How to Find the Location of a User
There are several ways to find out the geographic location of a user. One of the most frequently used methods is to use the Geolocation API in the user’s web browser. Another easy and convenient method is to use a third-party dedicated geolocation API to analyze the user’s IP address. If you are building a React app, there are also several libraries and packages that provide geolocation data.
In this article, we’ll take a look at how to geolocate a user in a React app. We’ll look at two methods: the in-browser Geolocation API, and a dedicated IP geolocation API from AbstractAPI.
Getting Started
Spin up a simple React app using create-react-app:
This should give you everything you need to get started. We don’t need to install any extra packages yet, because we’re using the browser-based API that should be built into every web browser.
To check that things are working, start up the app
and visit http://localhost:3000. You should see a page displaying the Create React App starter page:
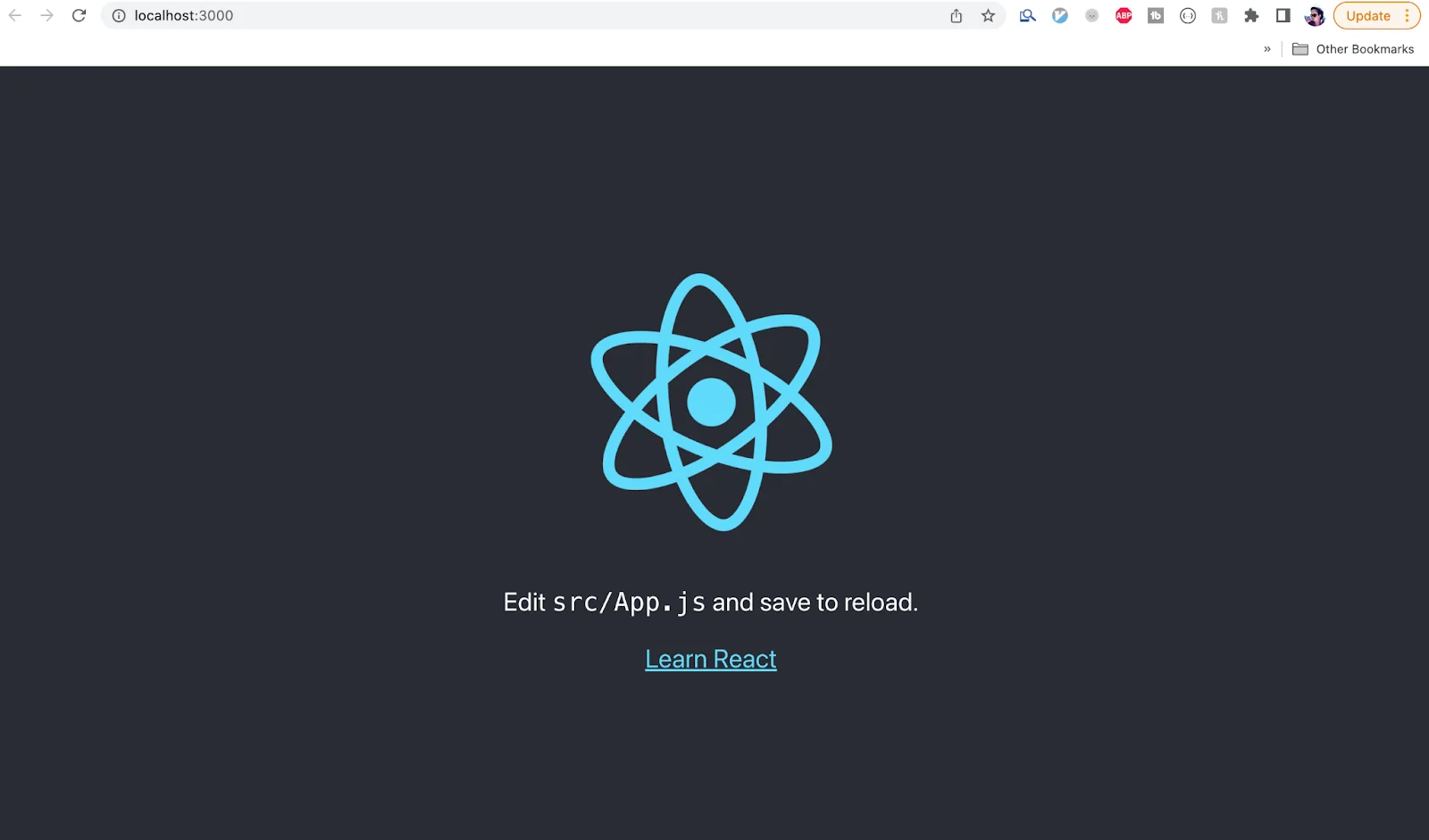
Great! We’re now ready to start geolocating.
