Get Client IP Address Using Express
If you're running Express on your Node JS server, it's very easy to get the request's ip address. Express is a lightweight and robust framework for Node JS that provides a set of tools for quickly and easily building web applications.
The Express Request Object
Express wraps the HTTP request from the client in an Express request object, extending the basic HTTP request with handy methods that you can use in to access the properties of the request in your Node app.
The request object is typically written as req, and it is the first argument to any method found on the Express app interface. There are two main ways to pull the IP address from the request object: using request.connection and using request.header.
The Response Object
In addition to the request object, Express also provides a response object as the second argument to the app interface. The response object is used to send information back to the client. In our examples, we'll use this to send the client's IP address back to the browser so we can display it.
Get Client IP Address Using request.socket
One property on the request object is the socket object, which allows you to get information about the request's connection. The request's IP address is a property that is easily accessible on this object. The socket object used to be called connection, but connection was deprecated.
Create a file called server.js, import Express, and use it to spin up a basic application with a single route at '/'.
Inside this route, access the request.connection object by pulling it off the incoming request object.
Open up your browser at https://localhost:3000 and you should see a line of text with the client's IP address. In this case, the client is your device, so the address you'll see will be the loopback address.
If the Express socket property sends back the IPv6 version of the loopback address, you'll see '::1'. You can think of this as the same as '127.0.0.1', which is the IPv4 version of the loopback address.
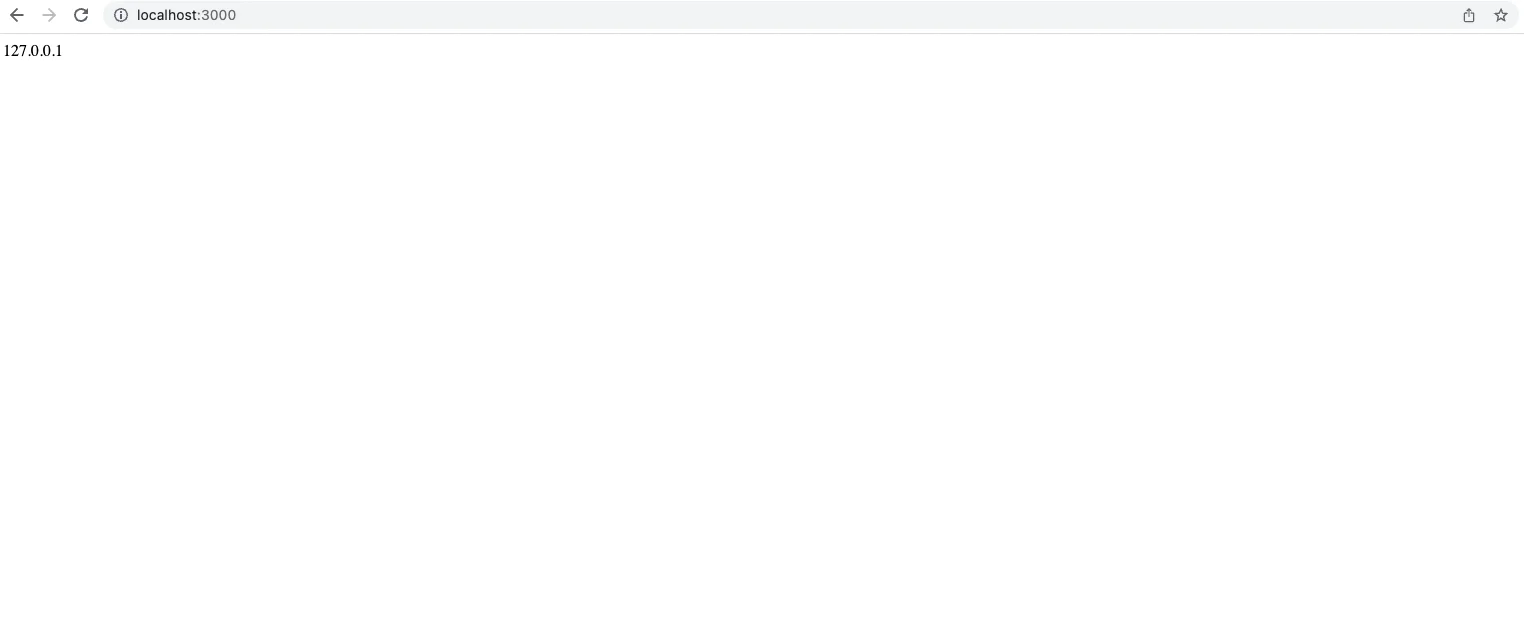
Get Client IP Address Using request.header
If the request was made behind a proxy server, the IP address that you get from the socket object may not be the IP address of the original client. Instead, it will be the last IP address in the forwarded chain. To get the originating client IP address, and other proxy addresses, you'll need to look at the request object's headers.
