How Email Validation is Done
The first step in email validation is to check if the address matches a valid email ID format.
- Valid Email Address Syntax Check
We use Regex (regular expressions) to check the format of an email address to make sure it matches the allowed format in the specifications (RFC 5321 and RFC 5322).
A valid email address has the form localpart@domain. Here:
- The localpart can have 64 characters - The specifications allow a wide range of characters including letters, numbers and many special characters. However, most mail service implementations support a smaller restricted set of characters.
- We will see various methods of email validation using jQuery for these different supported characters to choose how strict we want to be with our email validation.
- The domain name part has much tighter guidelines - it has to match the pattern for a valid hostname. A valid hostname comprises several 63-character blocks separated by dots. Each block can only have English letters, numbers, and the hyphen (-) character in it. We refer to this as the LDH (Letters, digits, and hyphen) pattern.
We start with the simplest method to validate email address syntax and build up to more robust and complex solutions.
Email Validation Using jQuery - Simple Method
The rules of email ID syntax vary from one mail service provider to another and can get pretty complex. So, we can be clever and start with a simple hack to clean our mailing list.
The logic for this method is straightforward - we only match with a regex to check if the input email ID has an "@" character in it. Here is the code:
Here is the related markup file.
Here is the associated email_validation.css code.
Here is the demo output of this solution.

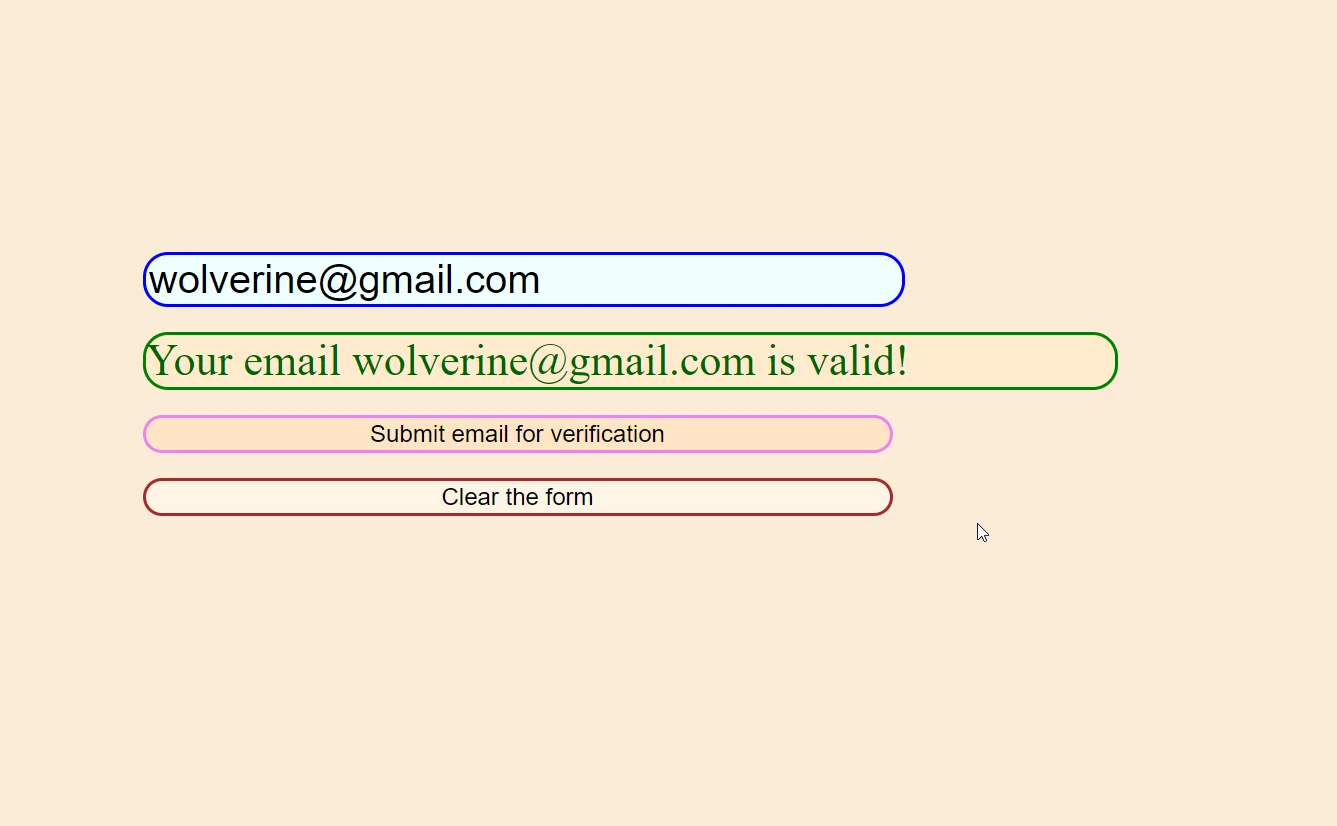
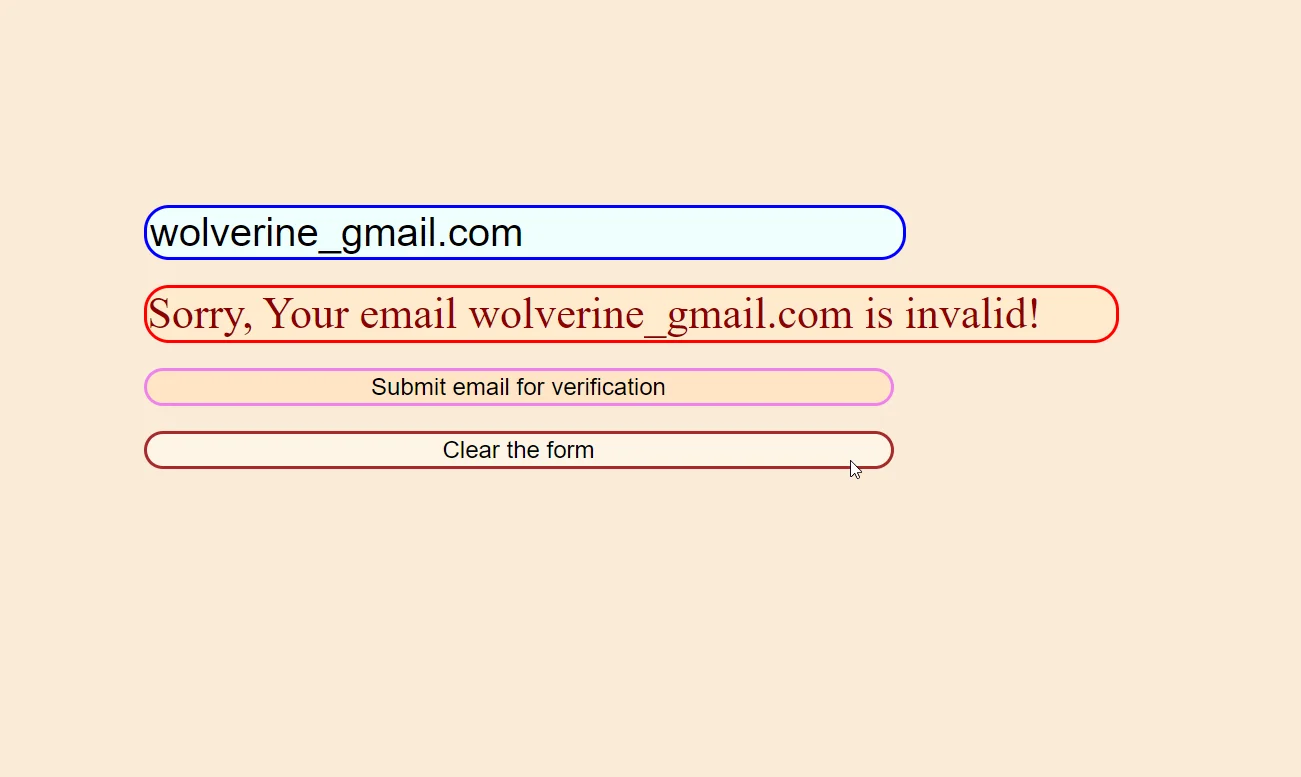
Let's break down the code to see what's going on here.