How to Use the In-Browser Geolocation API
The Geolocation API is a browser API that allows the user to provide their location to a website. If you’ve ever visited a website and seen a dialog box pop up that looks like this:

then you’ve been on a website that is using the Geolocation API. The API provides methods to ask the user for permission to access their current location. If the user grants permission, the API returns a Geolocation object with the user’s latitude and longitude coordinates, which you can then use in your app.
Edit App.js
In the existing App.js file that Create React App made for us, remove the boilerplate code and add some state using the useState hook:
The lat and long variables are where we will store our latitude and longitude to display to the user.
Write a Get Location Function
We’ll use the Navigator interface from the browser API to get the information we need. The Navigator object contains information about the user’s current state and device. To get geolocation information, we can use navigator.geolocation to access the Geolocation API.
Now, we’ll write a getLocation function that uses the geolocationAPI to get the user’s coordinates.
There’s a lot going on here, so let’s break it down.
First, we check to make sure that the call to navigator.geolocation returned the expected interface. If the response from that call was null, then the browser can’t access the Geolocation API and we need to show an error.
If the Geolocation API is available, we make a call to getCurrentPostion(), which accepts a success callback and a failure callback as arguments. getCurrentPosition will first ask the user for permission to access their position. If the user grants permission, the success callback will run, providing us with the user’s position. Inside the callback, we grab the user’s coordinates from the returned position object and set them in the app state.
If the user does not grant permission, or if something else goes wrong while getting the user’s position, the failure callback will run. In this case, we set an error to show to the user.
Display the Results
