1. Retrieve User IP Address From the Request Object's Socket Property
First up, we find the request's IP address with a simple vanilla Node JS solution.
Node's standard http module provides the remoteAddress property in the socket property of the request object. We retrieve the request's IP address from this value.
Here is the code for this solution.
The code logs the address in the console.
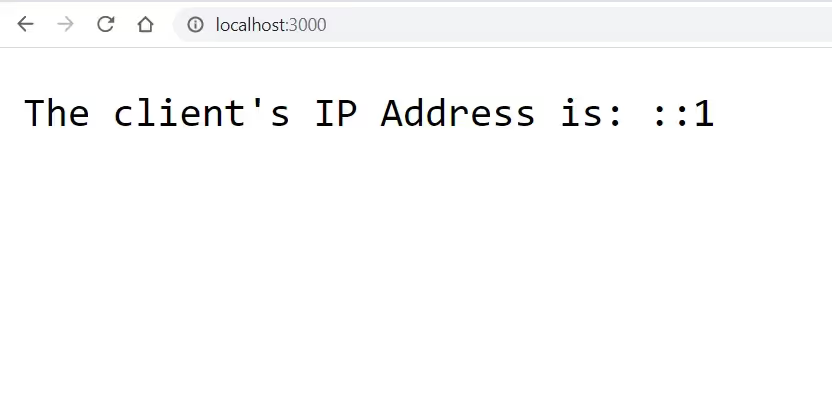
We display the user IP address using IPv6 above. It is a short form of localhost:
0000:0000:0000:0000:0000:0000:0000:0001
which IPv6 abbreviates to ::1.
2. Retrieve Multiple IP Addresses With the X-Forwarded-For (XFF) Header
The above simple solution works only if the user directly connects to the server. But, if the request routes through multiple proxies, the req.socket.remoteAddress property only logs the final proxy address.
But, we need the originating client's IP address for our business use case. So, we use X-Forwarded-For (XFF), a de-facto HTTP header for this.
XFF has the following syntax:
We parse it to extract the left-most value. This is our request's IP address. Here is the code for it in Node JS:
Let's quickly break down the code logic.
We access the XFF header in the req.headers object with the key ['x-forwarded=for'] to return multiple IP addresses of the proxies and originating client.
Sometimes, the XFF header may not be present. So, we use the modern JavaScript optional chaining (?.) operator in the command to do away with an error in such a situation. Then, we split the XFF header by commas to return multiple IP addresses as an array. Finally, we retrieve the client IP address with the JavaScript shift array method to extract the left-most value.
If they do not set XFF, the optional chaining operator will return undefined. To provide the user with a meaningful response, we default to the req.socket?.remoteAddress value.
Here is the output for this code. We see the IP address in the log in our console.

Once you’ve obtained the client’s IP address, you can integrate it into broader security workflows. For example, combining IP tracking with Firebase email validation can help prevent fraudulent sign-ups by verifying email authenticity and identifying suspicious login patterns based on IP data.
3. Request Local IP Address With Node JS
The above two methods return localhost. Localhost means a loop back to the same device that we request IP from. But, our device also has a local area connection to our router. So, it also has a private local IP address.
We use a Node JS module called ip to request this local IP address.
We first install the ip module with the following command:
We now request the IP address in our local area network with the ip.address() method of this module.
Here is an example Node JS application to do so:
We now run our app. The output on localhost:3000 looks like this:
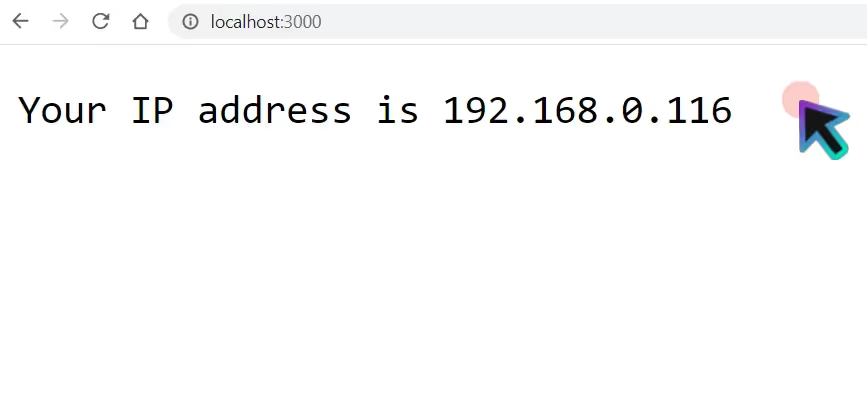
4. Retrieve Client IP Address in Node JS with Express
Express stores the originating IP address in the req.ip property of its request object.
Here is the example code for this solution:
The code is easier to write if we use Express on top of Node JS.
5. Request Client IP Address When Using Edge Computing Services
With the volume of data expanding each day, the traditional client-server model for web apps is not enough. Many times, you might have your server behind proxies to deliver superior performance and security using edge computing and content delivery networks (CDN).
In that case, you need specific headers that different edge computing companies support to retrieve the client's original IP address.
Let us look at a few of these specific headers.
Request IP Address in Cloudflare
If you use a Cloudflare network of proxies to improve speed and security, you can get the request's IP address with Cloudflare's custom headers:
CF-Connecting-IP Header
Cloudflare provides their proprietary CF-Connecting-IP request header to send the client IP address to your origin web server.
