Phone Number Validation Check With Yup
Yup phone validation is easy with yup-phone-light. The yup-phone-lite package adds a phone number method to the basic Yup String type. This method takes the country of the phone number we are validating for, and an error message to show the user if validation fails. We can also chain the Yup String required() method to show a message if the user leaves the field blank.
Build a PhoneSchema
We can build a basic Yup schema object, using the .phone method as our validation method for a phone field.
Call ValidateSync
When we call phoneschema validateSync() on the schema and pass it the phone number from our input, Yup will validate the provided number against the schema. The phoneschema validateSync method does syncronous validation, as opposed to the regular Yup validate method, which is async.
When you call phoneschema validateSync in your submit handler, Yup will validate the schema and raise errors, which you can catch and display to your user.
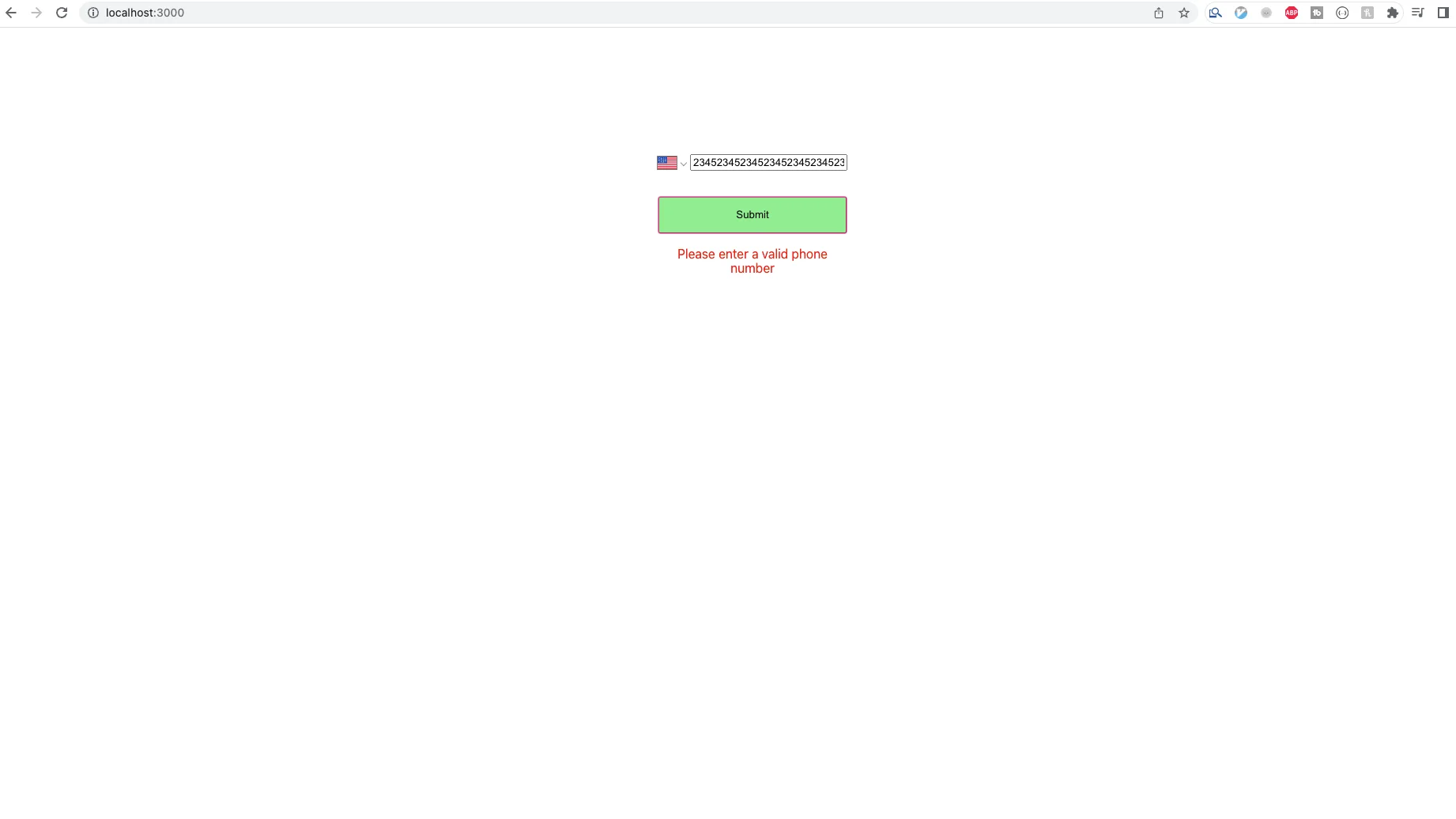
Alternatives to Yup
Another way to validate a phone number is using a dedicated phone number validation API like AbstractAPI’s Free Phone Number Validation API. This API checks the provided phone number against a database of numbers.
AbstractAPI ensures not only that the number is real and doesn't contain unexpected characters, but also gives you information about the number, like the specific location of the number, the carrier, and a few other useful things.
Conclusion
We’ve set up a basic phone input and explored two different phone number validation methods. There are many ways this can be improved. We need better styling on the input, and we also need to include a loading spinner for any asynchronous requests.
FAQs
How Can I Validate My Mobile Number In Yup?
Unfortunately, Yup does not provide out-of-the-box phone number validation as it does for email validation. However, you can extend Yup with yup-phone-lite, which adds a phone number method to the basic Yup String. You could also use yup-phone which is a Yup validator using Google libphonenumber.
How Do I Do a Phone Number Validation Check?
There are three steps is fully validating a user’s phone number. The first is collecting the user's number in some form field. Next, you must validate that the number is well-formatted and does not contain unexpected characters. Finally, you should verify that the user has access to the number by sending an SMS.
What Is Yup?
Yup is a JavaScript validation package that can be downloaded using a package downloader such as npm or yarn. Once added to your project, you can use it to create simple object schemas that can be used to either validate input or cast invalid input to a valid state. The best way to use Yup is to validate emails, phone numbers, passwords, and other fields in your forms.