First up, we prompt the user to input the email they want to validate. Below that, we have a submit button to run the email validation, and a clear button to reset everything. Last, we display the result of the email validation check using jQuery in a paragraph (<p>) element that we initially hide.
In our email_validation_simple.js file, we attach an event handler to the submit button on the click event.
We extract out the user’s input email value and save it in the email_val variable using the .val() method on the input element.
We then run a function by passing in the email_val and regexSimpleEmail arguments with the logic for the email check (more on that a little later). If the user’s input email is valid and the function returns a true value, we display the results paragraph (<p>) element block with a confirmation message that the email is valid. We also add an isemail class to style the output in green and color-code it as a valid email confirmation message.
If the regexSimpleEmail functions returns a false value, we display an invalid email output message. Also, we remove the class we added earlier to color-code it in red as a negative result.
But, as we said, this is a very basic email validation filter for a quick cleaning of your mailing list. So, it does not remove some bad email addresses from your list. A few typical such use cases are:

The above email address is not valid as the domain name part does not have a Top-Level Domain (TLD) at the end (TLD's are the ending labels we all know, like .com, .au, or .org). But, our simple email check cannot filter it out.

The above email ID has an incorrect local name part (before the @ sign) as it has many special characters that most email implementations do not support. But, our simple email filter does not catch these.
So, we need to do better and build a more robust function.
Email Validation Using jQuery - Robust Method
Most email service providers support the following formats of valid emails.
The Local Part :
- May have all the letters of the Latin alphabet in both uppercase and lowercase i.e A-Z and a-z.
- May have any digit from 0-9.
- May have these special symbols : -, ., +, and _
- May have any of the above repeated any number of times.
The Domain Name Part:
- May have letters, both uppercase or lowercase i.e. A-Z and a-z
- May have digits from 0-9
- May have the dash character (-).
- It may have 4 "labels" that combine characters from the above three sets.
- The last "label" is special - we call it the Top Level Domain (TLD). It may only have 2 to 4 symbols of the supported character sets.
We devise a regex to match the above pattern and embed it in our code. Here is the JavaScript file for this jQuery validation method. (We use the same HTML and CSS files as before.)
Let us break down the regex in this code.
^([a-zA-Z0-9) matches for letters and digits. The ^ sign upfront means the email ID has to begin with this pattern.
We then also match for the special characters allowed in the local part. So, we add -/./+/_ to our regex. Here, we use the backslash (/) before these symbols to escape their usual meaning inside a regex (these carry special meanings inside a regex). So, now the code treats them just like normal dots, dashes, pluses, etc.
We then put a trailing + sign. This means the local part can repeat the above characters many times.
We then put the @ sign. We then add the pattern for a valid "label" inside the domain part of the email ID.
We then have a dot to separate the different labels. The trailing + sign allows us to repeat this pattern multiple times.
Last, we write the regex part for the TLD. It has the same character set as any other label, but we add {2,4} at the end to ensure that it is only between 2 and 4 characters long.
Let us look at the output for different input emails.
