Basic Phone Number Validation in React JS
The easiest way to do phone number validation in React is using Regex. Regex (regular expressions) allow you to construct a pattern that can be used to match input strings.
There are many ways to write a regular expression for phone number matching. One way is the following:
This will match phone numbers of the following formats:
- 123-456-7890
- (123) 456-7890
- 123 456 7890
- 123.456.7890
- +91 (123) 456-7890
To use this regular expression, you can simply pass it as the pattern attribute to an HTML phone input:
This will allow the HTML5 input to do basic validation for you. If the number entered does not match the pattern, an error message will be raised to the user.
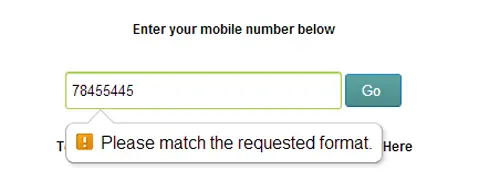
React Hook Form Phone Number Validation
React Hook Form takes a lot of the headache out of building web forms. It also provides built-in validation methods that allow you to easily validate any input field.
IMAGE: react-hook-form-logo
React Hook Form uses uncontrolled inputs and useRef to create a form that can be updated without depending on state. It provides a slew of out-of-the-box methods for form control, validation, and submission.
Let’s take a look at building a simple React component for phone number validation using React Hook Form.
Install Dependencies
Spin up a new app using Create React App using the following commands:
Next, install react-phone-number-input and react-hook-form.
Start the app
This will open a new browser window with the app running at localhost:3000.
Scaffold component
Inside src create a new file called phone-number-input.js alongside app.js and import dependencies.
Next, create a React component called PhoneNumberInput.
Render component
Remove everything between the two <header> tags inside App.js and render the phone number input component instead using the following code:
Check out your browser window at http localhost 3000. You should now see something like this:
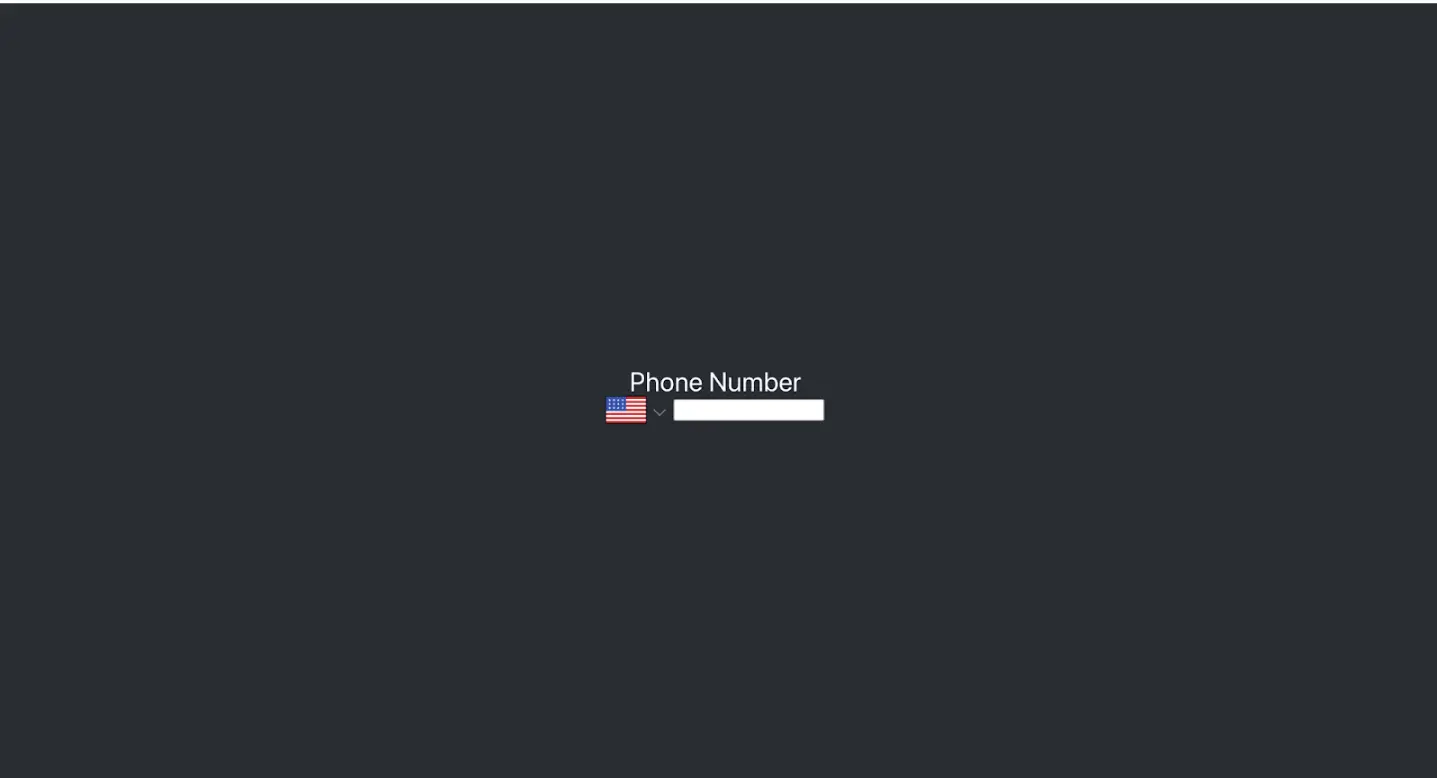
Add component logic
Create the React Hook Form wrapper inside phone-number-input.js using the components and handlers provided by React Hook Form. Write an onSubmit function. For now, this function will just log data to the console.
The useForm hook gives us a handleSubmit function. Pass this to the onSubmit handler of the <form> element. When the user hits the return key or submits the form, this function will be called.
Delete the <p> tags from the component and instead render a form element that includes the <label>, and a <Controller>
