Validate Email Addresses With Django
You can do Django email validation using a few different methods. Django provides out-of-the-box validation through the is_valid method and through its validators attributes. These methods can be used alone to do basic validation without any additional work. Django also gives you the option to define your own custom validation functions if you need more control over your validation.
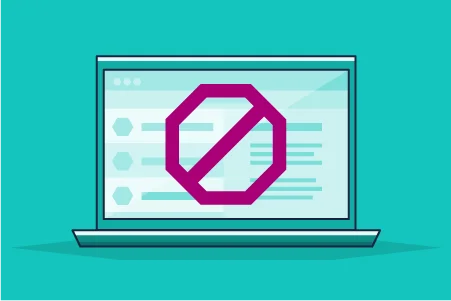
We'll cover all three of these methods in this tutorial. First, let's learn about the is_valid function.
Validate Email Address With is_valid
The is_valid() method is provided by Django to validate all the data in a form against known Python datatypes. A Django form is made of predefined Python classes like CharField and EmailField, meaning Django can check that the input of each field is formatted as expected by comparing what's in the field to the expected datatype.
Assume you have a Sign-Up form that requires an email address and first name from your user. The form is likely defined inside a modes.py file like this:
And you will have an HTML template for the form that looks like this:
Add the is_valid() call to the form logic inside a views.py file to validate the data before sending it to your back end.
If you input an invalid email address and try to submit the form, the is_valid function performs email validation on the string to check the format of the email address. If it is found to be wrong, your HTML error message will display.
Validate Email Address With Validators
The is_valid function can be used to validate all the data in a form at once. If you'd rather just check a single form field for a valid e-mail address, you can use HTML validators.
Validators are attributes that exist on HTML5 forms. There are five basic types of validators.
- required: Specifies whether a form field must be filled in before the form can be submitted.
- minlength and maxlength: Specifies the minimum and maximum length of strings.
- min and max: Specifies the minimum and maximum values of numbers.
- type: Specifies whether the data needs to be a number, an email address, or some other specific preset type.
- pattern: Specifies a regular expression that defines a pattern the entered data needs to follow.
These are always available on HTML input fields. They can also be accessed through Django using the validators field and used to do model validation.
Add some validators to the form fields inside your model file.
This makes the first_name field and the email field required using the required attribute. It also uses a built-in email validation method to run a validation function against email addresses typed into the email field. The EmailValidator function bases its validation on the type attribute and a Regex pattern that matches valid email addresses.
Validate Email Address With Custom Validators
The validators attribute also allows custom validation functions. Instead of using a generic Django form validation method, you can define your own function and pass that to the validators array instead.
Create an email validation function that uses a third-party validation service to validate emails. A third-party validation service might be a validation library or an API endpoint that accepts email addresses as input and returns a JSON validation response.
In this tutorial, we'll use the AbstractAPI Free Email Validator to validate an email before you submit your form data.
Acquire an API Key
To use the API, you'll need an API key. Go to the AbstractAPI Free Email Validation API homepage and click "Get Started."
