In this article, we'll learn how to use an IP address to determine a device's zip code. We'll use React to build a basic frontend app that looks up a device's IP address and displays a zip code for that device's location using reverse geocoding.
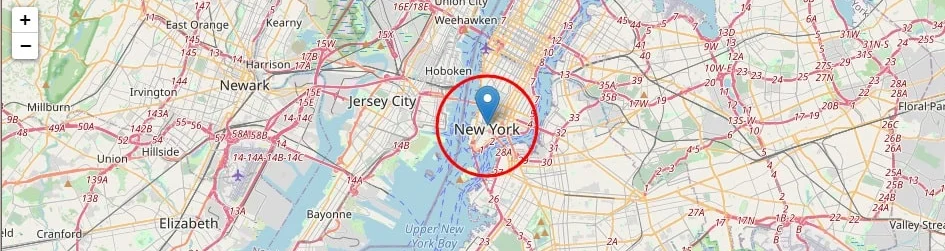
Get Started
Spin up a simple React app using create-react-app.
We don’t need to install any extra packages, because we’re going to use an online third-party API to do the heavy IP geolocation lifting for us.
To check that things are working, start up the app
and visit http://localhost:3000. You should see a page displaying the Create React App starter page.
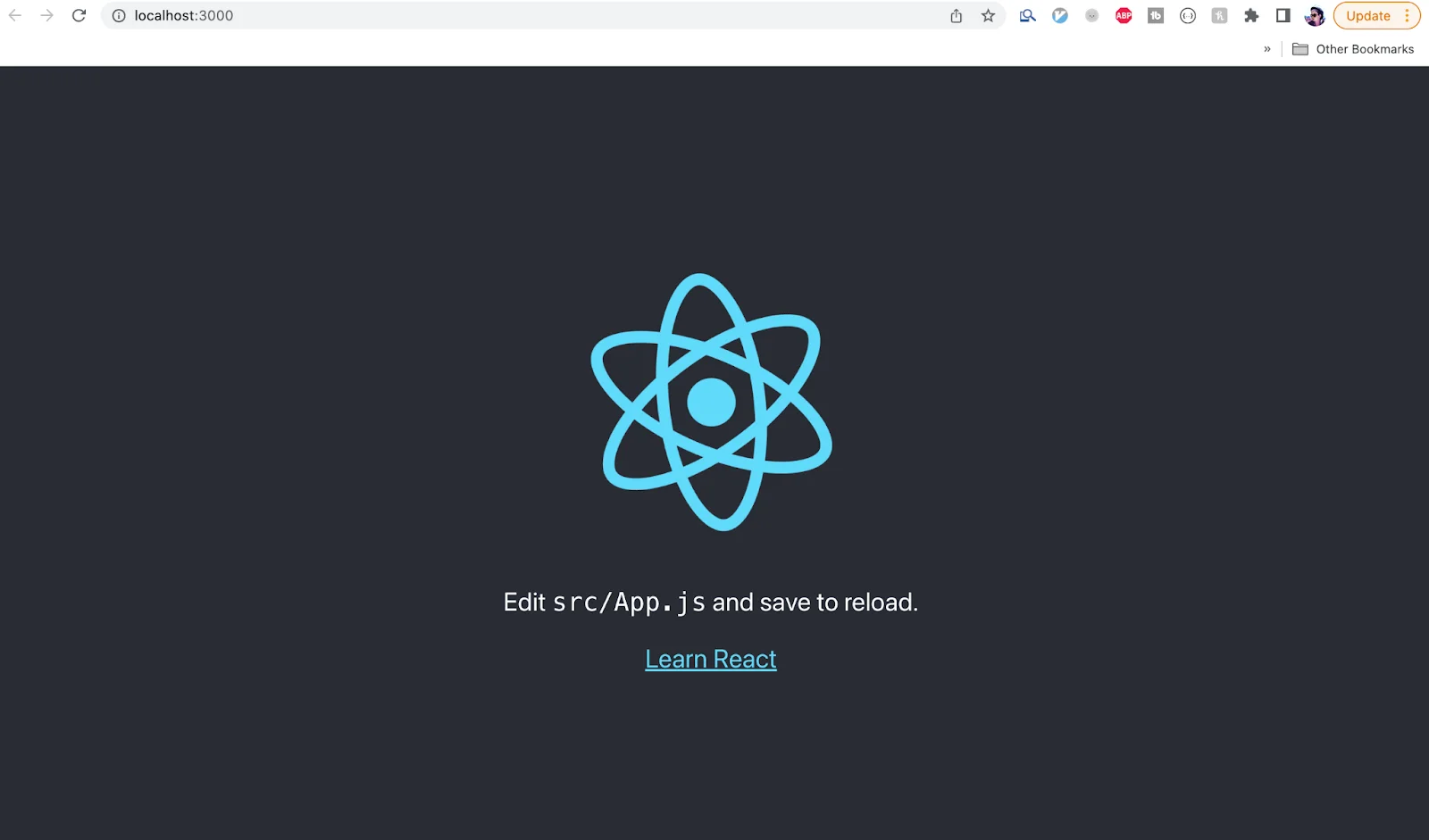
Great! We’re now ready to start getting our geolocation information.