Notice that we name our controller “phone-input” because naming it will allow us to access it by name in the errors object later. We pass the control prop from the useHook hook to the <Controller>.
The render field accepts a function that returns a child component. It passes to the child component two values: an onChange handler, and a value. The child component we’re returning is the react-phone-number-input PhoneNumber component.
This means that React Hook Form takes care of updating the value of the form. We don’t write our own initial value, use setState, or write an onChange function.
Add validation
React Hook Form and react-phone-number-input make validation easy. Write a function that uses the isValidPhoneNumber method to validate a provided phone number:
Log the isValid value to the console so you can see what’s going on before you return it. Next, add the function to the validation rules in the React Hook Form Controller component:
The validation function will be called every time the form value updates. Finally, render an error message if the phone number is invalid:
We’re using the errors object provided by the React Hook Form formState. React Hook Form adds any errors that the React component encounters to the errors object under the same name we gave our input.

Mobile Number Validation in React JS
When you’re validating phone numbers in React JS, you are mostly validating mobile numbers. Mobile numbers are what pretty much everyone uses these days, and it is very unlikely you will ever need to validate another type of number.
Keep in mind that when it comes to validating mobile numbers, you should also verify the number by sending an SMS to the number with a validation code. This is a very secure way of validating the mobile number.
SMS validation is beyond the scope of this article, but some good services to look into to handle this part of the process include Twilio, sms-verification on Github, and Google’s SMS verification API.
React Phone Number Validation With an API Key
If you want your phone number validation to be as robust as possible, you should use a phone number validation API in addition to Regex or the built-in validation methods provided by libraries. Let’s take a look at how to use AbstractAPI’s Free Phone Number Validation API to add an extra layer of validation to our existing app.
Get an API key
Navigate to the API documentation page. Click the “Get Started” button. You’ll be taken to a page where you’re asked to input your email and create a password.

