Validate Email Address Using Python Regex
The first method we'll look at for validating email addresses is using Regex. We're also going to talk about why you should not use Regular expressions to validate email addresses in your application.
Using Regex in Python
To use Regex, we need to import the Python re module. Next, we’ll use the .match() method from the module to match a string input against our Regex expression.
The output of running re.match() will look something like this:
If the string you pass to re.match() matches the provided pattern, re creates a Match object with information about the match that was found. We don’t need all that info to do a simple validation check, however, Match returns null if not match was found, so we can treat the result as a boolean.
If we want an explicit boolean response from a function, we can cast the result to a boolean
Validating Emails With Regular Expressions
The above code could be used in a valid email check that checks whether an email address exists when the server receives a login attempt or sign up request from your front end. The valid email check could look something like this:
The Difference Between Match and Search
The Python regex module contains two primitive methods for evaluating a string: match and search. These perform very similar functions and can both be used to validate an email address in Python. The difference is that search checks for a search pattern match anywhere in the string, while match checks for a match only from the beginning.
Why You Shouldn't Validate Emails Using Regex
Using Regular expressions to validate email addresses is not recommended for several reasons.
It's Almost Impossible to Match All Valid Email Addresses
Most people don't really understand how Regex works. Usually, when you find Regex in a codebase, it's been copied and pasted from somewhere, or built using the help of a Regex Helper.
Most of the examples you will find out there for matching emails with Regex will be incomplete, too restrictive, or will fail to match all valid email addresses, such as internationalized email addresses.
It Doesn't Check for Domain Validity
Using Regex to check whether an address exists won't actually tell you whether the address exists. All it will tell you is whether the email address string that was provided is well-formatted and looks like an email address (and, as mentioned in the last paragraph, sometimes it doesn't even do that.)
In order to catch an invalid email, you need to check whether the domain part exists, whether the user account exists, whether it is a known spam account and a whole host of other things.
It Doesn't Prevent Temporary Addresses Getting Through
Even if an email address can be proven to be from a valid domain and user account, it could still be an invalid email for other reasons. Temporary mailbox providers allow users to create valid email addresses that last for only 10-15 minutes before expiring. If you are collecting user emails for a newsletter or some other marketing campaign, that means you could easily end up with a lot of useless email addresses on your mailing list.
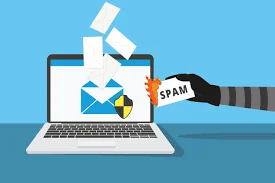
Validate Email Address Using Python Library
Another way to check for email validity is using a package or library dedicated to the task. One such library is the email-validator library. This library validates not only the syntax of a given address but also checks the deliverability.
The library provides user-friendly error messages when validation requirements are not met, supports internationalized email addresses and domain names, converts emails into a normalized format, and blocks unsafe characters. It rejects emails with null MX records.
To use the library, install it using Pip or Pip3.
The package exports several methods that you can import into your modules, along with error messages. To use the package to validate an incoming email for signup, for example, you can do the following:
This validates the email and returns the normalized format. Other error messages exported by the package include EmailUndeliverableError and EmailSyntaxError, which are both subclasses of the EmailNotValidError.
This is certainly a step up from using basic Regex to validate an email. The only drawbacks of using this library are that it doesn't handle obsolete addresses (for that, use PlysEmail) and that it isn't intended to validate the "To" field of an email (for that, use Flanker.)
Validate Email Addresses Using AbstractAPI
Arguably the best and most robust method for validating emails in Python is to use a dedicated third-party API. APIs such as the AbstractAPI Free Email Validation API provide a secure REST endpoint that accepts an email address string and returns a JSON response containing information about the validity of the address.
The AbstractAPI endpoint is actively maintained, making it more reliable than a library, which may often be outdated. It checks syntax, domain validity, MX records, and verifies SMTP servers. In addition, it can also tell you whether the email address is a free email, a temporary email, or a role email (i.e. staff@domain.com.)
The API can also validate bulk emails. Simply upload a CSV file containing a list of emails, and the API will email you a report on the validity of each email on the list. This is great for auditing a mailing list.
Acquire an API Key
Navigate to the API Get Started page. You’ll see an example of the API’s JSON response object, and a blue “Get Started” button on the right. Click “Get Started.”

If you’ve never used AbstractAPI before, You’ll need to input your email and create a password. If you have used Abstract before, you may need to log in.

Once you’ve logged in, you’ll land on the API’s homepage. You’ll see options for documentation, pricing, and support, and you’ll see your API key. All Abstract APIs have unique keys, so even if you’ve used the API before, this key will be new.
Use Your API Key to Send a Request to the API
We’ll use the requests module to send a GET request to the AbstractAPI endpoint, so let’s install that first.
Next, we’ll write a function called sendEmailValidationRequest to send the request to the API and examine the JSON result.
The response data should look something like this:
The best way to determine that the email address is valid, well-formatted and deliverable is to create a helper function that checks all of those fields and will return True only if all validity requirements are met.
This function will check every value in the JSON object and return False if any value indicates that it is an invalid email.
Conclusion
In this tutorial we looked at three methods for determining whether an email address exists in Python: regex, a Python library, and a dedicated validation API. The method you choose for your Python app will depend on your unique needs and the scale of your application.
For a test app, an app that won't be widely used, or an app where the email parameters are known and controllable, using Regex might be a viable option. For anything else, however, we recommend choosing one of the excellent Python packages or using a dedicated API.
FAQs
How Do You Write a Regex for An Email Validation in Python?
While there are general-purpose email Regex patterns that can be used to validate email address format in Python, it's not recommended to use Regex as an email validator in production. Beyond simply validating the syntax of the address string, you must also verify that the email domain exists, that it is not a spam domain, that the MX records are current, and that the address is not temporary or a role-based email account. Regex cannot tell you any of these things.
If you must use a regular expression to validate an email address, it's recommended that you use the RFC5322-approved pattern for email addresses. That looks like this:
How Do I Know If a Python Email is Real?
There are a few ways of determining if an email address is valid in Python. To start, you could use Regex to validate the syntax of the string (although this is not recommended as it is error-prone and does not provide a complete validation picture.)
You can also use several Python email validation libraries such as the email-validator library, PlysEmail, or Flanker.
What Is the Simplest Regular Expression for Email Validation?
The simplest regular expressions for email validation look for the presence of a username and domain, separated by the '@' symbol and followed by '.com' or some other domain extension. The most basic check would be the following:
^(.+)@(.+)$
It is not recommended that you use a basic regular expression like this to validate emails in your app as it does not catch every type of email address.