Getting Started With React Native
React Native is a framework for building cross-platform mobile apps using React JS code. It’s easy to get started, and there are many libraries available to help you do common things, including many of the same libraries you would use to build a regular React app. Using React Native to build your Android app means you don't have to use Android Studio or import Android specific code and libraries.
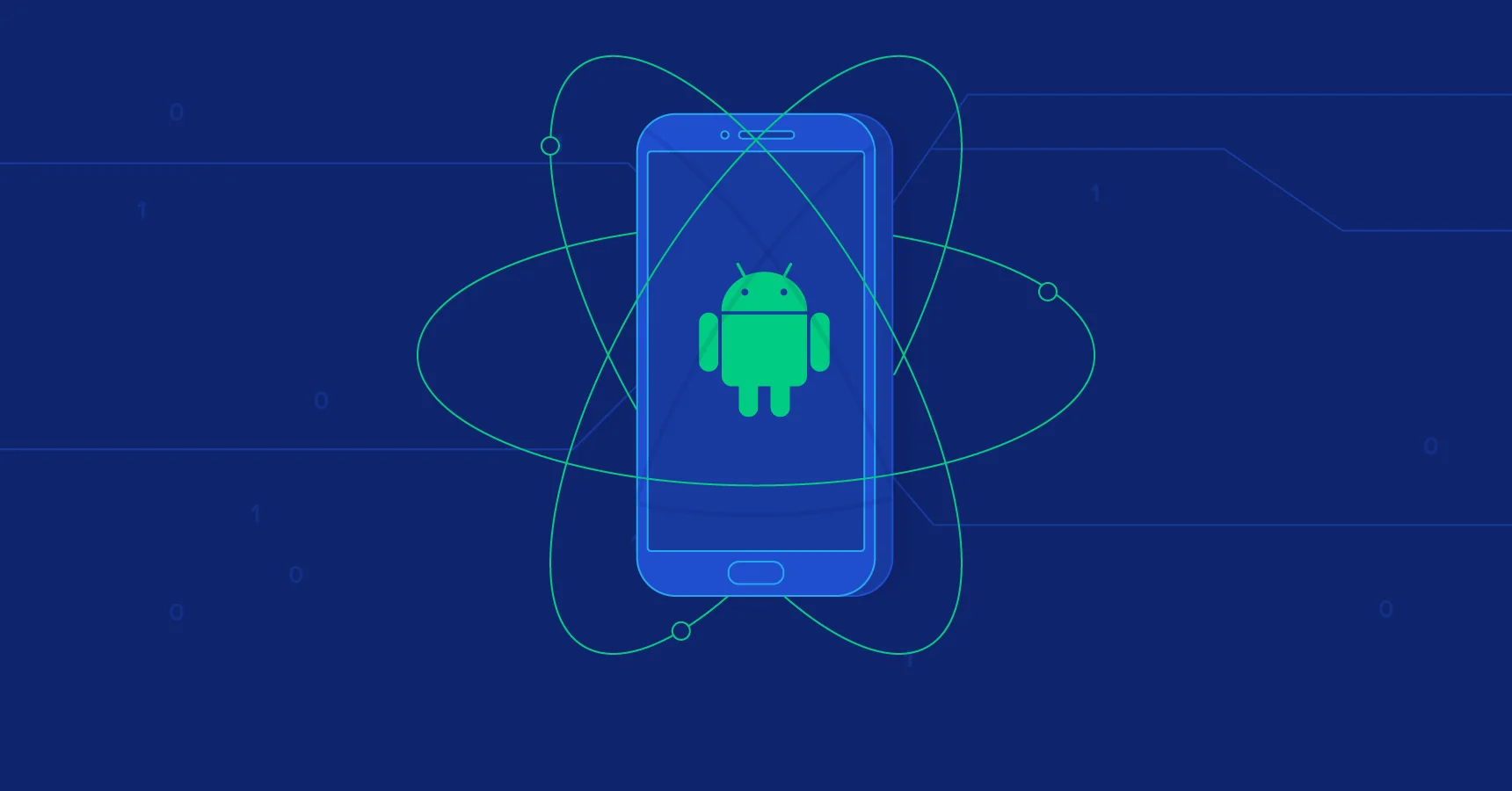
The easiest way to get started with React Native is to use Expo. Expo is sort of like Create React App for React Native. It provides everything you need to get a mobile development environment up and running quickly.