Edit App.js
In the existing App.js file that Create React App made for us, remove the boilerplate code and add the following:
The zipCode variable is where we will store the zip code information we get back from the API when we send it the IP address to analyze.
Get Started With the API
The API we'll be using to get geolocation information is the AbstractAPI Free Geolocation API. The API is free to use and does IP geolocation for you. Just send an HTTP request containing the IP address you want a zip code for, and the API will send back a response with information about that IP address.
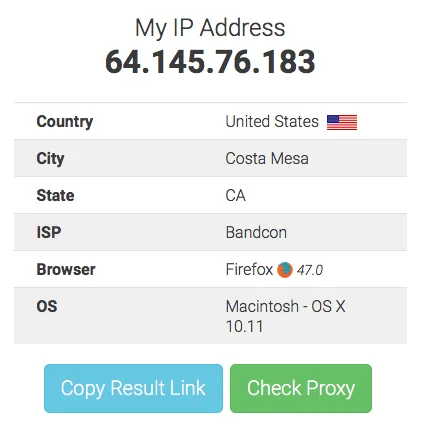
Acquire an API Key
Before you can use the API, you'll need to create a free account and get an API key. Go to the API homepage. You’ll see an example of the API’s JSON response object, and a “Get Started” button.

Click “Get Started." Once you’ve signed up or logged in, you’ll be taken to the API’s documentation page where you should see options for documentation, pricing, and support. You’ll also see your API key.
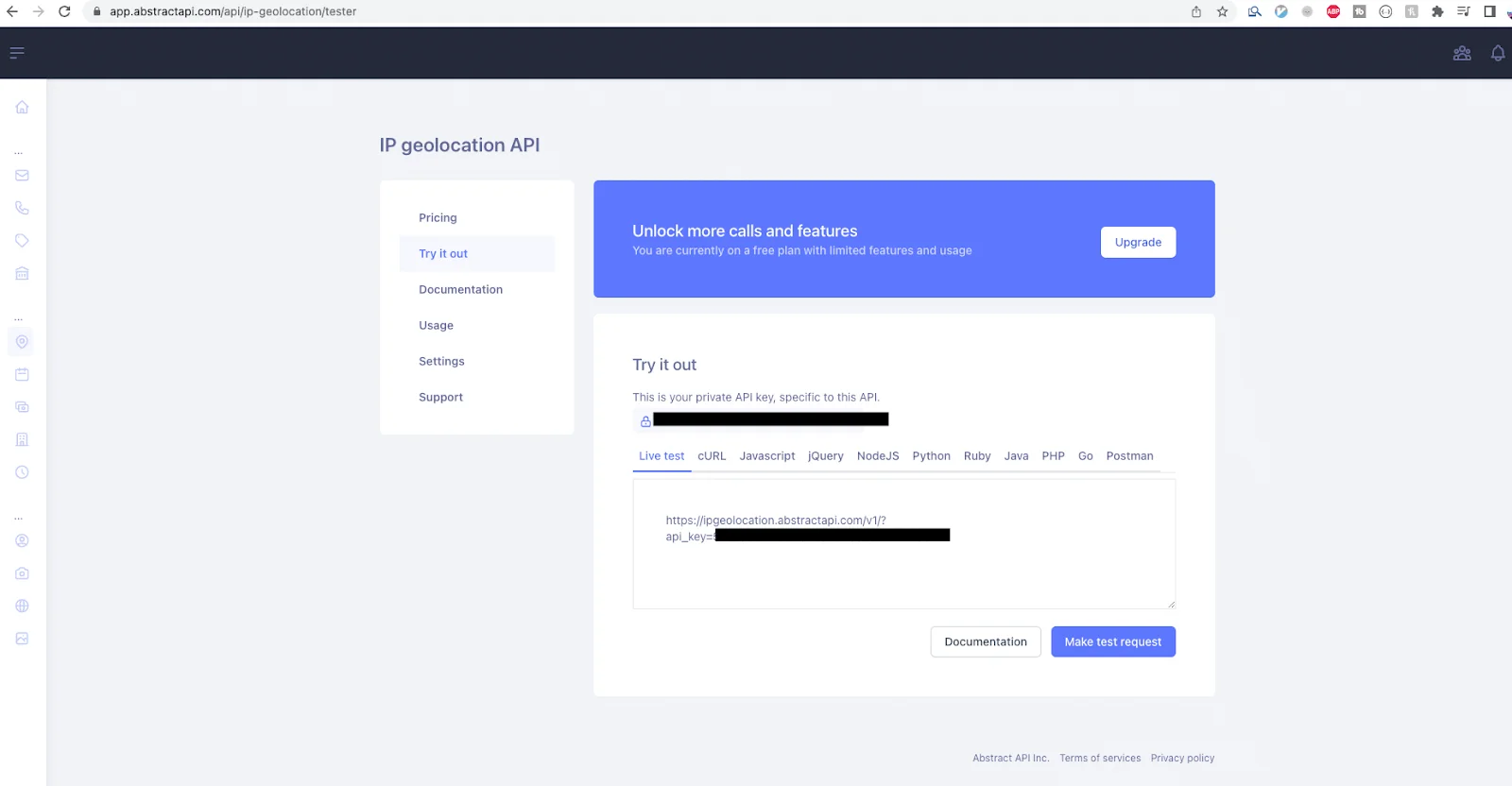
Get Zip Codes From the API
We’ll use React's built-in HTTP module, fetch, to send a GET request to the AbstractAPI endpoint. In App.jsx, write a function called getUserLocation to send the request to the API and examine the JSON result.
Let's quickly break down what's going on here.
We wrote a function called getUserLocation that uses fetch to send a request to the API. We added our unique API key to the URL as a query string to authenticate against the endpoint.
You'll notice that we're not actually sending an IP address to the endpoint: that's because if you don't include an IP address, the API automatically uses the address of the device you sent the request from.

Finally, we call our getUserLocation function from componentDidMount so that it runs as soon as the app loads. For now, we're just logging the API's response to the console so we can look at it.
When you look at the log of the response data, you should see a JSON object like this.
(Note: identifying information has been redacted to retain privacy of the author.)
The information we want is the postal code field. Many countries use the term "postal codes" instead of "zip codes." Let's pull this information out of the response object and display it to our user.