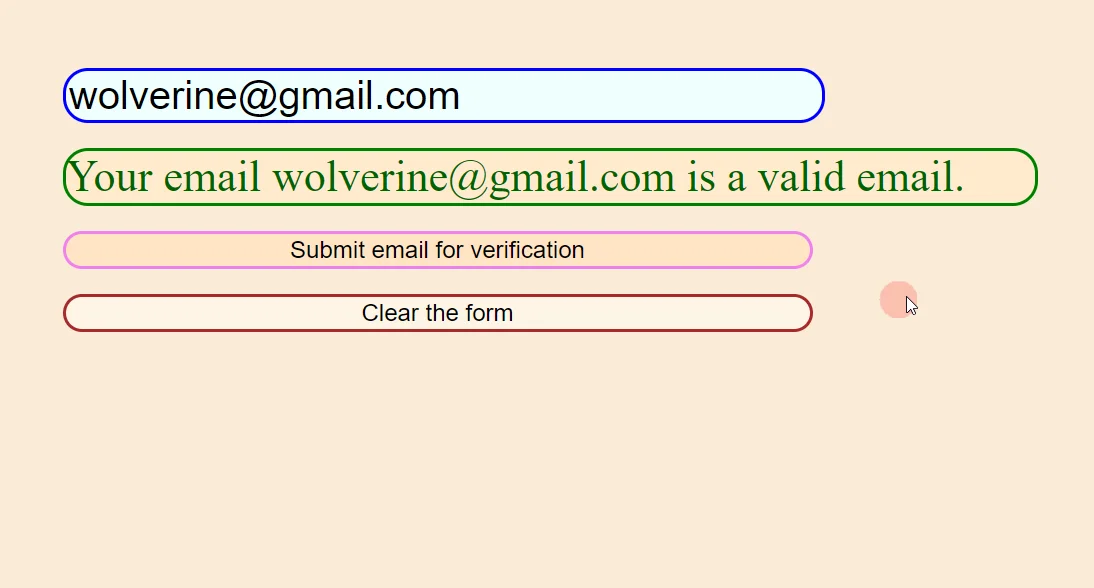
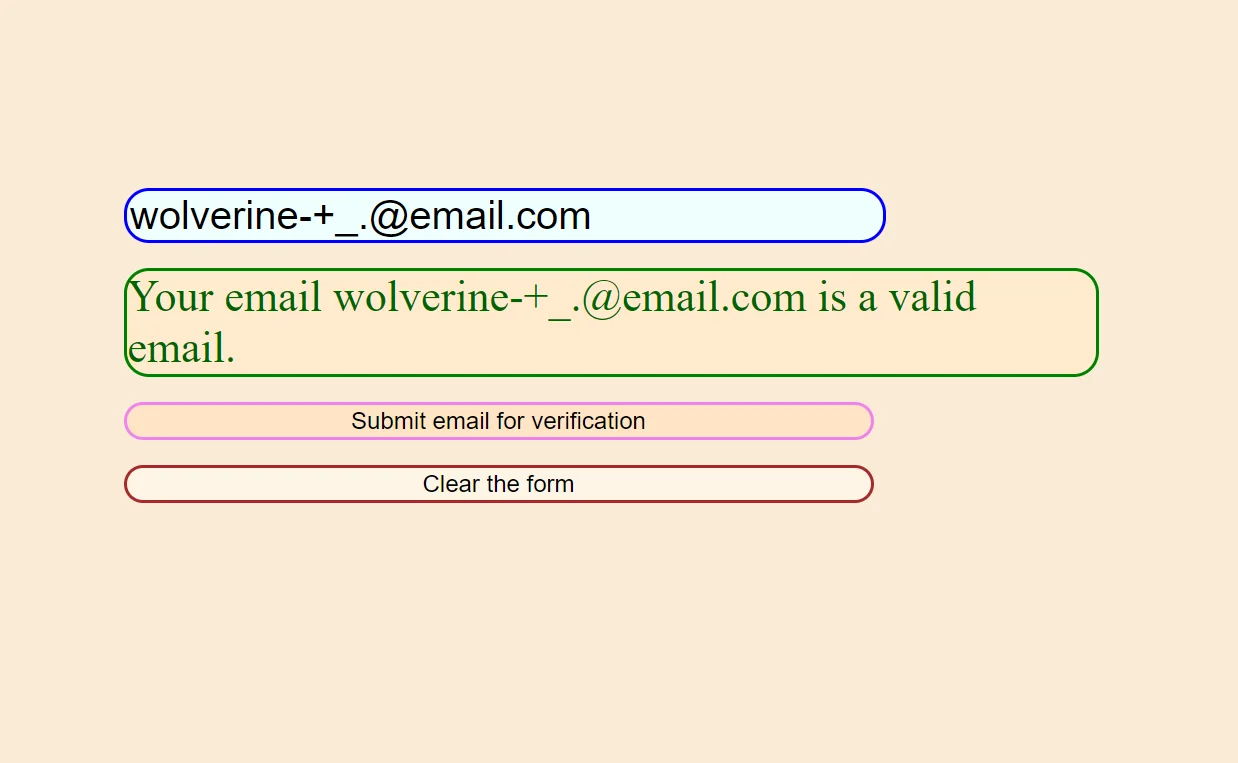
The regex passes email addresses with allowed special characters as valid.

