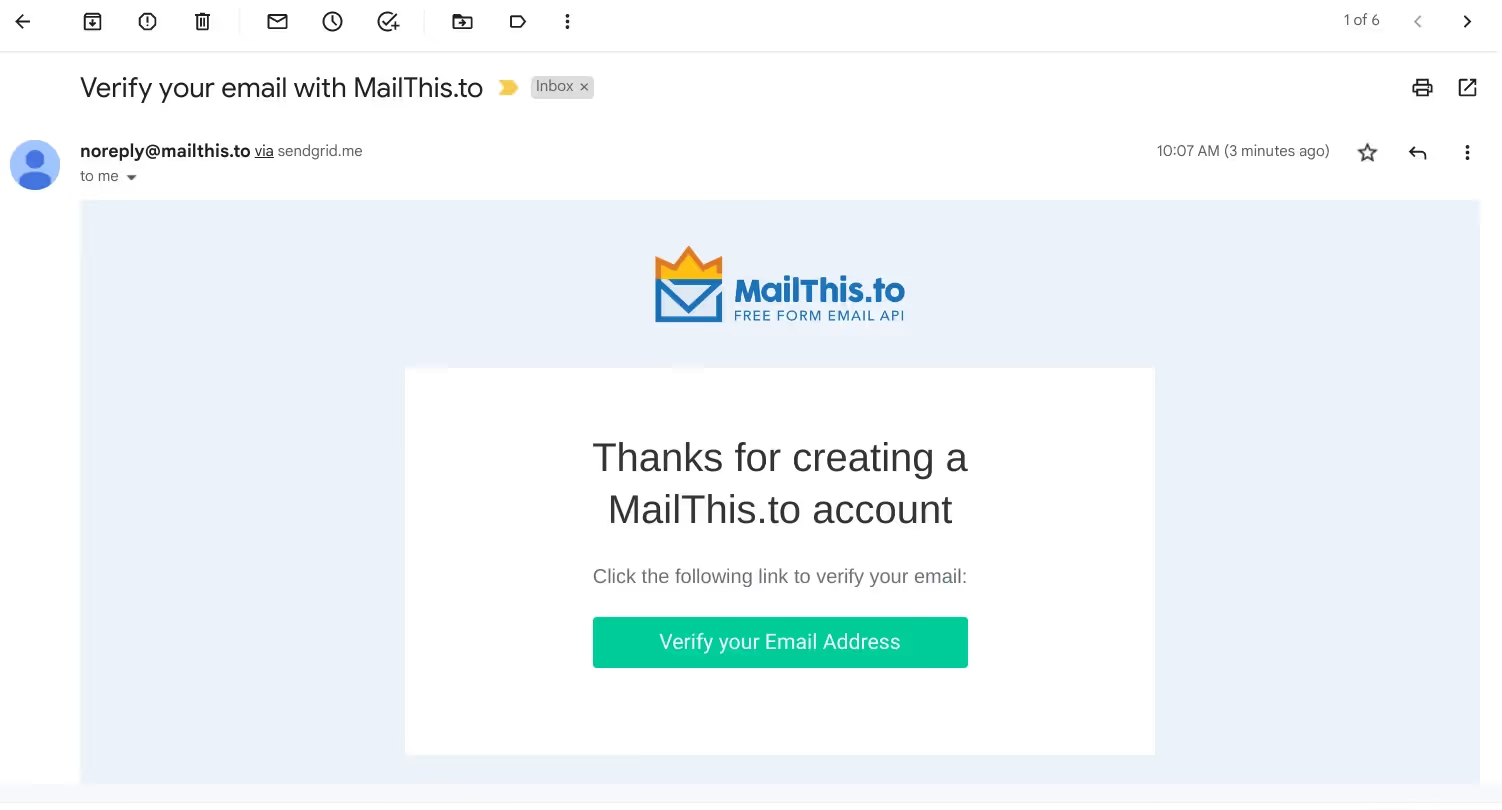
Mailthis will create a unique URL that you can send a simple POST request to with the information you want to send in your email.
Add the URL you received from Mailthis to the url variable in your service.
Submit the Email
Finally, in our email-form.component.ts file, create a submitEmail function that sends our request to Mailthis.
That's it! We've successfully set up a client-side email API using Mailthis and Angular.
Conclusion
It's a bit trickier to send emails from the front end than it is to send regular HTTP requests. Email requires a special set of security protocols to prevent spam and bad actors from getting into people's mailboxes. Fortunately, there are tons of free services out there like Mailthis that can help you send emails from the front end.
FAQs
Can we send emails using Angular?
It is possible to send emails from Angular without writing any server-side code, using a free service like Mailthis, a free email API, or smtpJS, a client-side Javascript library. You will need to sign up with the service or download the library in order to include it in your application. Follow the documentation on the company's website for complete instructions on how to get started.
How to send emails without a backend in Angular?
Sending emails without writing your own backend is possible in Angular if you use a third-party SMTP service. These platforms act as a middleman between your application and your email client, performing all the necessary SMTP checks and following proper protocol for sending emails. It is possible to write your own Node server that will do this, but it can be complicated and difficult to scale.
How do I send a front-end email?
The mailto link is the easiest way to send an email from a front-end Javascript app, however it is not recommended. To use the mailto link, put the email address you're sending to after mailto: as part of the string. When the user clicks on the anchor tag, mailto opens the user's preferred email client and populates it with the email address.
A better way to send front-end email is to use a platform like SMTPJS or Mailthis.