Implementing Email Verification: Step-by-Step
Implementing email verification in your Android application is a crucial step in enhancing user authentication, ensuring data accuracy, and improving account security. Here’s what you need to know:
Firebase Authentication
Firebase Authentication provides a straightforward and secure way to add email verification functionality to your Android app. Here's how you can implement it:
Integrate Firebase with Your Android Project: Visit the Firebase console, create a new project (or use an existing one), and add your Android app to the project. Follow the instructions to download the google-services.json file and add it to your app module root directory. Add Firebase SDK dependencies to your build.gradle files.
Enable Email/Password Provider: In the Firebase console, navigate to the Authentication section, go to the Sign-in method tab, and enable the Email/Password provider.
Implement Sign-Up and Sign-In Functionality: Create a user interface for users to sign up or sign in with their email password. Use the FirebaseAuth class to create a new user with email and user password on sign-up. On sign-in, authenticate the user with their email and password.
Send Verification Email: After a user signs up, use the FirebaseUser object to send a verification email.
Check Email Verification: Before allowing the user to proceed, check if the email has been verified.
Custom Email Verification
For developers who prefer more control over the email verification process or need to use a service other than Firebase, implementing a custom email verification system might be the way to go. This process typically involves:
Sending a Verification Email: When a user signs up, generate a unique verification token and associate it with the user's account. Send an email containing a verification link with the token as a parameter.
Verifying the Email: Create an endpoint in your backend to handle verification requests. When a user clicks the verification link, extract the token from the URL and verify it against the stored token. If the tokens match, mark the email as verified in your database.
Securing Your Verification Process: Ensure the verification token is securely generated and stored. Consider token expiration times to enhance security.
Real-Time Verification
Real-time email verification checks the validity of an email address as soon as it is entered, before the user completes the sign-up process. This approach requires integrating with a real-time email verification service. Here's a basic approach:
Choose a Real-Time Verification Service: Select a reputable email verification service that offers real-time API access.
Integrate with Your Sign-Up Process: When a user enters their email address, make an API call to the verification service to check the validity of the email address. Based on the response, you can immediately inform the user whether the email address is valid or not, even before they complete the sign-up process.
Proceed with Standard Email Verification: After validating the email address in real-time, proceed with the standard email verification process (using Firebase Authentication or a custom solution) to ensure the user owns the email address.
Comparison Table of Firebase Authentication vs. Custom Verification Methods
Code Examples and Best Practices
Implementing email verification in your Android application involves more than just the basic setup; following best practices and understanding the nuances of the code can make your implementation more effective and secure.
Firebase Email Verification Code Example
Firebase Authentication simplifies the process of sending a verification email. The following code snippet demonstrates how to verify your email and complete the sign up with Firebase in your Android application. The following example utilizes the Java coding language:

Best Practices
Always check if the `FirebaseUser` object is not null to avoid `NullPointerException`.
Inform the user that a verification email has been sent and guide them to check their inbox.
Handle failure scenarios gracefully, such as by informing the user of the issue and potentially retrying the operation.
Custom Verification Process
Implementing a custom email verification system gives you more control but requires handling more aspects, such as token generation, storage, and verification.
Token Generation and Email Sending
Generate a unique, secure token for each user upon registration. Store this token in your database, associated with the user's account and a timestamp for expiration. Send an email to the user's email address with a link containing the token.
Email Verification Endpoint
Create an endpoint in your backend to handle email verification requests. This endpoint should:
Extract the token from the request. Look up the token in your database and verify it against the user's account. Check if the token has expired based on the stored timestamp. Mark the user's email as verified if everything checks out.
Real-Time Feedback Implementation
Real-time feedback on email validity can significantly enhance user experience by catching mistakes early. Here's how you can integrate it with a third-party API:
Choosing a Service
Select an email verification service that offers real-time API access. Many services provide detailed information about the email address, such as validity, domain existence, and whether it's a disposable email address.