Our regex allows email IDs with multiple labels in the domain part as valid addresses.
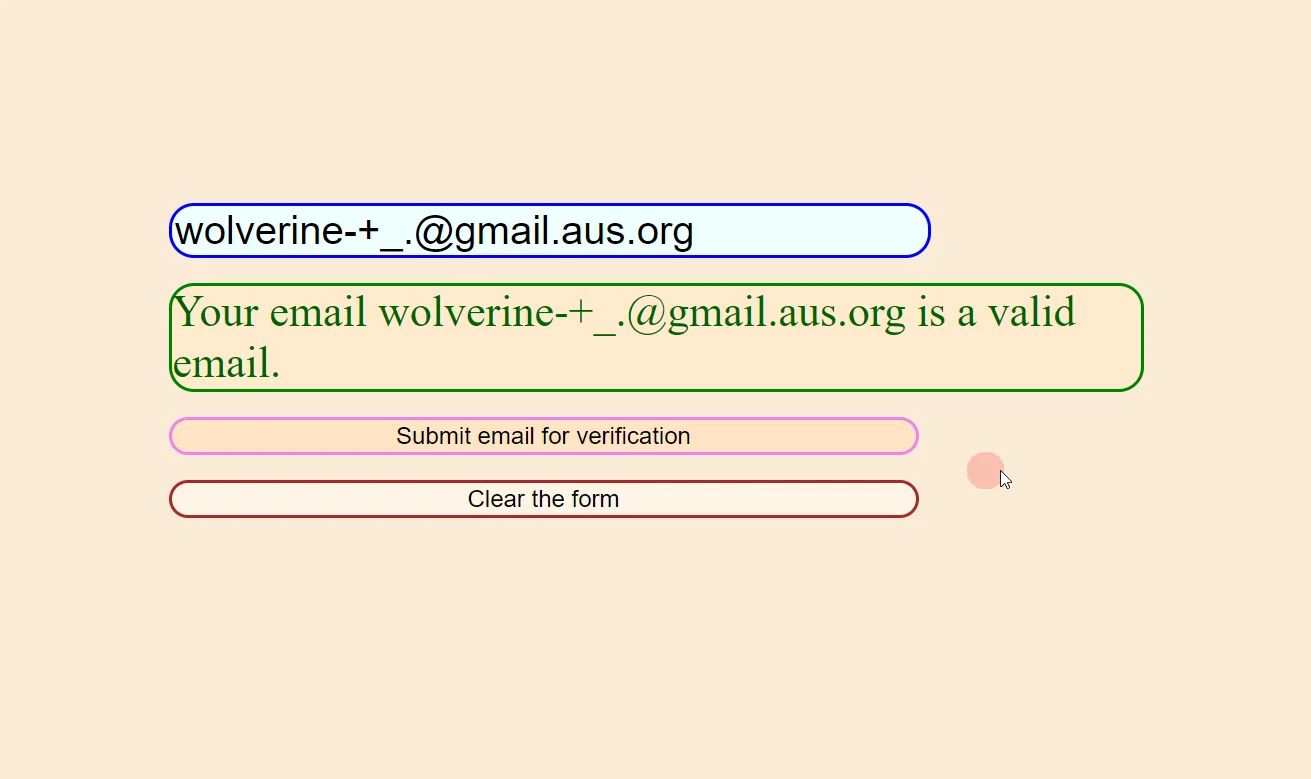
Now, let us see how our regex catches invalid email addresses.

