Understanding Image Cropping
Cropping images is a common process in image processing. It involves removing unwanted areas or outer areas from an image. It usually changes the size and shape of the original image and focuses the eye on the most relevant portion of the photo or illustration.

There are many different ways to crop images: using a piece of image cropping software, using an API, or using intelligent image processing.
Image Cropping using OpenCV
OpenCV (also called Open Source Computer Vision library) is a library with advanced computer vision capabilities that can be used to crop an image in Python. OpenCV can also be used with C++ and Java.
Get Started
First, you'll need to install OpenCV. This can be a little complicated, so we highly recommend checking out the documentation at PyPi and the OpenCV documentation first. There are a few things to keep in mind when installing OpenCV:
- If you have another version of OpenCV installed (i.e. one not installed via Pip - for example, a CV2 module in the root of Python's site-packages), remove it before you install another version to avoid conflicts.
- Ensure your pip version is up-to-date using pip install --upgrade pip. You can check what version of pip you are running version with pip -V. The minimum supported version is 19.3.
- Make sure you select the correct package for your environment. There are four different packages on the PyPi website and you should only choose one. Never install multiple packages in the same environment
Once you have installed OpenCV, you can import it where needed using the following:
Read an Image Using OpenCV
OpenCv exports three main functions for working with images:
- imread() reads an image
- imshow() displays an image
- imwrite() writes an image to a file
There is no method explicitly for cropping images: rather, NumPy array slicing is used to specify the area of pixels you want in the cropped image. When you read an image, it gets stored in a 2D array. Specify the height and width (in pixels) of the area to be cropped.
We'll test this out using the following image:
https://s3.amazonaws.com/static.abstractapi.com/test-images/dog.jpg

Because this image is hosted at a URL, we'll need to use a separate library called UrlLib to open the image from the URL. Then, we'll use NumPy to turn the image into a NumPy array before decoding the array with OpenCV:
Note that instead of using the standard imread method, we used imdecode. We can now use imshow to view the image in a window.
Crop an Image Using OpenCV
Now that we have the image, it's very easy to manipulate the NumPy array to crop image dimensions. First, we can check the original image dimensions using shape:
Next, select the area to be cropped and perform the cropping operation by slicing the array:
Image Cropping using Pillow
Another library that is commonly used to crop images in Python is Pillow. Pillow is a fork of PIL (Python Image Library) and provides all types of image processing functions: cropping, color manipulation, resizing, histograms, etc.
Get Started
To get started with Pillow, we suggest first reading the docs. Once you're familiar with the library, you can install Pillow using Pip. Be aware that Pillow and PIL cannot exist within the same environment, so if you already have PIL installed, remove it first.
Cropping Images With Pillow
We'll use Pillow to crop the same image we looked at before. Again, because this image is hosted at a URL, we'll need to use UrlLib to access the image first. Since Pillow operates on image files and not NumPy arrays, this process is a little easier. Simply retrieve the image using UrlLib and save it to a file:
Now that we have the image object loaded into Pillow, we can use the crop method that Pillow exports to crop the image to some specified dimensions. We'll decide those dimensions by first examining the dimensions of the original image:
Here, we specified that the image should be cropped in the middle, with 50 pixels removed from the top, right, bottom, and left of the image.
Image Cropping using AbstractAPI
AbstractAPI's Image Processing and Optimization API provides a free, easily accessible REST endpoint for cropping and resizing images. There are two methods available: multipart form data for uploading full image files, and the option to send a URL to a hosted image in a JSON object.
Let's look at cropping an image from a URL. We'll use the same test URL as before.
Get Started
AbstractAPI's free tier allows up to 100MB of uploads per month at one request per minute. Sign up with an email and password to get an API key. Once you do that, it's free for life. Even if you've used other AbstractAPI endpoints before, you need a unique API key for each endpoint.
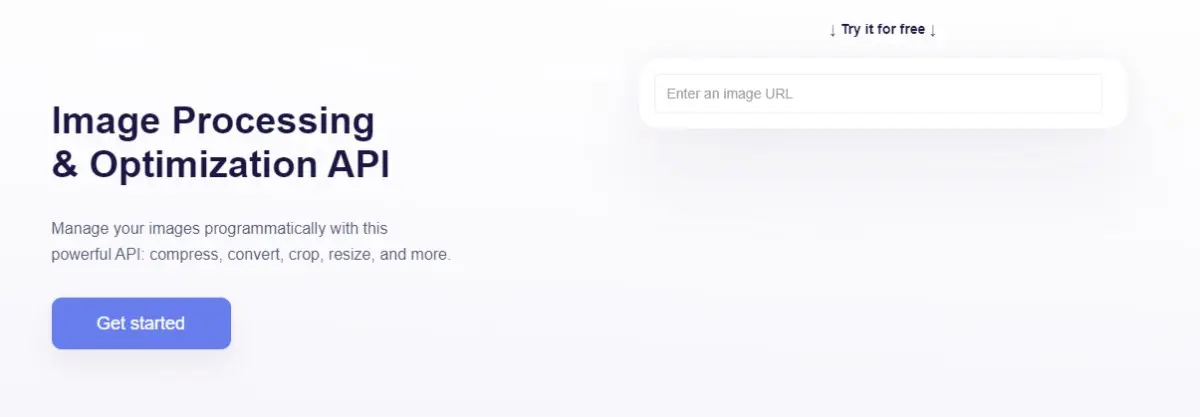
Send a POST request to the endpoint with a data object containing the url to the image you want to crop, your api_key, and resize options. To crop, specify that in the resize object.
The crop strategy will crop the image in the center by default and will use the width and height specifications provided to determine the size of the area to crop. Check the API documentation for more cropping options.
The response you get back will look something like this:
Conclusion
In this article, we looked at three different methods for cropping Python images: using OpenCV, using Pillow, and using AbstractAPI. All three methods can be used to crop images for all sorts of applications, including for computer vision applications.
The current use cases for image cropping in computer vision and machine learning include selecting relevant areas of photos to use to train AI. Image cropping is also used to automatically crop images such as user thumbnails quickly and intelligently.