Common Regex Patterns for Phone Numbers
Before we dive into how to use regex for phone number pattern matching, it’s important to understand that there is no one correct way to detect phone numbers using regular expressions. In fact, it can be really difficult to come up with a pattern that captures all phone number (especially international phone number) formats.
Here is an example of a commonly used regex pattern for detecting a 10-digit phone number:
Let’s break this down and take a look at each capture group. A capture group groups multiple tokens together and creates a substring for matching back-references.
This capture group contains 3 subsequences. It matches the country code.
Matches the plus sign “+” special character
Matches any digit
Quantifier: match the previous selection 1 or 2 times
Matches whitespace
Next, we have a question mark, another quantifier, which quantifies the whole capture group:
It tells regex to look for either 0 or 1 of the preceding capture group. The next capture group matches the first three numbers in the phone number, plus the parentheses: (123)
There are 5 subsequences:
This is another special character match for the opening parentheses character “(“
Another quantifier specifying there should be either 0 or 1 of the preceding match
Matches digits
Another quantifying specifying there should be 3 digits
Special character match for the closing parentheses character “)” After this capture group we have another quantifier, specifying we should match the previous capture group either 0 or 1 times:
Finally, we have the capture group that matches the final 4 digits in the phone number, plus any included whitespace, and separators like dashes or periods:
This capture group contains 6 subsequences, with 2 of those subsequences containing 3 smaller subsequences.
This is a character set. It tells regex to match any character in the set. It contains 3 subsequences of escaped characters:
Matches whitespace
Matches the dot character
Matches the dash character
Matches any digit
Quantifier: match the previous digit 3 times
The same character set as before
Match any digit
Quantifier: match the previous character four times
Indicates the end of a line
This complete Regex pattern matches phone numbers in the following formats:
It’s a pretty comprehensive pattern that accounts for a large percentage of possible phone numbers - however, it’s not perfect (no regex pattern is.)
Implementing Phone Number Regex in Different Programming Languages
Let’s briefly look at how we might use the previous pattern in a few different languages, namely Javascript, Python, and Java.
JavaScript
To use a regular expression in JavaScript, you need to use the Regular Expression object, which is available on the global scope. It provides a handful of methods for creating and using regex.
This will create a new variable re that holds a regex object with our phone number pattern. To use it, call methods provided by the object using dot notation:
The test, match, search, and replace functions are the most commonly used methods.
Python
In Python, regular expressions are accessed via the re module, which provides a set of functions similar to those available on the JavaScript RegExp object.
Java
In Java, you can import the java.util.regex package to work with regular expressions.
Best Practices in Phone Number Validation
Regex is one part of phone number validation, but it cannot form a complete validation solution on its own. All regex can do it look at the phone number format and tell you whether the string matches an expected set of criteria. It can’t tell you if the number is active, valid, or if it belongs to the user in question.
To answer these questions, you must use an API. A phone validation API such as AbstractAPI’s Free Phone Validation API will tell you whether a phone number is currently in use, plus provides valuable information about the carrier, geographic location, line type, and more.
An API like this accepts a network request containing a phone number for validation, and returns a JSON response object with information about the number:
Additionally, if you plan to do SMS verification, you’ll need to use an API like Twilio to handle sending automated text messages to user phone numbers after they have been validated.
Common Mistakes and How to Avoid Them
The primary mistake people make when using regex to validate telephone numbers is forgetting that a regex pattern usually can’t cover all use cases. There are so many ways to format a phone number that writing an expression to cover all your bases is very difficult.
This is another reason it’s important to back up your validation with an API or other service.