We see above that the TLD is longer than 4 characters. So, our regex catches it and throws up a warning.

We use the disallowed special symbols %, $, and ! in our email address above. So, our regex catches them and throws an alert.
Other Steps in Validating Email Addresses
Besides its syntax, we also need to ensure if the email address exists and is active. For this, we need to query the domain server for the correct MX records (for existence) and proper SMTP settings (for configuration).
Writing a jQuery script from scratch for all the above functionality is a bit complex. So, it is best to use an off-the-shelf easy-to-use email validation API service for this.
Here is the code to use the Abstract API for email validation.
We send a request to the Abstract email validation API with a single line in the $.getJSON() function. We append our unique API key (you can get this when you register for an Abstract API) and the input email ID at the end.
The Abstract API auto-checks the format and the existence of the email address and returns the data as a JSON object.
We check if the key fields of is_valid_format, is_mx_found, and is_smtp_valid are true to mark an email valid.
Let us look at a few demo output cases.

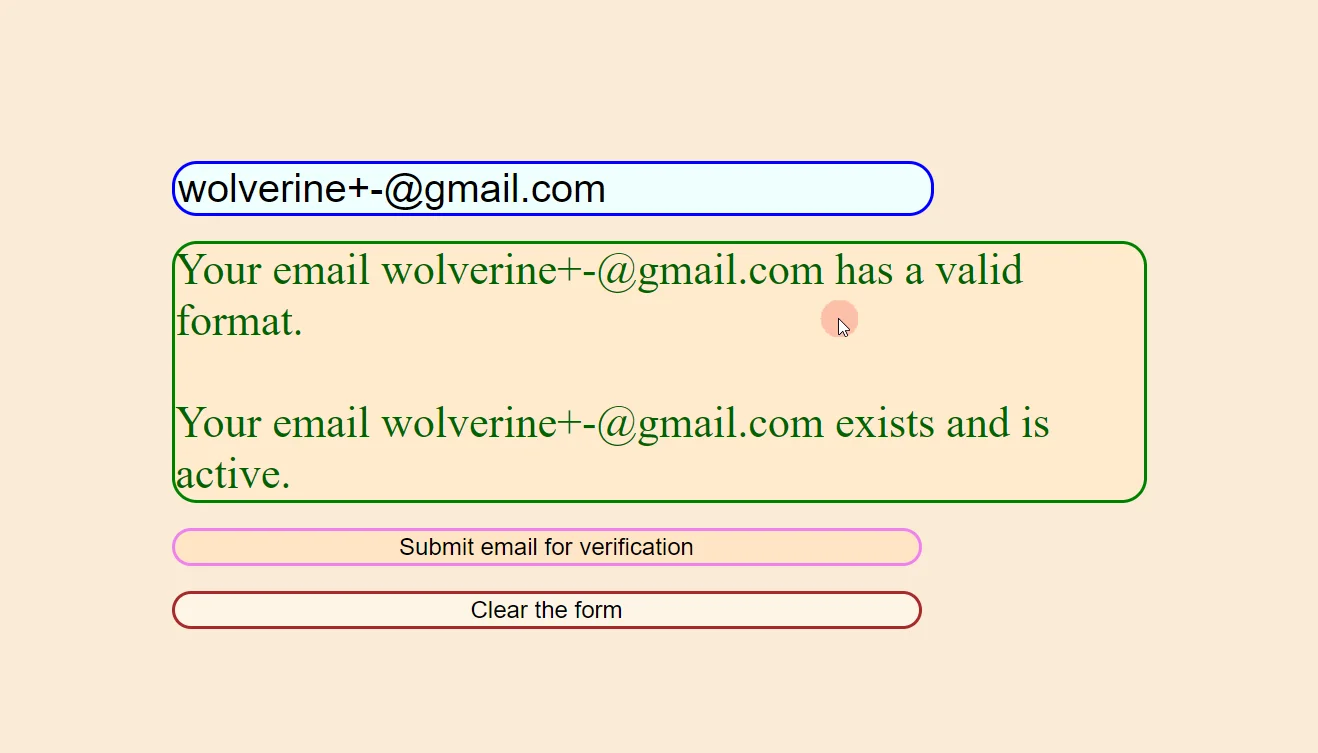
We see above the Abstract email validation API correctly passes email addresses with allowed special characters as valid email IDs.

