Method 1: Using the Sockets Module for Internal IPv4 and IPv6 address Retrieval
Retrieve your system's internal IPv4 or IPv6 address using the socket.gethostbyname method, a straightforward solution for local hostname resolution compatible with Windows, Mac, and Linux systems.
IPv4:

- import socket: This line imports the socket module, providing access to the BSD socket interface for various network functions.
- hostname = socket.gethostname(): Here, gethostname() retrieves the current machine's hostname, a label assigned to the device on a local network for identification purposes.
- ipv4_address = socket.gethostbyname(hostname): The gethostbyname() function translates the retrieved hostname into its corresponding IPv4 address, which is the internal address used by the device on the local network.
- try...except blocks: These blocks add error handling to the script. The try block encompasses the operations that might fail, such as hostname resolution. The except blocks catch and handle specific errors. socket.gaierror is caught if there's an issue with address resolution, and a general exception is caught for any other unexpected issues.
- print(f"IPv4 Address for {hostname}: {ipv4_address}"): This line prints the hostname and its resolved IPv4 address. The output is helpful for identifying the internal IP address through which the computer communicates over IPv4-based networks.
This method retrieves the internal network device IP only. It is useful for diagnosing and resolving local network issues, which is critical in a variety of settings, from advanced smart home systems to complex corporate networks. For developers, this approach is invaluable for testing network applications within a LAN, ensuring smooth communication among devices.
IPv6:
IPv6 addresses can be retrieved using the socket.AF_INET6 family within the socket module. This example demonstrates how to obtain an IPv6 address:
- import socket: Imports the socket module, used for network communications, as in the IPv4 version.
- hostname = socket.gethostname(): Retrieves the current machine's hostname, a label for device identification on the network.
- ipv6_address = socket.getaddrinfo(hostname, None, socket.AF_INET6): Fetches the IPv6 address for the hostname. The getaddrinfo function returns IPv6 address details, indicated by socket.AF_INET6.
- try...except blocks: Error handling is added to manage issues during hostname resolution or IPv6 fetching. The try block executes the address retrieval, and the except blocks catch specific errors, including general exceptions for robustness.
- print(f"IPv6 Address: {ipv6_address[0][4][0]}"): Prints the retrieved IPv6 address, located in the first tuple of the getaddrinfo result.
Method 2: Using the Requests Module for External IPv4 IP Retrieval
To determine your public IP address, you can exclusively use Python's requests module. This method is straightforward and solely focused on obtaining your public IP address, using an external service like the ipify API.
This snippet demonstrates how to obtain your true public IP address using Python's requests library and the ipify API:
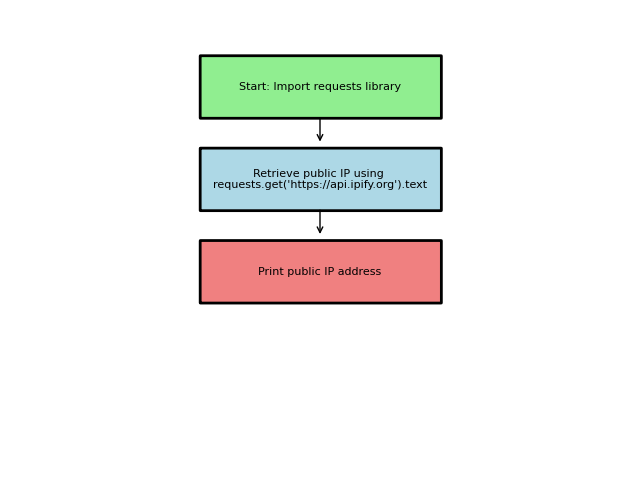
- import requests: This line imports the requests library, essential for making HTTP requests in Python. Install it using pip install requests.
- public_ip = requests.get('https://api.ipify.org').text: Here, a GET request is made to ipify API (https://api.ipify.org), known for its reliable service in providing external IP addresses. The .text attribute is used to extract the public IP from the response.
- print(f"Public IP Address: {public_ip}"): This line prints the retrieved public IP address, with Python’s f-string formatting for clearer output.
- Error Handling: The script includes error handling to manage potential issues like network connectivity problems or service unavailability.
In network management and IT, the ability to retrieve a public IP address is crucial. This method facilitates remote system monitoring, dynamic DNS services, and cybersecurity efforts where identifying a system's public IP is essential for tracking network activities and addressing security concerns.
Method 3: Using an API (AbstractAPI.com)
For a more comprehensive understanding of an IP address, including its geographical location, ISP, and timezone, consider using Abstract API's IP Geolocation service. This method requires obtaining a free API key from Abstract API, which then enables access to a wealth of detailed information about any given IP address. Register and get your IP Geolocation API key.
- import requests: This line imports the requests module, a Python library used for sending HTTP requests. It's essential for interacting with web APIs like AbstractAPI, allowing you to send and receive data over the internet.
- response = requests.get('https://api.abstractapi.com/v1/ipaddress/?api_key=YOUR_API_KEY'): Here, a GET request is sent to AbstractAPI's IP Geolocation service. The URL includes your unique API key (replace <YOUR_API_KEY> with your actual key). This request fetches detailed information about your IP address, including geographical location, ISP, and timezone.
- You can sign up for your free API key at AbstractAPI’s website.
- ip_info = response.json(): Once the request is made, the API responds with data in JSON format. This line uses the .json() method to parse the JSON response into a Python dictionary. It allows for more convenient access and manipulation of the data, such as extracting specific details about the IP address.
- print(ip_info): This line prints the entire response dictionary to the console. It provides a comprehensive view of the data returned by the API, including location data, ISP information, timezone, and more. It's a useful way to quickly verify the data retrieved from AbstractAPI.
