IPv4 Addresses
An IPv4 address looks like this
123.456.78.90
There can be slight variations, but in general, the address will consist of one or more sections of one to three digits, separated by periods.
Write the Regular Expression
Create a Regex string that matches a valid IP address for IPv4. The clearest way to do this is the following:
Let’s break down the parts of this expression.
This indicates that we’re looking for any one of the characters inside the brackets. In this case, we’re looking for a digit or numeric character between 0 and 9
The numbers between the curly braces indicate that we’re looking for as few as one or as many as three instances of the previous character. In this case, we’re looking for as few as one or as many as three digits.
In Regex, the “.” character is a special character that means “any character.” In order to find the actual “.” character, we have to escape it with a backslash. This indicates that we are looking for a literal “.” character.
Those three components make up one byte of an IP address (for example, “192.”) To match a complete valid IP address, we repeat this string four times (omitting the final period.)
Use the Regular Expression
Import the Python re module. Use the match() method from the module to check if a given string is a match for our Regex.
Using match()
The match() method takes two arguments: a Regex string and a string to be matched.
If the string is a match, re will create a Match object with information about the match. If we are simply trying to determine that a match was found, we can cast the result to a boolean.
Using search()
The search() method also takes two arguments: a Regex string and a string to be searched.
The difference between search and match is that search will return a boolean value if a match is found in the string.
Check for Numerical Limits
No IP address can contain a string of three numbers greater than 255. What if the user inputs a string that includes a 3-digit sequence greater than 255? Right now, our Regex matcher would return True even though that is an invalid IP address.
We need to perform a final check on our string to make sure that all of the digit groups in it are numerical values between 0 and 255.
The easiest way to do this is to iterate over the string and check each three-digit numerical grouping. If the sequence of digits is greater than 255 or less than 0, return False.
Put It Together
Here’s an example of a complete Regex validation function in Python using re.search() and our custom iterator.
Additional Method
Validate an IP Address Using a Third-Party API
The API allows you to send an IP string for validation, or, if you send an empty request, it will return information about the IP address of the device from which the request was made. This is handy if you also need to know what the IP address of the device is.
Get Started With the API
Acquire an API Key
Go to the API documentation page. You’ll see an example of the API’s JSON response object to the right, and a blue “Get Started” button to the left.
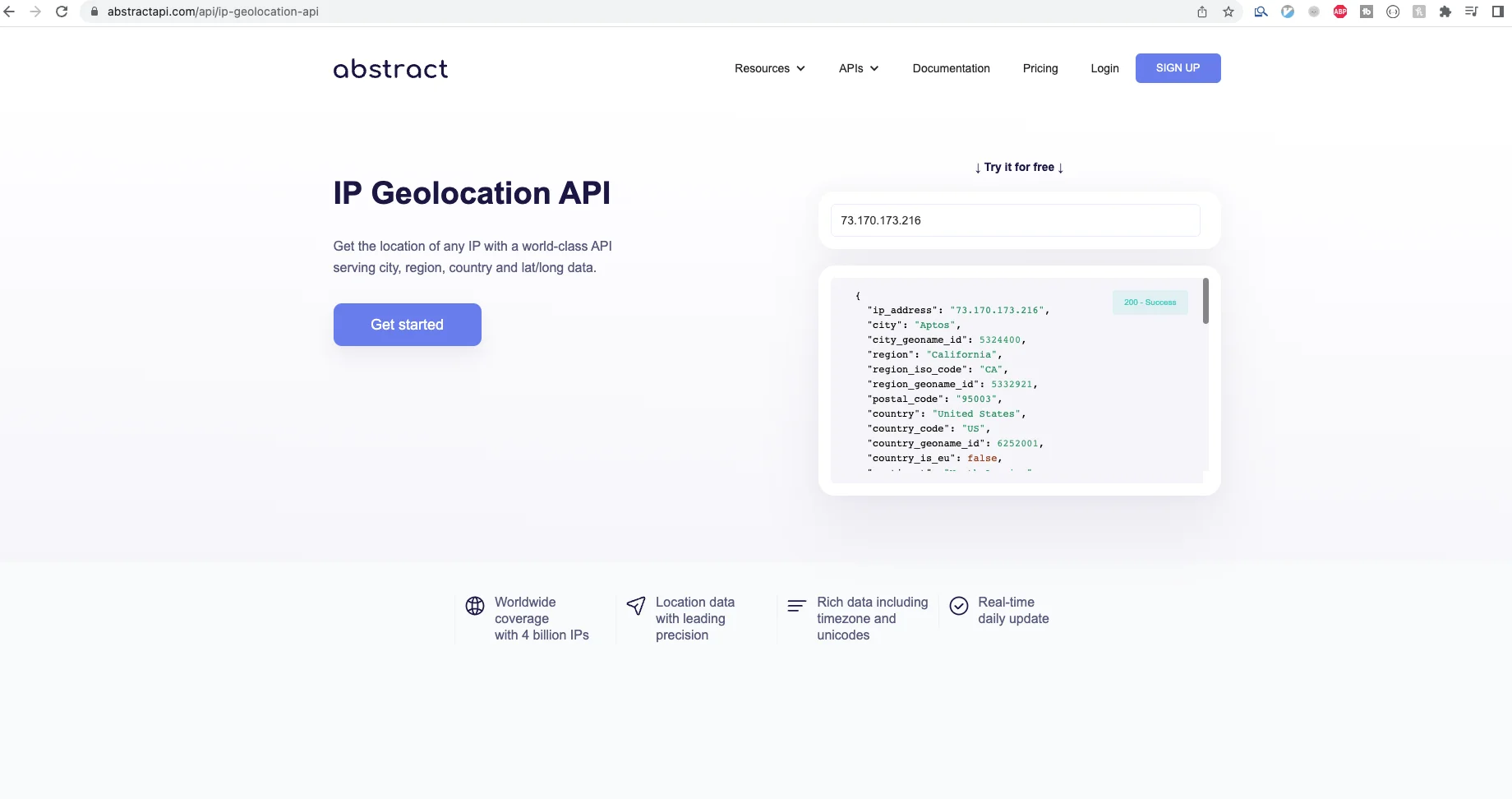
Click “Get Started.” If you’ve never used AbstractAPI before, You’ll need to create a free account with your email and a password. If you have used the API before, you may need to log in.