The Abstract email validation API filters out an email address with a wrong format with a warning alert.
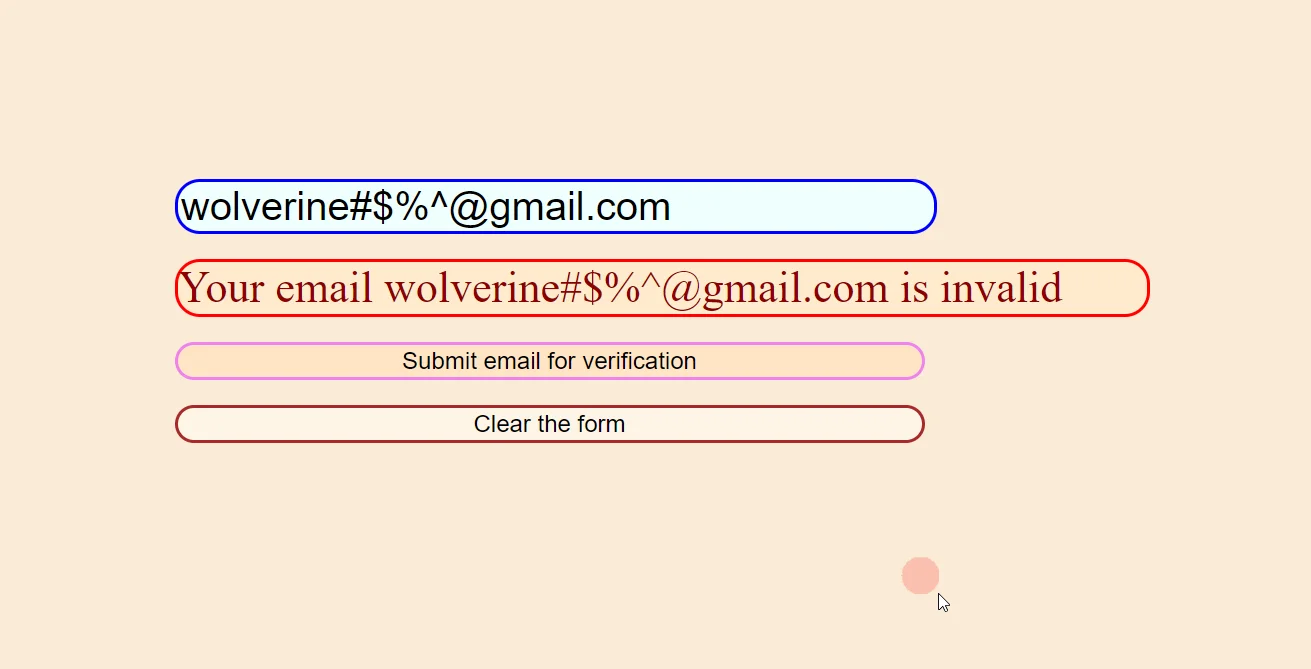
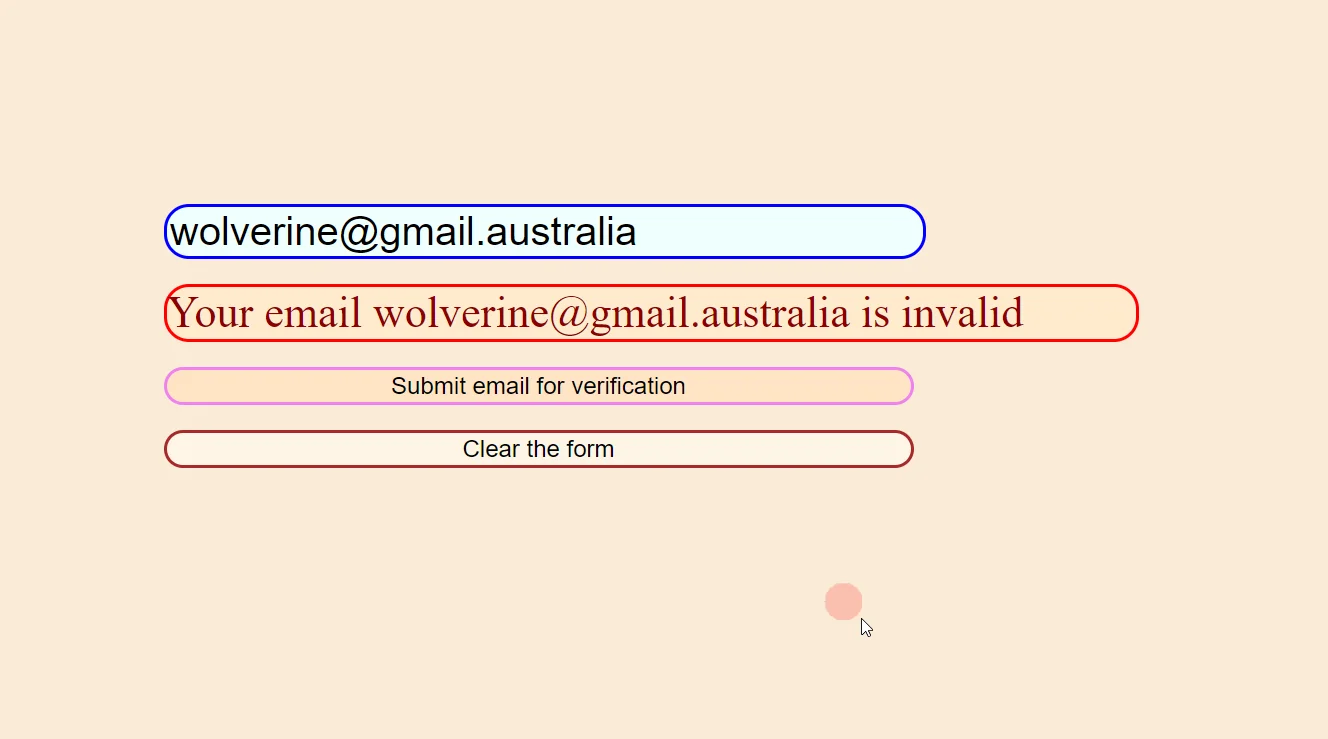
We see the Abstract API catches email addresses with disallowed special symbols and wrong TLD labels (longer than 4 characters).
Email validation is common functionality that developers need in apps. You can now use the different methods in our post to embed email validation in your apps easily!
FAQs
Why do I need to validate email addresses on my mailing list?
Invalid email addresses lead to poor deliverability and open rates. So, you waste dollars spent on email marketing. Also, your sender score drops, and if it gets too low, your email service provider may even ban you from sending out marketing emails.
How does email validation work?
Email validation starts by first checking if the email ID has the correct format. You should use regex patterns for this. Then, you need to confirm if the address exists and is active. You need to query the DNS server, look up MX records and check the SMTP configuration for this. This step can get complex. So, it is best to use an out-of-box email validation API service like the Abstract API for this.
How should I validate email addresses?
Email validation using jQuery is a great way to validate email addresses quickly. You can easily create regex patterns and match email IDs against them in jQuery. But, it is tough to write a DIY script to check if an address exists and is valid. So, you should use the Abstract email validation API for an end-to-end solution to validate email IDs in your list.