Integration
When the user enters an email address (e.g., loses focus on the email input field), it makes an API call to the verification service. Parse the API response to determine the email address's validity. Provide immediate feedback to the user based on the response, such as a green checkmark for a valid email or a warning message for invalid ones.
Best Practices
Throttle requests to the real-time verification service to avoid overwhelming the service and to reduce costs. Ensure privacy by handling email addresses securely and in compliance with data protection regulations (e.g., GDPR). Use this feature to enhance user experience, not as a sole method for email verification. Follow up with a standard email verification process to confirm ownership.
Checklist for Implementing Email Verification
- Choose Your Method: Decide between using a service like Firebase Authentication or creating a custom verification system.
- Set Up Your Backend: Ensure your backend supports email sending functionalities and stores verification status.
- Generate Verification Tokens: For custom methods, generate a unique and secure token for each verification attempt.
- Send Verification Emails: Use a reliable email service provider to send emails containing verification links or codes.
- Implement Email Validation: Validate email formats before sending emails to reduce errors.
- Handle Verification Links or Codes: Set up a mechanism to verify the token or code when the user interacts with the email.
- Update Verification Status: Once verified, update the user’s status in your database
- Resend Functionality: Provide users the option to resend the verification email if needed.
- Feedback to Users: Communicate clearly with the user throughout the process, especially if verification is pending or successful.
- Test Thoroughly: Ensure the system works under various scenarios, including invalid emails, expired tokens, and repeated requests.
How to Include AbstractAPI’s Email Validation Into an Android App
To include Abstract's Email Validation API into an Android application, you'll be integrating a powerful tool that can significantly enhance the real-time email verification process, providing immediate feedback on the validity of email addresses input by users. Here’s a comprehensive guide on how to add Abstract's email validation API into your Android app:
1. Sign Up and Obtain API Key
First, visit Abstract's Email Verification API page. Sign up for an account if you haven't already, and obtain your unique API key. This key is essential for making requests to the API.
2. Add Internet Permission
Your Android app needs permission to access the internet. Ensure your AndroidManifest.xml includes the following permission:
3. Implementing the API Call
Use Android's HttpURLConnection or a third-party library like Retrofit or OkHttp to make a GET request to the API. Here's a basic example using HttpURLConnection:
Note: Replace "YOUR_API_KEY" with your actual API key. It's also recommended to move the API key and endpoint to a secure location or configuration file.
Parsing the Response
Abstract's API returns a JSON response that contains various fields about the email address validation. You'll need to parse this JSON to retrieve the information you need, such as validity, deliverability, and quality score.
Use Android's JSONObject class or a library like Gson to parse the JSON response.
Updating the UI Based on Validation
Once you have the validation result, update your app's UI accordingly. For instance, if the API indicates that an email is invalid, you could display an error message near the email input field.
Ensure that UI updates are performed on the main thread.
Case Studies
From Medium.com:
1. E-commerce
Email verification tools have had a big impact on the rapidly expanding e-commerce industry. An extensive case study highlights how email verification changed an e-commerce company's email marketing campaigns. The company is experiencing rapid growth.
Deliverability rates increased significantly for this company as a result of their diligent efforts to find and remove invalid and inactive email addresses from their database. Higher open and click-through rates followed by a notable increase in overall revenue and campaign success were the results of this.
2. Nonprofit
Email marketing is a key tool used by nonprofits to build relationships with supporters and donors. An engaging case study from the nonprofit sector highlights how email verification tools have revolutionized their email marketing efforts.
They were able to save money by keeping a short, superior email list and saw a significant increase in engagement metrics. This nonprofit saw an increase in participation, donations, and the development of a stronger network of supporters by sending emails only to those who were truly interested in their cause.