Any request that is sent on the web contains an HTTP header property with meta information about the request. The request headers object contains fields that handle authentication, tell the server what type of body the request has (for example, JSON vs multipart), and also tells the server information about the client that the request was sent from.
The header that handles IP address information is the x-forwarded field. There are several ways that this field might be named on a request. It may show up as x-forwarded, x-forwarded-for, forwarded-for, or just forwarded.
Usually, the best way to access the field is to use x forwarded for.
In your server file, remove everything inside the app.get request, and replace it with the following solution:
Be aware that x-forwarded-for might return multiple ip addresses, in the format “client IP, proxy 1 IP, proxy 2 IP.” In this case, we are only interested in the first one.
This solution works only if the client request came from a proxy server and was forwarded. You should not rely on this as the only solution because if the request did not come from a proxy server, then the x forwarded for the field will be undefined. Keep the request.socket solution as a fallback.
Get Client IP Address Using Request-IP
You can install an NPM package called request-ip to look up the client IP address in Node.js. This package is middleware that sits in between an incoming request and the rest of your application.
Middleware modifies a request object or performs some side-effect function using the request object, then sends the object along to the next link in the middleware chain, or to the app itself.
Get started by installing the package using the command line.
Then import the package into your server file and replace your existing solution with the package's getClientIP function.
Head back over to https://localhost:3000 to check out your IP address in the browser (remember, if you're using IPv6, you'll see ::1, and if you're using IPv4, you'll see 127.0.0.1.)
Get IP Address Using IP
In both of these examples, we've been displaying the local IP address because that's where the request originated from. But what if we want to get the local IP address of our device while it's in production and we're not making a loopback request?
In that case, we can use a handy NPM package called ip. This package works similarly to request-ip. It allows you to access your local IP address and also provides a lot of useful methods for validating IP addresses, comparing IP addresses, and manipulating IP addresses.
Install it in the same way
Import it into your file and use it in your app.get function.
Head over to the browser to see your client IP address. Notice how it is no longer a loopback address. This is the actual client IP address on the current network. It will most likely be a 192.168.XXX.X address.

Find a User’s Location Using AbstractAPI's IP Geolocation API
Once you have the client's IP address, you can use that IP address to determine the user’s geographical location.
AbstractAPI is a simple REST API that accepts a request with an IP string, and returns a JSON response with information about that IP address.
Once we've extracted our request IP address from the incoming HTTP request, we can send it to the AbstractAPI endpoint to get geolocation information.
Get an API Key
First, you'll need to acquire an API key by going to the AbstractAPI Geolocation API homepage, clicking "Get Started" and signing up for a free account. Once you land on the API homepage, you'll see your API key.
Install Axios
The easiest way to send an HTTP request in Node is to install axios, a third-party HTTP request client.
Send the Request IP to the API
Create a new function called sendAPIRequest. The function should take an IP address string as its only argument. Head back over to AbstractAPI and copy the endpoint URL and your API key.
Don't forget to swap out your API key for the temp variable here. Head back over to https://localhost:3000 to check out the IP address information in your browser.
Note that because the address you're sending to the API in development is just a 192.168 address, you won't get much useful information from it. Usually, a complete response object will look something like this:
(Note that I've obscured a lot of identifying information in this snippet.)
Conclusion
In this article, we looked at how to extract a client IP address in Node.js, and how to use the AbstractAPI geolocation endpoint to get some useful information about that IP address.
Once you have this information, it's easy to customize your users' in-app experience to provide translations, currency conversions, etc.
FAQs
How Do I Find My IP Address in Node.js?
The easiest way to find your IP address in Node.js is to pull the client IP address from the incoming HTTP request object. If you are running Express in your Node app, this is very easy to do. Access the socket field of the Express request object, and then look up the remoteAddress property of that field.
The remoteAddress will be the address of the client that sent the request. If you are running the app in development, this will be the loopback address: 127.0.0.1 for IPv4 and ::1 for IPv6.
Can Javascript Read IP Address?
Yes. It's very easy to look up an IP address in Javascript. If you are running your Javascript app in the browser, you can use a free third-party service like AbstractAPI to make a request and get both your IP address and some information about it.
If you're running Javascript on the server (i.e. a Node JS server), the Express framework makes it easy to pull information about the client IP address from the incoming request object. The easiest way to do this is to use the request.socket.remoteAddress field.
How Do You Look Up an IP Address?
There are many ways to look up an IP address. If you simply want to know your own IP address for the device you are currently using, go to a site like https://whatismyipaddress.com/, or simple type "what's my IP address" into a Google browser or search bar.
If you are trying to look up an IP address inside the browser or on the server, the easiest way is to examine the incoming request object. Depending on the framework and language you are using to build your server, the exact method for doing this will differ. Look at the documentation for your language and framework.
There are many free third-party services that can give you not just an IP address but also some information about it, like geolocation data, carrier, and provider data, etc.