True-Client-IP
Enterprise customers of Cloudflare can also use the True-Client-IP header to get the request's IP address. The True-Client-IP header is best for legacy devices to read the original IP without updating firewalls/load balancers.
Request IP Address of Client in Fastly CDN
If you use Fastly CDN to provide your app or website visitors with a fast user experience, use their proprietary Fastly-Client-IP header to get the request's IP address. As the requests jump from one Fastly proxy to another, Fastly automatically adds this Fastly-Client IP header to subsequent requests.
Request IP Address of Client in FireBase Hosting
If you host your app on Firebase, you can retrieve the original IP address from a Firebase hosting header. You can specify a Firebase hosting header in the 'headers' attribute in the config file.
Client IP Address in Nginx
If you use Nginx proxy servers to improve your site performance, you can retrieve the client IP from the X-Real-IP header.
Client IP in Rackspace Load Balancer
Rackspace lb optimizes site performance by distributing load along with caching and other features. You can find the original client IP in the X-Cluster-Client-IP header in Rackspace lb.
You can follow along with the official tutorials and docs for any of these services to install them properly with the specific headers to get the client's IP address. You can find example tutorials for all major platforms like Windows, Mac OS, and Linux for these services.
6. Robust Solution to Retrieve Client IP Address in Node JS - Use the Abstract IP Geolocation API Integration
As we see, the original client IP address may hide in several places - inside native Node JS objects (the req.socket.remoteAddress property), any Node JS framework object (req.ip property in Express), or inside several custom specific headers if you use edge computing and CDNs to improve your site performance.
It's tough to keep track of individual headers and the different configuration commands for any full stack developer to get the client IP address when you use different proxy companies.
So, we can use an end-to-end pro-grade geolocation service like the Abstract IP Geolocation API to cover the different cases.
Note: Read more about the Abstract IP Geolocation API here.
With an easy JavasScript code snippet, you can use the Abstract IP Geolocation API to retrieve the client IP address in Node JS with the following solution:
Here is the output of this example:

The logic is straightforward. We send an Axios request to the API end-point. It returns a rich set of geolocation data in the response object as JSON. The first field in the response data is ip_address, and it contains the original client IP address.
The API call looks up in several headers and JavaScript/Node JS objects to retrieve the IP. The full stack developer need not waste effort on looking up each individual request header, and can focus on the business logic.
Abstract API provides several useful ready-to-use integrations for many common tasks a full stack developer needs. For example, their Email Validation API provides a full stack developer with a plug-n-play easy solution to validate email addresses and improve marketing ROI. Abstract provides many tutorials to help you easily integrate their APIs into your code.
For example, you can learn how to use the Abstract Email Validation API to clean your mailing list of bogus addresses in this tutorial.
7. Alternate Solution to Request Client IP Address in Node.js - Use the Request IP NPM Module
If you want to use an open source solution to find the IP address in Node.js, then you can try the Request IP NPM module.
First, Install Express With the Below Command
Install the Request IP Module With the Following Command
Write the following JavaScript code in a server.js file
We now launch the Node JS app in the example above with the following command:
If we now point to localhost:3000 in our browser, we see our IP address.
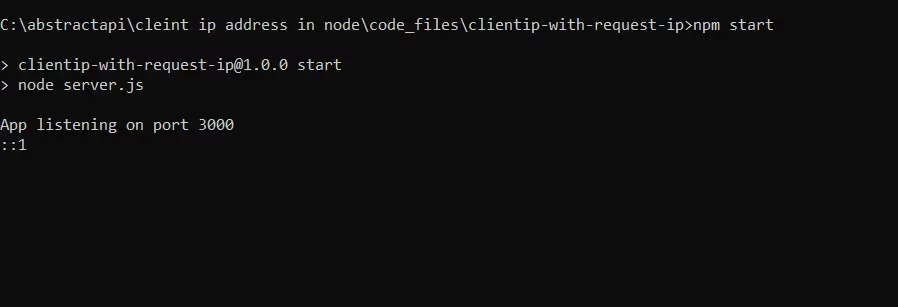
The Request IP Node module covers all the edge cases for the different headers and objects where the client's IP address may be present. For example, it will look through the following for the IP address:
Specific Headers:
- X-Client-IP
- X-Forwarded-For
- CF-Connecting-IP
- Fastly-Client-IP
- True-Client-IP
- X-Real-IP
- X-Cluster-Client-IP
- X-Real-IP
- X-Cluster-Client-IP
Node JS and Express Objects:
req.connection.remoteAddress (for older Node JS versions)
req.socket.remoteAddress
So, this method is a one-shot powerful solution that covers most use cases.
FAQs
How do I find the IP address in Node JS?
You should look up the request.socket.remoteAddress property with vanilla Node JS or the req.ip property if you use Express on top of Node. Also, you can find the IP in request headers like the X-Client-IP or the X-Forward-For (XFF). If you use a CDN service like Cloudflare or Fastly to improve performance, then you should check their proprietary headers.
What is a proxy, and why should I use a proxy network?
A proxy network between the client and your server improves site performance and security with the power of load-balancing, caching, and edge computing.
How to find the original request's IP address if I use proxies?
You can find the original request's IP in the X-Forwarded-For (XFF) header or custom headers such as the CF-Connecting-IP for Cloudfare, or the X-Real-IP for Nginx proxies.