Once you log in, you’ll land on the homepage of the Phone Number API, which looks like this:
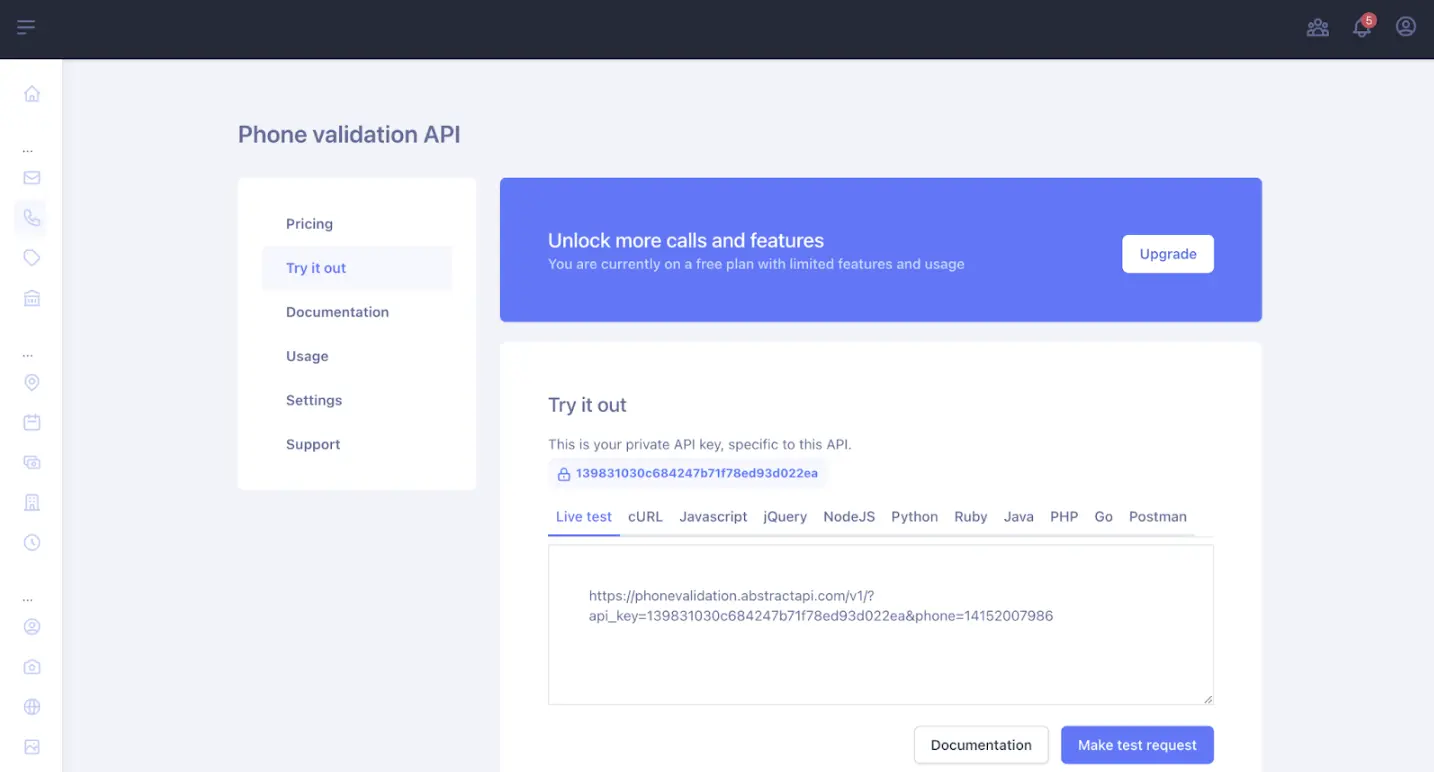
Add API Validation to the Validation Function
First, install axios to send a network request.
Write a function that sends a network request to the API, containing the phone number for validation:
This is now an async function. It will be called every time the React Hook Form updates. However, you won’t need to debounce the function: according to the validate documentation, React Hook Form allows you to pass an async function as a validator.
Use the Response
The response you get back from the API will be a JSON object like this:
We simply pulled the valid boolean value out of this object and used it to determine the validity of the provided phone number.