Getting Started With the Abstract API Resizing Images Endpoint
Abstract API's Free Image Resizing API allows you to make a POST request to an authenticated endpoint, with the URL for an image to be resized included in the request.
Abstract API returns a URL to the resized image. The image is resized according to the specific dimensions and parameters you included as options in the POST request.
Acquire an API Key
Navigate to the Images API home page and click the “Get Started” button. If you’ve never used AbstractAPI before, you’ll be asked to input your email and create a password. If you already have an Abstract API account, you may need to log in.
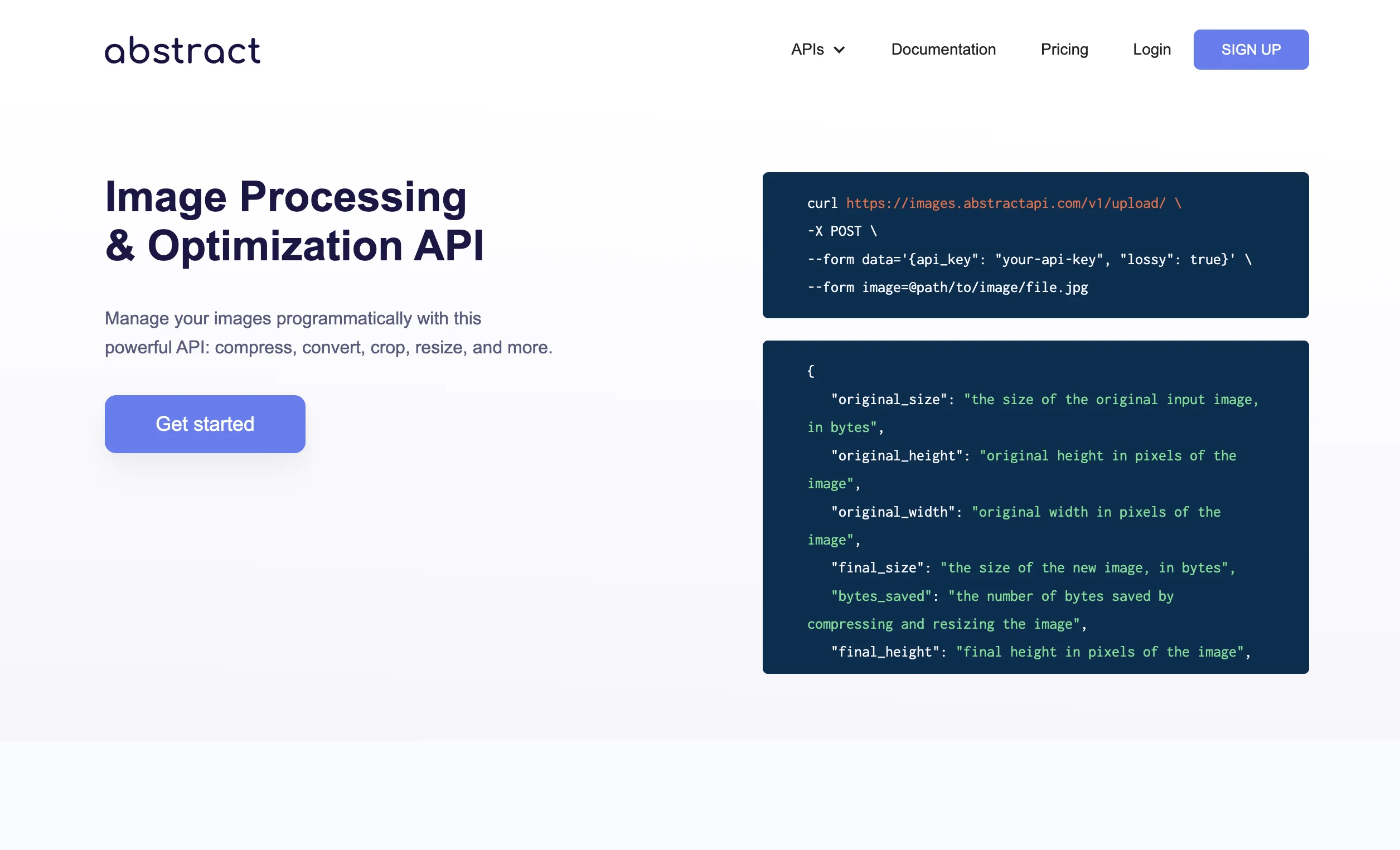
Once you’ve created an account or logged in, you’ll land on the API dashboard.

Every AbstractAPI API requires a different API key, so even if you have an existing API key from a different AbstractAPI API, the key for this API will be unique.