Install the Expo CLI
First, install the Expo CLI.
Download Expo Go
Next, download the Expo Go app for your mobile device. You can run Expo Go on an Android or iOS device. Make sure you download the Android version of Expo Go for this tutorial.
Expo Go at the Android Play Store
Spin Up Your Expo App
For more detail on Expo, getting started, and what else you can do with Expo, check out the Expo docs.
To check that things are working, start up the app using the CLI.
This command tells Expo to start the Metro bundler, which compiles and bundles the code and serves it to the Expo Go app. Once it starts successfully, you should see a QR code in your console. Scan it or just open up the Expo Go app on your phone to connect to the Metro bundler and view the app on your mobile device.

That’s all we need to do to get our new React Native app up and running! No need for Android studio or to import Android-specific code.
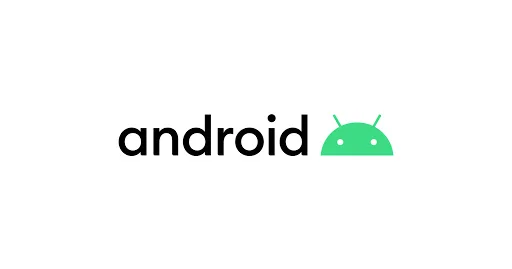
Getting Started With the Geolocation API
Next, let’s take a look at the API we’ll be using to do our geolocation. We’re going to use the AbstractAPI Free Geolocation API. This API takes a GET request and returns a JSON object with information about the IP address of the device that made the request. You can also send a POST request with a different IP address as the data, and the API will return information about that IP address instead.