Display Zip Codes to the User
Remove the console.log statement and add the following code instead:
Next, render the zipCode variable in a <p> tag in your HTML.
Head back to localhost:3000 and refresh the page. The app will make a request to the API endpoint, which will send back a JSON response with our geographical location data. From that geographical location information, we pull the postal_code and set it in the state to be rendered.
Handle Errors
This is all well and good if our request to the API goes well, but what if something goes wrong while making the network request? In that case, we should show an error to our user to let them know something went wrong.

Create a variable in state for your error, and set an error message inside the catch block of your try/catch.
Now, add a separate <p> tag to render the error message instead of the zip codes if there is an error.
Conclusion
In this tutorial, we learned how to get area code information from an API using a user's IP address. There are many other ways to use this type of geolocation information, including rendering a user's position on a map or using position information to authenticate a user.
FAQs
What is my IP zip code?
Your IP address is a unique string of numbers that identifies your computer or mobile phone to the internet. Some people think an IP address is a zip code, but that is not the answer. An IP address is not the same as a zip code, but you can get information about your area code, city, country, region, etc. by analyzing your IP address.
What is the geolocation of an IP address?
IP geolocation is the process of mapping IP addresses to their geographic location in the real world. Every device that is connected to the internet has an IP address. The mapping of an IP address to its real-world location can be done with easily the help of an IP geolocation lookup tool, such as an API, library, or online website.
Can you find location from an IP address?
You can find some location information from an IP address. An IP address will tell you zip code, country, city, and state information. It will not reveal your precise location like a home address does. You cannot get someone's name, phone number, or other identifying information from an IP address.