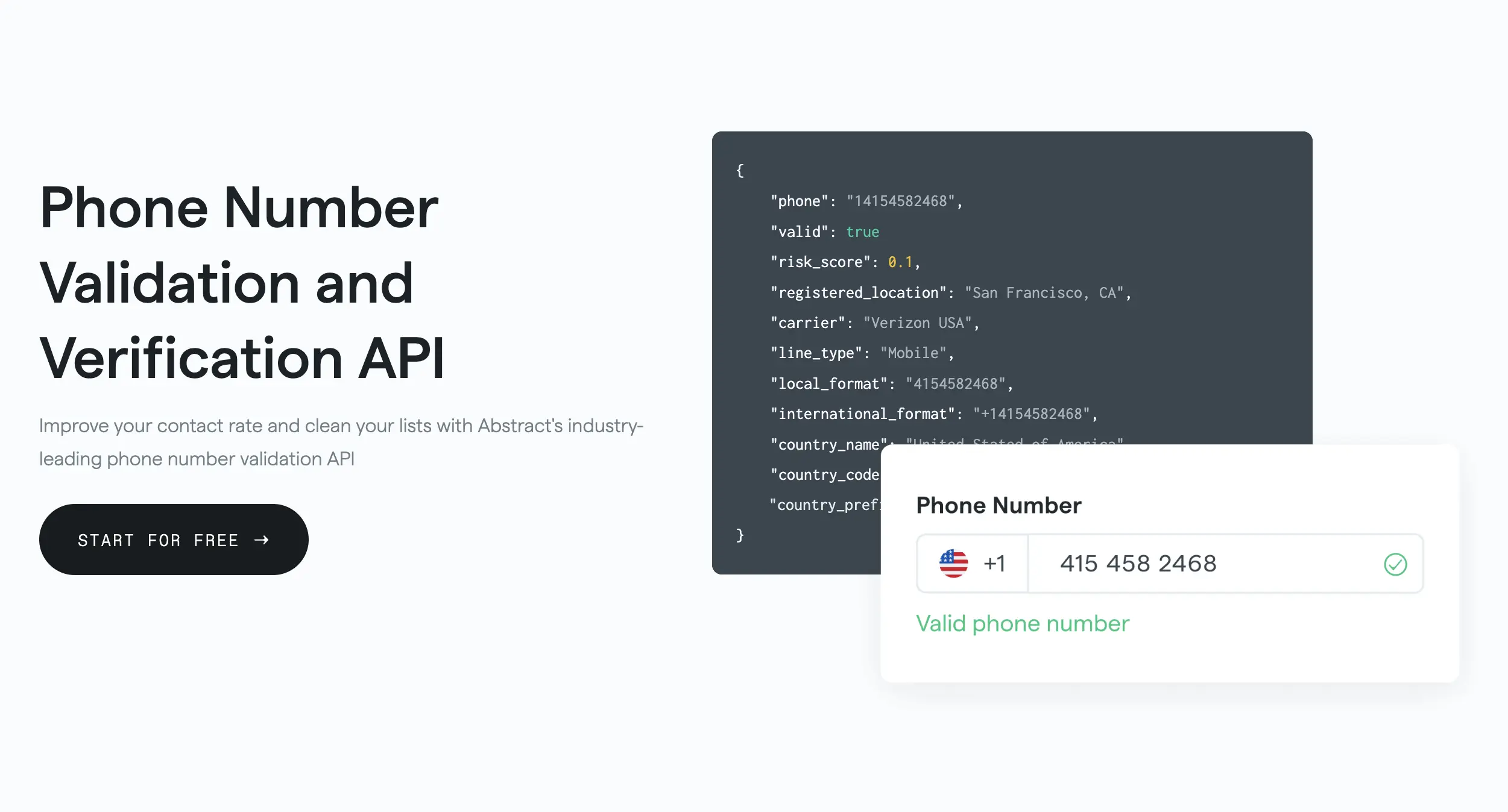
Tools and Resources
Here are some useful tools that can make dealing with regex and phone validation in your applications easier.
- AbstractAPI Free Phone Validation API
- Twilio
- Regexr
- Regex101
- Stack Overflow (online forums and answers to questions)
- Github (to download packages that can be used for phone number validation)
Conclusion
Regex is a complicated beast and few people ever truly master it. Ideally, if you must use regex for phone validation in your app, it’s a good idea to back it up with a trusted online resource like a phone validation API.
FAQs
What is Regex and why is it important for phone number validation?
Regex, or Regular Expressions, is a sequence of characters used for pattern matching in text. It's crucial for phone number validation as it helps identify and correct improperly formatted numbers, ensuring accurate user input.
Can Regex alone suffice for validating phone numbers in large-scale applications?
While Regex is effective in format validation, it's not comprehensive for large-scale applications. It can't verify if a number is active, valid, or belongs to the user, which is where APIs like Abstract's Phone Number Verification API become essential.
How does the Abstract Phone Number Verification API complement Regex validation?
Abstract's API goes beyond format checking by validating whether a phone number is in use, and providing information on the carrier, geographic location, line type, and more. This complements Regex by adding a layer of real-world verification.
Is there a standard Regex pattern for all phone numbers?
There isn't a one-size-fits-all Regex pattern for phone numbers, especially considering international formats. However, common patterns exist, like the one discussed in the article, which cover a significant portion of phone number formats.
Are there programming language-specific ways to implement phone number Regex?
Yes, the implementation of phone number Regex varies across programming languages. The article discusses examples in JavaScript, Python, and Java, each using their language-specific methods and libraries.
What are some common mistakes to avoid in phone number Regex validation?
A common mistake is relying solely on Regex, which may not cover all phone number formats or validate the actual usage of the number. It's crucial to complement Regex with a robust validation service like an API.