- Error Handling: Error handling is implemented to manage potential issues during the API request. The try...except block catches exceptions related to HTTP errors, connection problems, timeouts, etc., ensuring the script can gracefully handle and report any errors."
Handling Multiple Network Interfaces in Python with Netifaces
In environments with multiple network interfaces, such as servers or complex network setups, it's often necessary to identify the IP address of each specific interface. Python's netifaces library offers an efficient solution for this task, allowing you to enumerate and interact with various network interfaces on your system. This method is particularly useful for applications that require knowledge of the network configuration, including interface-specific IP addresses.
First we want to list our network interfaces:
- import netifaces: This line imports the netifaces module into your Python program.
- interfaces = netifaces.interfaces(): Here, we're using netifaces.interfaces() to retrieve a list of all network interfaces available on the system. This function returns a list of interface names, making it easy to iterate over or select a specific network interface for further operations.
- print("Available network interfaces:", interfaces): This line prints out the list of network interfaces obtained in the previous step.
After you have a list of network interfaces we can use the following script to retrieve the device’s IP address:
- Fetching Address Information: The function begins by retrieving all addresses associated with the given interface using netifaces.ifaddresses(interface).
- IPv4 and IPv6 Address Check: It then checks if the interface has IPv4 (netifaces.AF_INET) and/or IPv6 (netifaces.AF_INET6) addresses and stores them in the ip_info dictionary.
- No Address Found: If no IPv4 or IPv6 addresses are found for the interface, the function returns a message indicating this.
- Returning IP Information: If valid IP addresses are found, the ip_info dictionary containing these addresses is returned.
- Specifying the Interface: The interface_name variable is set to 'eth0' (or any other interface name as required). This is the network interface for which the IP address(es) will be retrieved.
- Function Call: get_ip_address(interface_name) is called with the specified interface name. The function returns the IP address information for that interface.
- Output: The script prints out the IP address information. If the specified interface has an IPv4 or IPv6 address, it will be displayed; otherwise, an appropriate message indicating the absence of an IP address or an error will be shown.
Best Practices for IP Address Retrieval in Python Networking
Effective network scripting in Python hinges on choosing the right tools for your needs:
- Internal vs. External IP Retrieval:
- Use socket and netifaces for internal IP address tasks within a local network.
- Use external IP retrieval such as ipify or Abstract API for applications that interact with the wider internet or for remote access.
- IPv4 and IPv6 Compatibility:
- Ensure your network applications support both IPv4 and IPv6, balancing current needs with future-proofing for IPv6's growing adoption.
- Using Public IP Services Carefully:
- Be mindful of privacy and reliability when using external services for public IP retrieval. Consider potential service limitations and security implications.
- Robust Error Handling:
- Implement robust error handling for network operations and test your applications across different network scenarios.
By understanding and applying these practices, you can develop robust and secure network applications in Python that effectively handle both local and internet-facing networking tasks.
FAQ
Q. How do I find my public IP address using Python?
A. You can find your public IP address in Python by making a GET request to an external service like ipify using the requests module.
Q. Can Python retrieve both IPv4 and IPv6 addresses?
A. Yes, Python can retrieve both IPv4 and IPv6 addresses. The socket module can be used for local address retrieval, and netifaces provides a more detailed approach for network interface information.
Q. What libraries are needed to find an IP address in Python?
A. To find IP addresses in Python, the socket library (part of Python's standard library) is used for local IP addresses. For external IP addresses, the requests library is helpful. Additionally, netifaces can be used for detailed network interface information.
Q. How can I handle errors when retrieving IP addresses in Python?
A. Implement try...except blocks for error handling. For instance, when using the requests library for external IP retrieval, catch exceptions like requests.RequestException to handle connectivity issues, timeouts, or HTTP errors.
Q. Is it possible to fetch the geographical location of an IP address in Python?
A. Yes, you can fetch the geographical location of an IP address by using external APIs such as AbstractAPI's IP Geolocation service. This requires sending a request to the API and parsing the response.
Q. How do I choose between internal and external IP address retrieval methods?
A. Choose internal IP address retrieval (using socket or netifaces) for local network configurations and tasks. Opt for external IP retrieval (using services like Abstract API) when you need to know how your system is accessed from the internet, such as for remote server access or network monitoring.
What now?
Now that you've learned how to retrieve your IP address using Python, it's time to put your new skills into practice. Here are some real-world applications where knowing how to find an IP address can be incredibly useful:
Network Diagnostics: Use your Python script to troubleshoot network issues by verifying the IP addresses of your devices within a computer network.
Server Configuration: When setting up servers, especially in a Linux environment, you'll often need to know the server's local IP to configure network settings.
Developing Web Applications: If you're a beginner in Python web development, you can use the IP address to implement user-specific features based on their location or to enhance security by logging IP data.
Internet of Things (IoT): For those working with IoT devices, such as routers or home automation systems, retrieving the local IP can help in device management and network configuration.
Whether you're a software developer, network administrator, or just starting out, understanding IP addresses and how to retrieve them using Python is a valuable skill. The socket module is your gateway to network programming in Python, and with the power of Python 3, you can easily write scripts that interact with your operating system and networking interfaces.
If you're ready to take your skills to the next level, consider diving into topics like TCP/IP, domain name resolution with DNS, or even automating network tasks with Python scripts.
Links
Here are a few resources to help you on your journey.
Python Socket documentation
https://docs.python.org/3/library/socket.html
Requests Module Documentation:
https://pypi.org/project/requests/
AbstractAPI — Free Geolocation API service
https://docs.abstractapi.com/ip-geolocation
Netifaces Module Documentation:
https://0xbharath.github.io/python-network-programming/libraries/netifaces/index.html