Making an API Request Using Node.js
In this tutorial, we’ll use the API’s URL endpoint, rather than the direct multipart/form-data upload endpoint. This endpoint takes the URL to a hosted image object on the web. We recommend hosting your images through AWS.
In this tutorial, we'll use a test URL for an already-hosted image:
Spin Up a Simple Node.js App
As a reminder, if you don't already have a Node app running on your computer, you can refer to these tutorials to get up and running:
- Check out this tutorial for help installing Node and creating a basic project folder.
- Check out this one to learn how to create a basic web server using vanilla Node or Express.
Install an HTTP Client
If you don’t already have an HTTP client installed in your app, we’ll quickly install the Axios client using yarn. Run the following command from the root of your app:
Create a Route to Handle Image Resize Requests
Create a file called image-processing.js.
This is where you’ll handle all HTTP requests and responses to and from the API.
Create a route in your index.js file to handle requests from the main app to the image processor and scaffold out the response.
Start the app using yarn start and go to localhost:3000/resize-image in your browser. You will see the words “Image Resized!” in the browser window. You will also see the request object printed in the console.
Great! Our app is running and the image processing route is hooked up.
Make a Request to the API
Add the following Javascript code to the image-processing.js file
Here, we've imported the Axios HTTP client, which we will use to send our request to the API, and assigned the Image API URL to a variable for clarity and ease of use. In the apiKey variable, we've put a placeholder string. You should replace this with your Abstract API key.
Inside the handler, we use Axios to send a POST request to the API, passing a JSON options object with our API Key and the photo URL.
Specify Parameters for the New Image
In order to tell the API how we want the original image source to be resized, we need to include the resizing logic. That is, we need to let the API know what should be the width and height of the resized version.
We can do that in just a few lines.
Add a resize field to the object and provide some specifications for how to change the image. The image we're sending is a landscape image, so we just tell it what the new width should be. The API will handle the aspect ratio (meaning the height will be automatically resized to maintain the correct dimensions.)
There are other options you can specify for the optimization, including lossy and quality. These will tell the API how to handle file size and image quality. We have not set the lossy field, so it will be false by default. This means no compression or loss of image quality will happen.
For a full list of parameter options, refer to the Image API documentation.
Handle the Response
The resizeImage code sends the request to the Image API, so now we need to handle the response from the API. We can then call the handler from the /resize-image route we created earlier in index.js.
We call the resizeImage function that we wrote inside our image-processor module. We create a handler called handleResizeImage, and pass in a source URL for resizing. You can pass the URL to the handler through the browser as a query string like so:
The sendResizeImageRequest function is an async function that handles the promise returned from our API request.
Use the Image Data
The /resize-image route takes an image URl as a parameter, sends that URL to the API for resizing, and returns the API response object as a stringified JSON object. If you run this in your browser, you should see the response object printed out on the page.
The new image is hosted in an Amazon S3 bucket. The API also returns information about the original image that was sent and the size, height, and width of the processed image.
To use the response, access the fields you want through dot notation. To display the resized image on the front end, use the URL string to populate a canvas or other image element or Javascript Image Object.
Conclusion
In this tutorial, we learned how to use the Abstract API Free Image Resizing API and Node.js to resize images. The image to be resized was already hosted at an S3 URL. The resized URL can now be used in a canvas element, or in some other Javascript element.
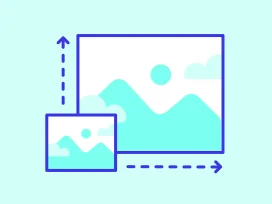
FAQs
How do I resize an image in code?
These days, the simplest way to resize an image in code is to send the image to an external library or API for processing. It is possible to create your own image processor, but it is time-consuming and difficult. Rather than spending the time to do this, you could host the image in an S3 bucket and then use either serverless AWS image resizing services or a third-party image processing API like AbstractAPI to resize the image.
How Do I Resize an Image in Node.js?
Node includes a library called Jimp, which can be used to resize images directly in Node. Jimp reads the image object into memory from a URL. You can then call ImageObject.resize() and pass width and height parameters. Pipe the output using the ImageObject.write() function, which allows you to write the new image to a file.
How Do I Resize an Image Using CSS?
There are a few ways to resize an image in CSS. The first is to set the width and height parameters on the class to a specified width in pixels. You can also set the width and height to a percentage, in which case the image will take up that much space in its parent container.
Finally, you can set the width or height to auto and set the max-width or max-height to 100% and resize the parent container. This will make the image take up 100% of the width or height of its parent, while maintaining the proper aspect ratio.