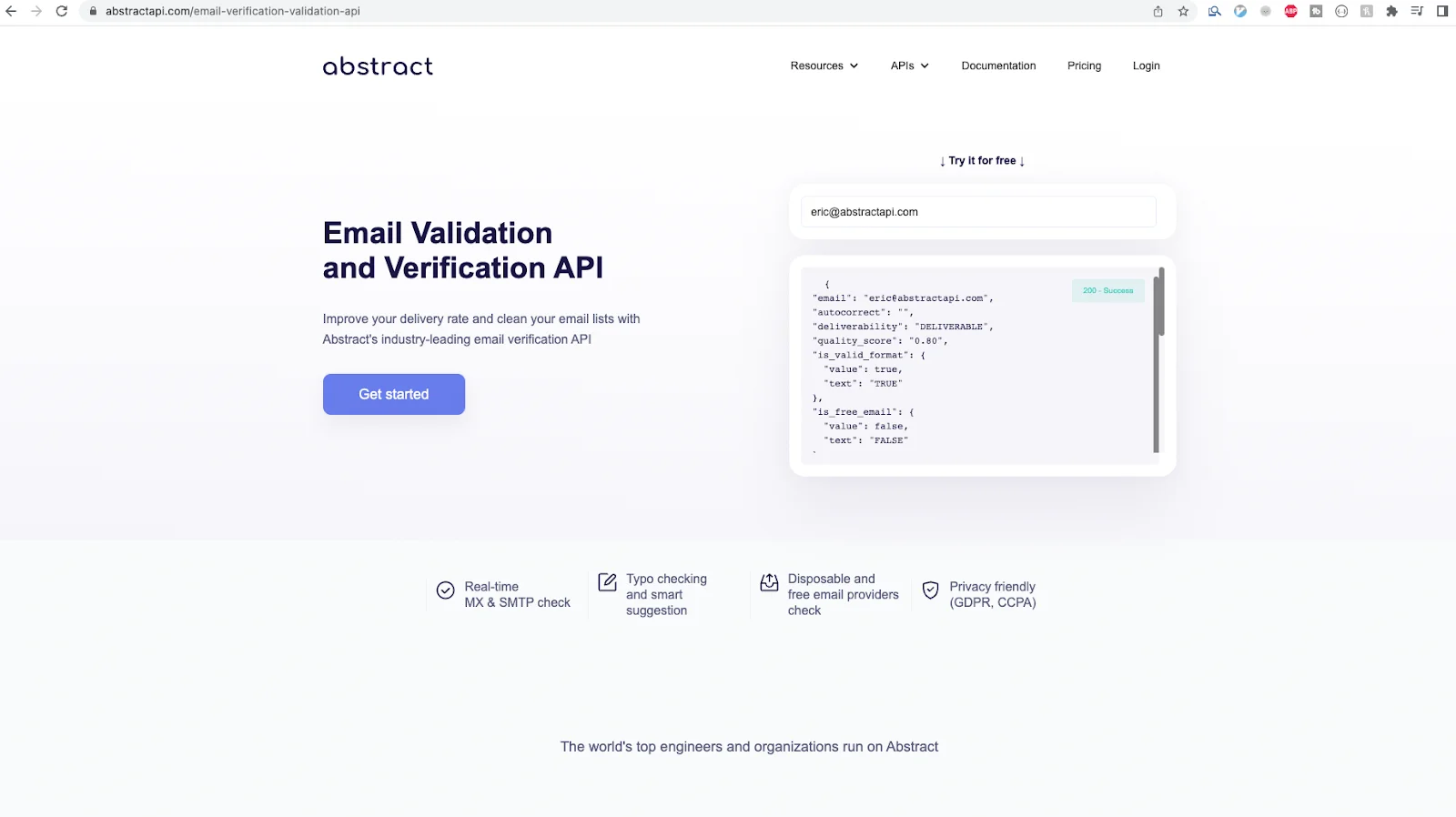
If you've never used AbstractAPI before, you'll need to sign up for a free account using an email address and password. When you’ve signed in, you’ll land on the API’s dashboard, where you’ll see your API key.
Send a Validation Request to the API
Use the Python requests module to send a validation request. You'll need the API key and the AbstractAPI URL, which you can also find on your API dashboard.

Write a function called validate_email that accepts an email as an argument and sends it to the API for validation. For now, just print the response.
Examine the API Response
Use the Django shell to call the function. Pass a test email, and look at what you get back. It should look something like this.
The benefit of using a service like AbstractAPI to validate emails is that it validates the email based on many more categories than a simple string match. It checks MX records, domain validity, known blacklists, role-based accounts, etc.
There are many fields in the JSON response that you can use to determine if the email is valid or not. Write a function that looks at all of the fields and returns True if the email is valid, and False if not.
Add the is_valid_email call as a step in your validate_email function.
Finally, pass the validate_email function as a validator to the validators array on the email field in your models file.
When a user inputs an invalid email into the signup form in the browser, the validator function sends a request to the API, examines the response, and raises a validation error if the email is invalid.