Acquire an API Key
First, we need to get an API key to authenticate us to the API. Go to the API documentation page. Click “Get Started”
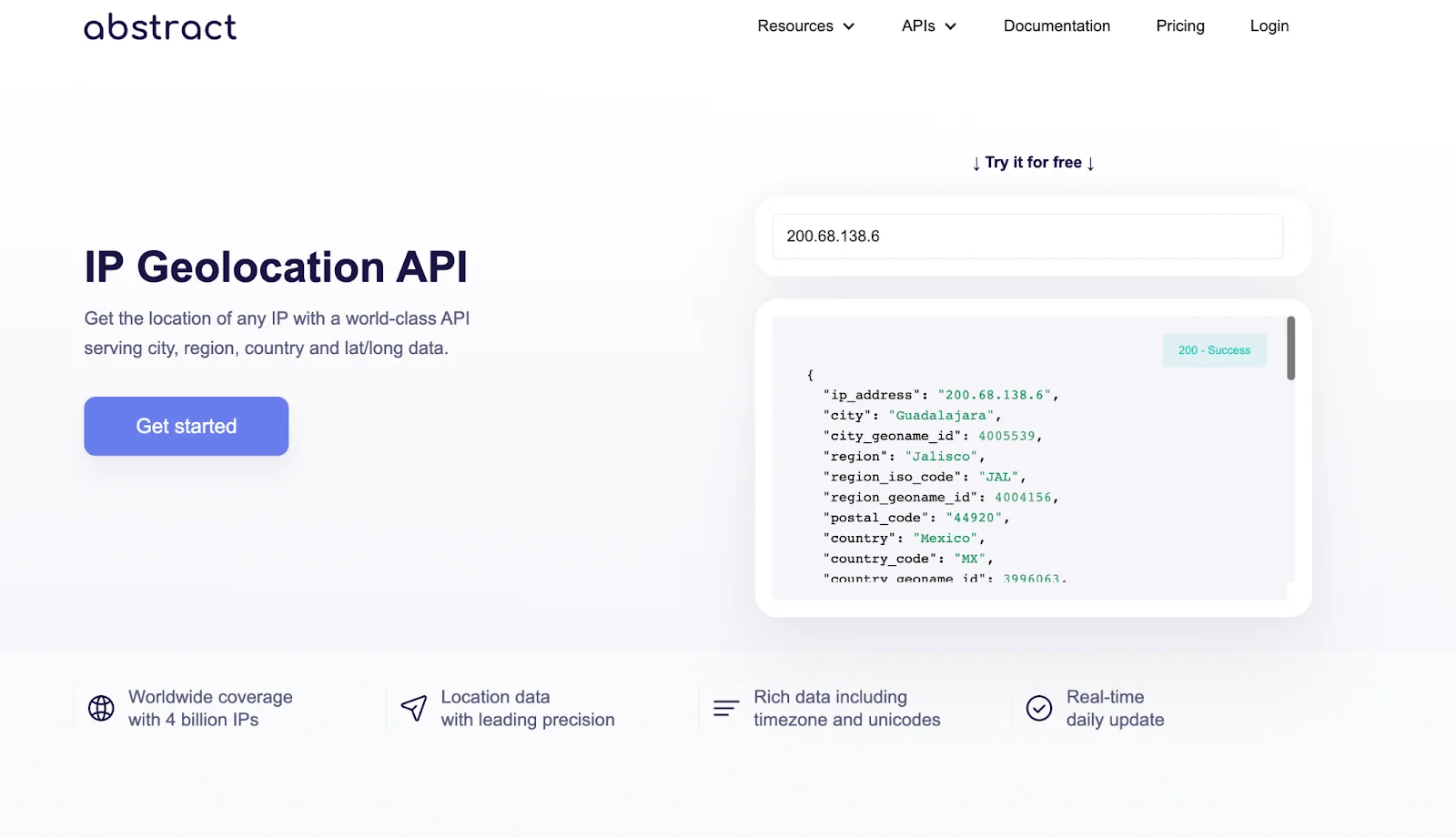
You will be asked to create an account using an email address and password. Don’t worry, the API is totally free and they do not send you any marketing emails or spam.
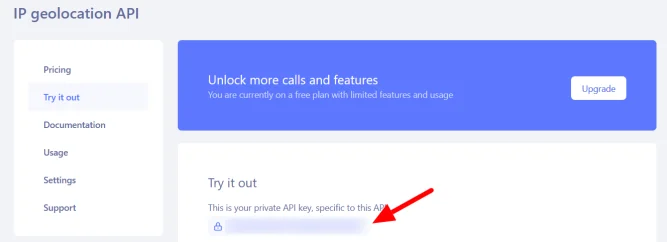
Once you’ve signed up and logged in, you’ll land on the API’s homepage where you should see options for documentation, pricing, and support. You’ll also see your API key.
Get Geolocation Information In Your Android App
We’re going to write a very basic app that displays a user’s location - specifically the city, state, and country information - using geolocation information from their IP address. The API will return an accurate location: IP lookup returns location data with about 75% accuracy.

Create the Display Component
First, delete everything inside App.js and replace it with the following code
The welcomeMessage variable will display a text string to the user telling them what city and country they are currently located in. Let’s write the function that will send the geolocation request to the API to get this information.