Now that we have our latitude and longitude coordinates, we can do whatever we need to with those. The most common use for these coordinates would be to show the user’s location on a map. For now, we’ll just display the coordinates to the user.
First, add a simple <p> tag inside the <div> where the boilerplate code used to be.
When the page first renders, the lat and long values will be null, so nothing will display. Let’s call our getUserCoordinatesFunction when the page loads.
Now, on page load, the dialog box that asks the user for permission to know their location will pop up
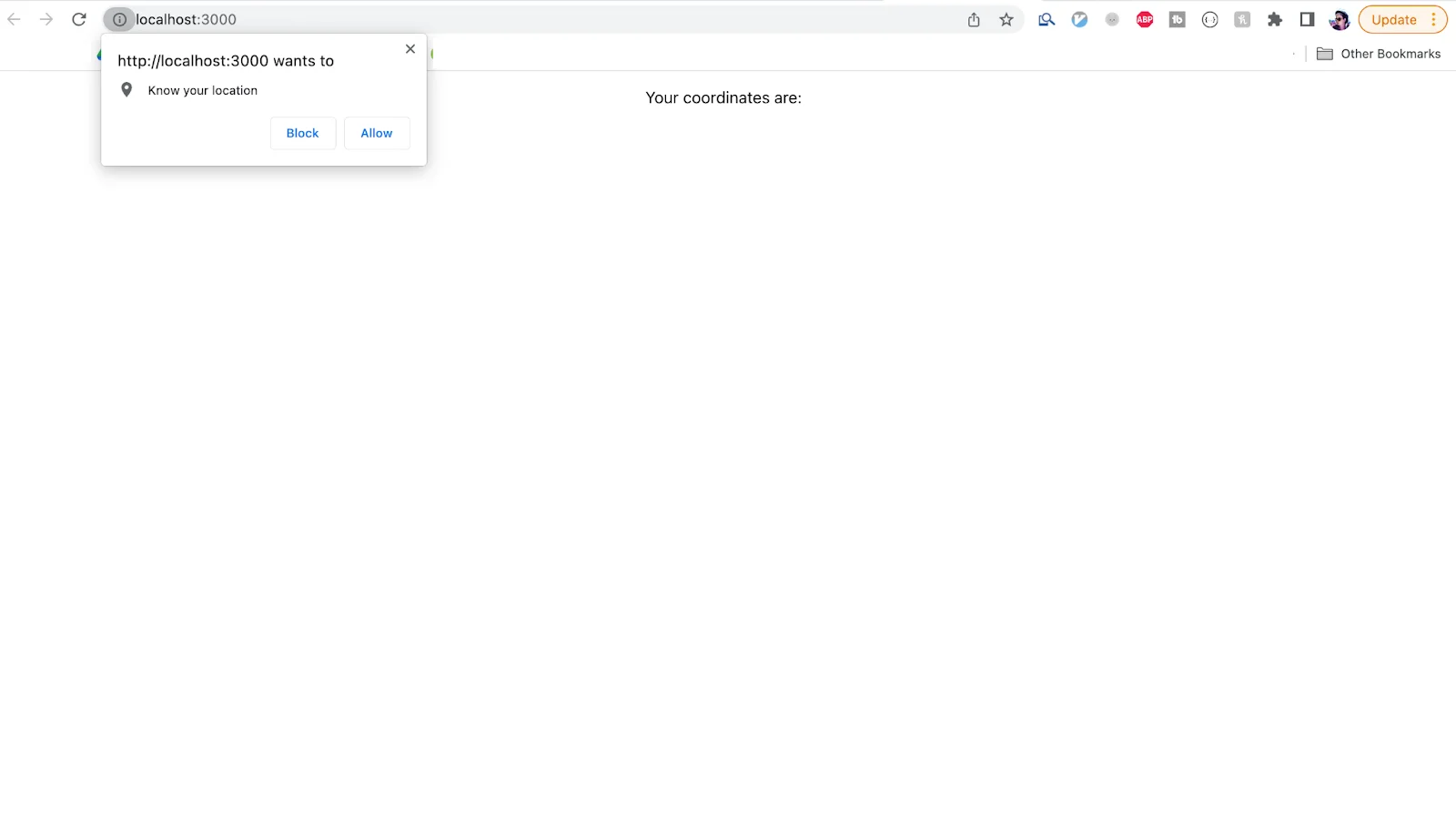
When the user hits “Allow,” the Geolocation API will return the coordinates. We update the state with the new values, which renders the coordinates inside our <p> tag.

That’s it! We successfully geolocated our user.
How to Use the AbstractAPI IP Geolocation API
What if you want more information than just the latitude and longitude? For that, you’ll need to use an IP geolocation service. Let’s take a look at how to use the AbstractAPI service to geolocate a user through their IP address.
Get Started With the API
Acquire an API Key
Navigate to the API documentation page. You’ll see an example of the API’s JSON response object, and a blue “Get Started” button.
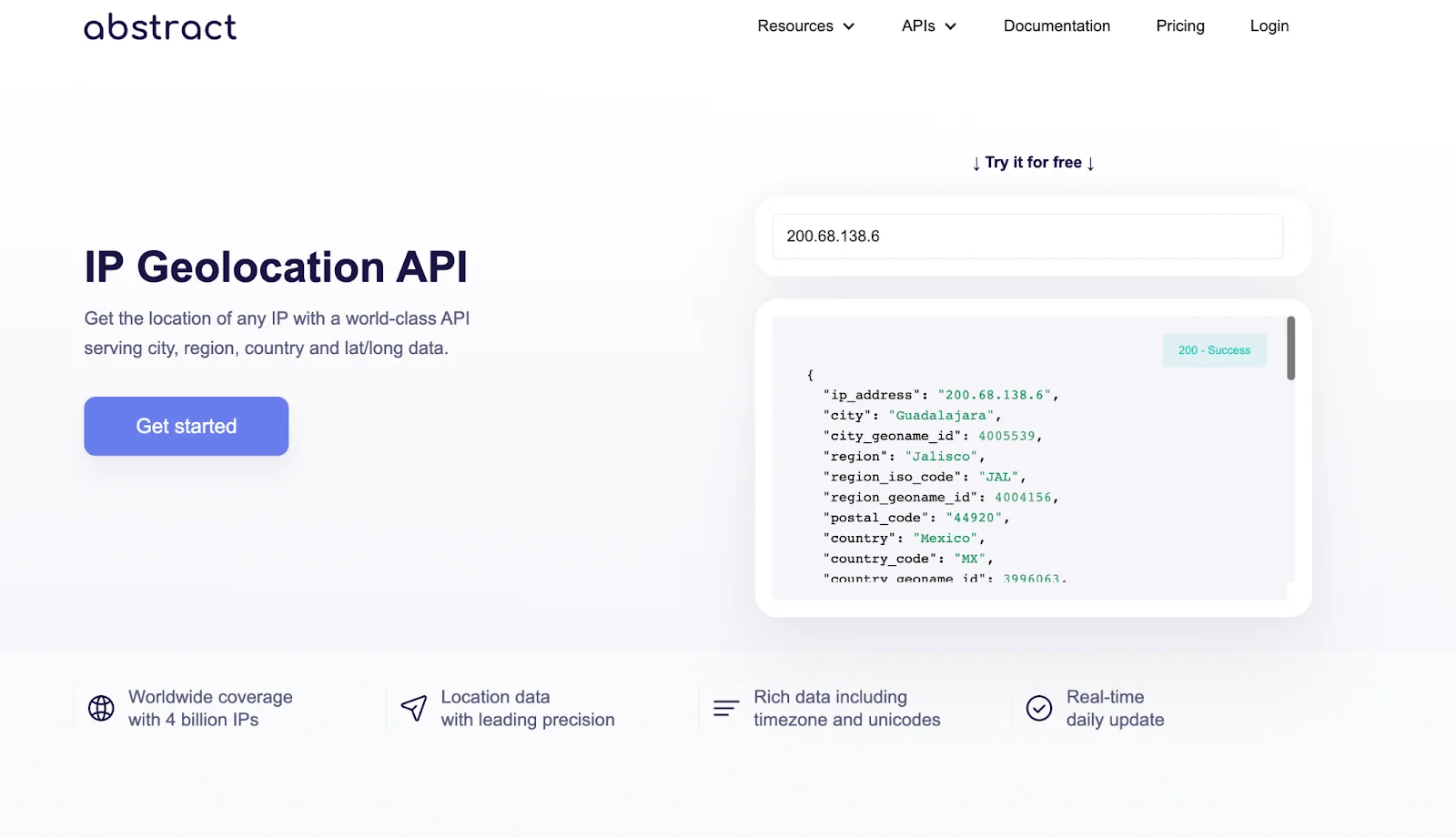
Click the “Get Started” button. If you’ve never used AbstractAPI before, You’ll be taken to a page where you’re asked to input your email and create a password. If you have used Abstract before, you may be asked to log in.
Once you’ve signed up or logged in, you’ll land on the API’s homepage where you should see options for documentation, pricing, and support. You’ll also see your API key.
Use the Key to Send a Request to the API
We’ll use Axios to send a GET request to the AbstractAPI endpoint, so let’s install that first.
Next, we’ll write a function called getUserLocationFromAPI to send the request to the API and examine the JSON result.
When you look at the log of the response data, you should see a JSON object like this:
(Note: identifying information has been redacted to retain privacy of the author.)
As you can see, a lot of information is returned here. This information would be useful if we wanted to display the correct currency to the user, show them their home flag, or the correct time, or show them localized results for their city.
For now, let’s pull the latitude and longitude out and display those to the user like we did before.
Now, let’s replace the getUserCoordinates call with the new function
That’s it! We’ve now looked at two completely different methods for determining a user’s location in a React app.
Conclusion
Determining a user’s location is very useful in an app. As you can see, there are many ways to do it. Which method you choose depends on what type of information you need and what kind of app you are building. Always consider the needs of your particular app so you don’t end up overegineering or underengineering your solution.
FAQs
What is geolocation?
Geolocation, also known as geopositioning or geotracking, is the process of figuring out the geographic position of an object. The term doesn’t just apply to web apps and IP addresses—anything on earth can be geolocated to a set of coordinates.
When we talk about geolocating within the context of an app, we’re usually talking about determining the user’s position and using that information to show something to the user.
How do I determine a location from an IP address?
There are many ways to determine a user’s location using their IP. One of the most convenient methods is to use a dedicated geolocation API, such as AbstractAPI’s IP Geolocation API. If you are using React, you could use a React geolocation package like react-ipgeolocation. If the user will be accessing the app via a website, you can use the browser’s built-in Geolocation API.
How Accurate is IP-Based Geolocation?
IP-based geolocation is quite accurate. It can get between 55 percent to 80 percent accuracy for a user's region or state. All geolocation APIs should return to you an accuracy mesaure along with the result, which give tell you a radius around the GPS coordinates.