Once you’ve signed up or logged in, you’ll land on the API’s homepage where you should see options for documentation, pricing, and support, along with your API key and tabs to view test code for supported languages.
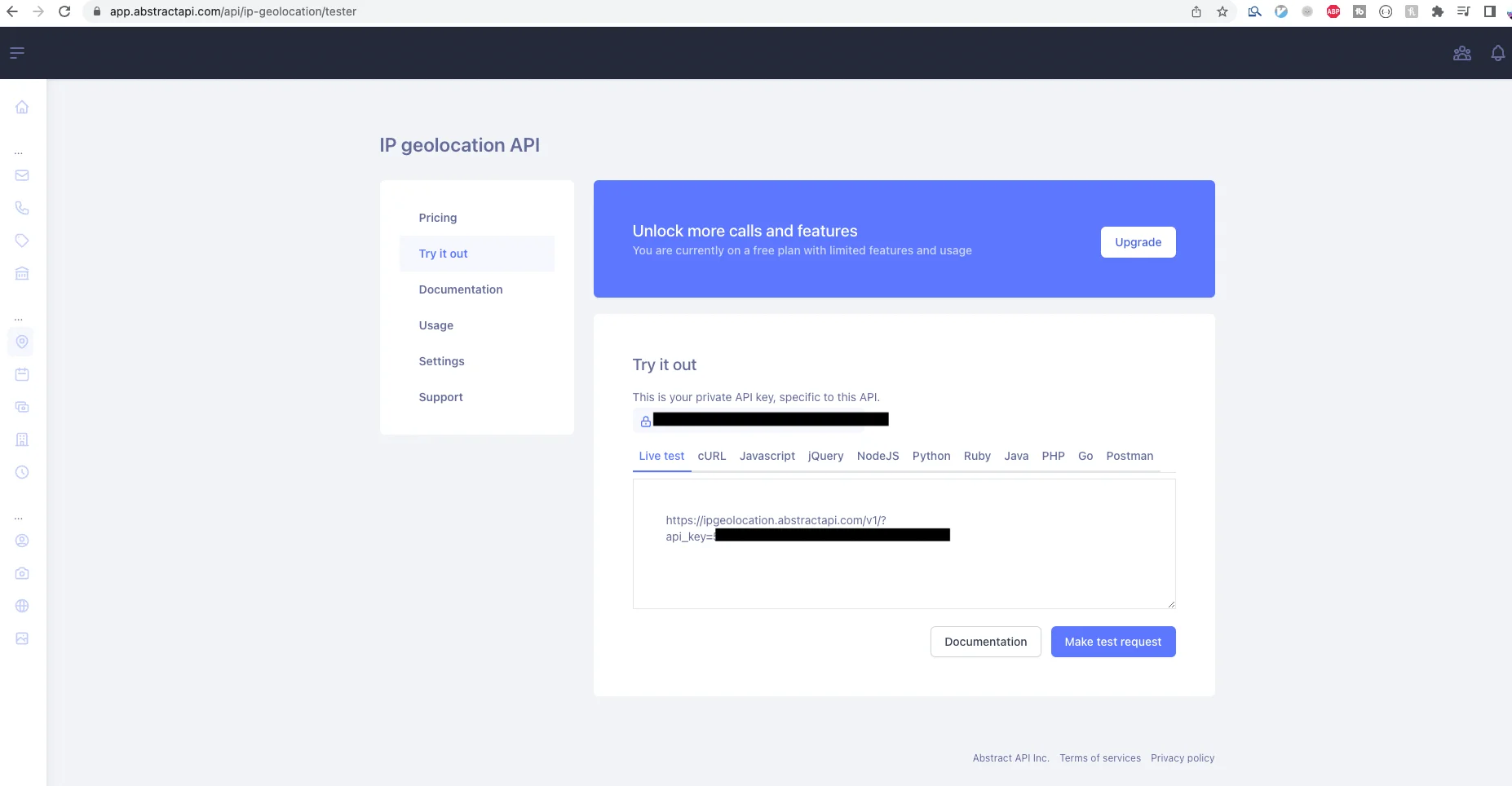
Make an API Request With Python
Your API key is all you need to get information for an IP address. Let’s use the Python requests module to send a request to the API.
When the JSON response comes back, we print it to the console, but in a real app you would use the information in your app. If the IP address is invalid, the API will return an error. Use the error information as your validator.
The JSON response that AbstractAPI sends back looks something like this:
Note: identifying information has been redacted.