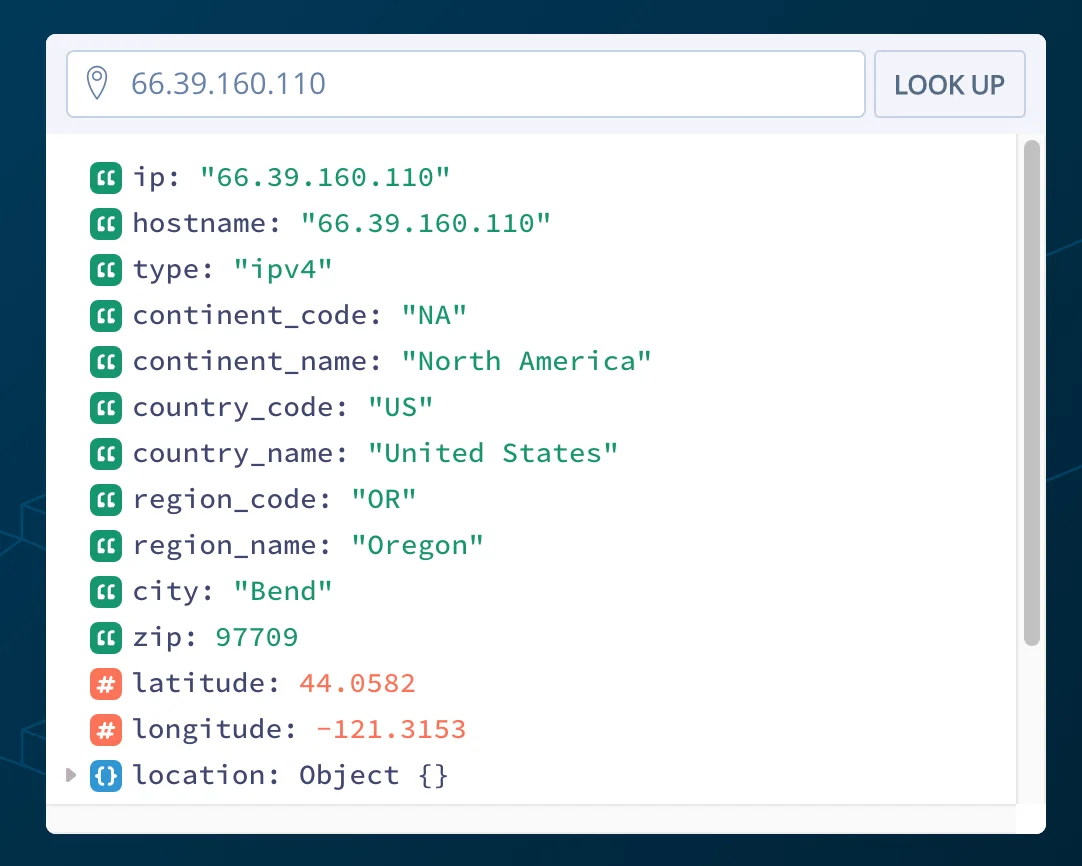
Write the API Request Function
Grab the API URL and your API key from the AbstractAPI Geolocation dashboard and create two variables for them in the imports section of your App component.
We’ll use fetch to make the API request, which is included as a global in React Native. Write a function called getGelocationData inside your main App component. The function should send a GET request using the apiURL variable, including the apiKey variable as a parameter. For now, just log the response to the console.

Write the function inside your main App component.
We want to call this function as soon as the app launches, so let’s put a call to this function inside componentDidMount.
When the app launches, the request will go out and the API will return a location object that looks like this:
(Note: identifying information has been redacted to retain privacy of the author.)
Display the API Data to the User
From this JSON object, we need to pull out the country and city fields and interpolate them into a string to create our welcomeText.
Replace the console.log statement inside your getGeolocationData function with this logic and then use setWelcomeText to update the value of the welcomeText variable in state.
Return to the app and reload. You should see your welcome message rendered.
Conclusion
This is a very basic geolocation app that uses a device’s IP address to display the user’s current location data as a text string. You could easily use more of the location data to do more complicated things: for example, using the lat and long points returned by the API to plot the user’s location on a map using the Google Maps API. Latitude and longitude points will give you a more accurate location than simply city and state.

The next step in improving our basic app would be to add some error handling in case the network request to the API fails. We should also add some additional UI information that tells the user when things are loading, such as network requests.
FAQs
How does geolocation work on Android?
Android smartphones have AGPS (Assisted GPS) chips installed. Assisted GPS uses network towers and WIFI access points to determine the nearby area, which helps the GPS satellites get a location fix on the Android device and display location data.
How do I get geolocation data on Android?
If you are a developer who wants to use location data to improve the app experience for your users, the easiest way to go about acquiring location data is to use the device’s IP address to look up the device location. You can get geolocation information about a specific IP address simply by making a GET request to a third-party service or geolocation API.
If you are a user who wants to view location data on your Android device, open your App drawer and type “Compass.” This will open the compass app that comes pre-installed on all Android devices. It should display your GPS coordinates. You may need to grant location access to the app in order for it to work.
How do I enable geolocation on Android?
To enable geolocation services for a particular app on your Android device, follow these steps:
- Open Settings
- Tap Location
- Select App Access to Location
- From here, you can choose which apps have access to location services and which do not.