Author Credentials
Ujjwal Maddy, an Android developer on Quora, spoke about his experience and contribution in the sector:
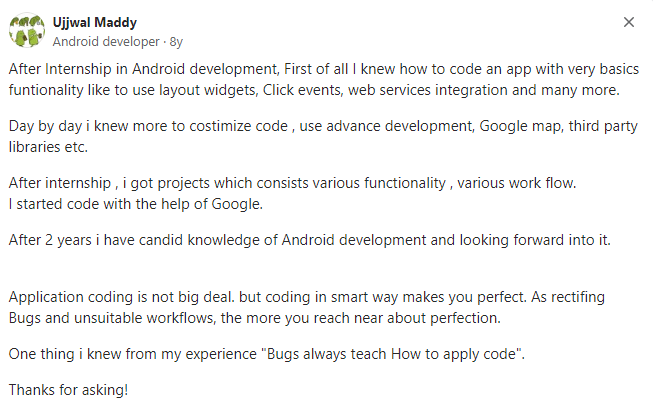
Common Questions About Android Email Verification
Addressing common questions about email verification in Android apps can help clarify the decision-making process for developers, especially when considering Firebase versus custom verification methods, ensuring email deliverability, and understanding the legal considerations for email collection. Here’s a breakdown of these common questions:
Firebase vs. Custom Verification: Which to Choose?
What are the advantages of using Firebase for email verification?
Ease of Implementation: Firebase Authentication simplifies the process, providing out-of-the-box support for email verification without the need for a separate backend.
Security: Firebase Authentication handles security concerns, such as secure storage of user credentials and tokens.
Scalability: Being a part of Google’s ecosystem, it easily scales with your app’s growth.
Why might someone opt for a custom verification system?
Flexibility: A custom solution offers more control over the verification process and user experience.
Customization: Allows for tailored verification flows, such as multi-step verification or integrating additional verification methods.
Independence: Avoids dependency on third-party services, which can be crucial for apps with specific compliance requirements.
Ensuring Email Deliverability
How can you improve the chances of your verification emails being delivered?
Use a Reputable Email Sending Service: Services like SendGrid, Amazon SES, and Mailgun are optimized for deliverability.
Authenticate Your Emails: Implement SPF, DKIM, and DMARC records to verify your domain and improve email authenticity.
Maintain a Positive Sender Reputation: Regularly monitor your email bounce rates and avoid spammy content to keep your sender reputation high.
Clear Call-to-Action: Ensure the verification email is clear and includes an easy-to-find verification link or code.
Legal Considerations for Email Collection
What legal aspects must be considered when collecting emails?
Consent: Under laws like GDPR in Europe and CAN-SPAM Act in the USA, it’s crucial to obtain explicit consent from users before sending them emails, especially for marketing purposes.
Privacy Policy: Your app should have a privacy policy that details how you collect, use, and store user data, including email addresses.
Data Minimization: Collect only the email data necessary for your app’s functionality, respecting user privacy and legal considerations email collection requirements.
Right to Withdraw Consent: Users should be able to opt out of emails easily, and you must honor these requests promptly.
Conclusion
Strengthening your Android application to incorporate email verification is crucial. Although there are a number of ways to accomplish this, such as using Firebase and creating custom solutions, using a dependable service like Abstract's Email Validation API can greatly expedite the procedure.
By verifying email addresses in advance, Abstract API provides real-time email verification, lowering the possibility of user input errors and guaranteeing that verification emails reach their intended recipients. For a reliable answer to your email verification requirements, take a look at Abstract's Email Validation API.
FAQs
How to handle users who do not receive the verification email?
Provide a feature to resend the verification email and suggest checking the spam or junk folder. Ensure clear communication on expected wait times and offer customer support contact information for unresolved issues.
Can email verification be bypassed for testing purposes?
Yes, for testing, you can implement a bypass mechanism by using a development flag in your code that skips the verification process or automatically verifies certain test accounts.
What are the common issues faced during email verification implementation?
Email verification challenges such as chat being marked as spam often stem from poor sender reputation or lack of proper email authentication. Additionally, delayed delivery might result from throttling by email service providers or network issues. Ensuring that email addresses are correctly formatted is also crucial, necessitating robust validation measures to prevent rejections.